C++ String Exercises: Check first string contains letters from the second
33. Check if Letters of One String Appear in Another
A string is created using the letters of another string. Letters are case sensitive. Write a C++ program to verify that the letters in the second string appear in the first string. Return true otherwise false.
A string is created by using the letters of another string. Letters are case sensitive.
Test Data:
("CPP", "Cpp") -> false
("Java", "Ja") -> true
("Check first string", "sifC") ->true
Sample Solution:
C++ Code:
#include <bits/stdc++.h> // Includes all standard libraries
using namespace std; // Using the standard namespace
// Function to check if str1 contains all letters from str2, regardless of case
bool test(string str1, string str2) {
int ctr = 0; // Counter to track matches between str1 and str2 characters
// Loop through each character in str2
for (int i = 0; i < str2.size(); i++) {
// Loop through each character in str1
for (int j = 0; j < str1.size(); j++) {
// If the characters match (case-sensitive), increment the counter and break
if (str2[i] == str1[j]) {
ctr++;
break;
}
}
}
// Return true if the counter equals the size of str2 and str1 is equal or longer than str2
return str2.size() == ctr && str1.size() >= str2.size();
}
int main() {
// Define test strings str1 and str2, then perform tests
string str1 = "CPP"; // Test string 1
string str2 = "Cpp"; // Test string 2
cout << "String1: " << str1; // Display string1
cout << "\nString2: " << str2; // Display string2
cout << "\nCheck first string contains letters from the second:\n";
cout << test(str1, str2) << endl; // Call the test function and display the result
// Repeat the same process for different strings
str1 = "Java";
str2 = "Ja";
cout << "\nString1: " << str1;
cout << "\nString2: " << str2;
cout << "\nCheck first string contains letters from the second:\n";
cout << test(str1, str2) << endl;
str1 = "Check first string";
str2 = "sifC";
cout << "\nString1: " << str1;
cout << "\nString2: " << str2;
cout << "\nCheck first string contains letters from the second:\n";
cout << test(str1, str2) << endl;
}
Sample Output:
String1: CPP String2: Cpp Check first string contains letters from the second: 0 String1: Java String2: Ja Check first string contains letters from the second: 1 String1: Check first string String2: sifC Check first string contains letters from the second: 1
Flowchart:
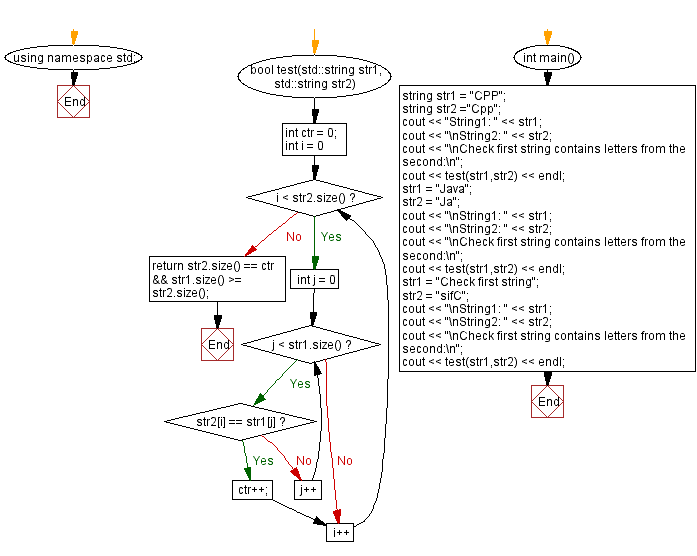
For more Practice: Solve these Related Problems:
- Write a C++ program to determine if all characters in a second string appear in a first string, considering case sensitivity.
- Write a C++ program that reads two strings and checks if the letters of the second form a subsequence of the first.
- Write a C++ program to verify that each character in one string is contained in another string regardless of order.
- Write a C++ program to compare two strings and return true if every character in the second string exists in the first.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Reverse the words of three or more lengths in a string.
Next C++ Exercise: Remove a word from a given string.What is the difficulty level of this exercise?