C++ String Exercises: Check whether a string is uppercase or lowercase
31. Check if a String Contains Only Uppercase or Lowercase Letters
Write a C++ program to check if a given string contains only uppercase or only lowercase letters. Return "True" if the string is uppercase or lowercase, otherwise "False".
Sample Data:
("ABCDEF") -> “True”
("abcdef") -> “True”
("ABcDef") -> “False”
Sample Solution-1:
C++ Code:
#include <bits/stdc++.h> // Include all standard libraries
using namespace std; // Using the standard namespace
// Function to check if the string is all uppercase or all lowercase
string test(string text) {
for (int i = 1; i < text.size(); i++) { // Loop through the string starting from the second character
// Check if the case of the first character is different from the current character
if (isupper(text[0]) != isupper(text[i])) { // Check if the first character's case differs from the current character
return "False"; // If cases differ, return "False" indicating it's not all uppercase or all lowercase
}
}
return "True"; // If all characters have the same case, return "True" indicating it's all uppercase or all lowercase
}
int main() {
string text = "ABCDEF"; // Test string containing all uppercase characters
//string text = "abcdef"; // Test string containing all lowercase characters
//string text = "ABcDef"; // Test string containing a mix of uppercase and lowercase characters
cout << "Original string: " << text; // Display the original string
cout << "\n\nCheck whether the said string is uppercase or lowercase: ";
cout << test(text) << endl; // Call the function to check and display whether the string is all uppercase or all lowercase
}
Sample Output:
Original string: ABCDEF Check whether the said string is uppercase or lowercase: True
Flowchart:
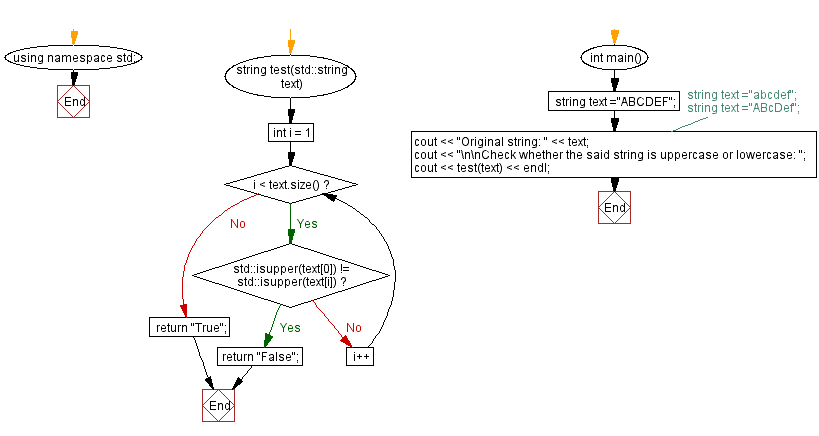
Sample Solution-2:
C++ Code:
#include <bits/stdc++.h> // Include all standard libraries
using namespace std; // Using the standard namespace
// Function to check if the string is all uppercase or all lowercase
string test(string text) {
// Check if the first character of the string is uppercase
if (isupper(text[0])) { // If the first character is uppercase
// Loop through each character in the string
for (auto ch : text) {
// If any character is not uppercase, return "False"
if (!isupper(ch))
return "False";
}
}
// Check if the first character of the string is lowercase
if (islower(text[0])) { // If the first character is lowercase
// Loop through each character in the string
for (auto ch : text) {
// If any character is not lowercase, return "False"
if (!islower(ch))
return "False";
}
}
// If all characters in the string match the case of the first character, return "True"
return "True";
}
int main() {
//string text ="ABCDEF"; // Test string containing all uppercase characters
//string text ="abcdef"; // Test string containing all lowercase characters
string text ="ABcDef"; // Test string containing a mix of uppercase and lowercase characters
cout << "Original string: " << text; // Display the original string
cout << "\n\nCheck whether the said string is uppercase or lowercase: ";
cout << test(text) << endl; // Call the function to check and display whether the string is all uppercase or all lowercase
}
Sample Output:
Original string: ABcDef Check whether the said string is uppercase or lowercase: False
Flowchart:
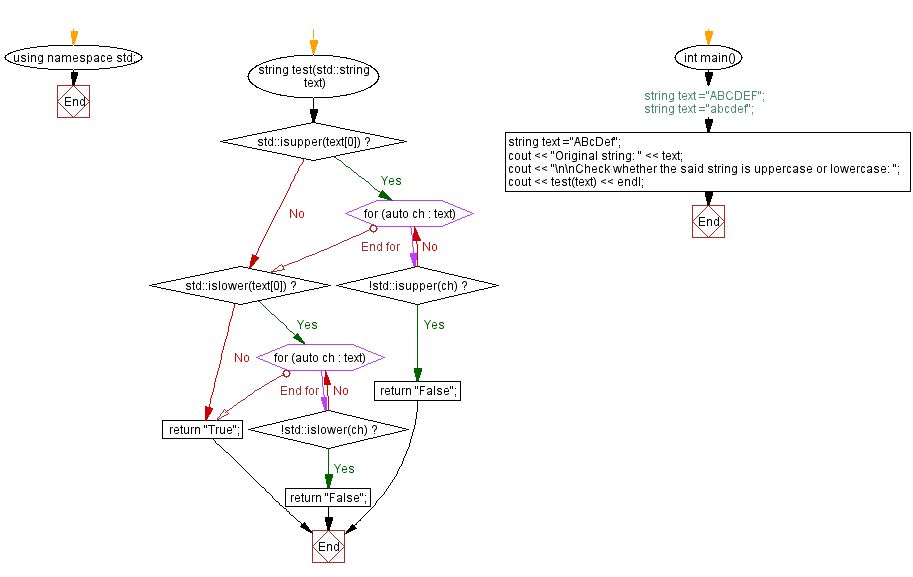
For more Practice: Solve these Related Problems:
- Write a C++ program to verify if all characters in a string are uppercase and return true if so.
- Write a C++ program that reads a string and checks whether it contains exclusively lowercase letters.
- Write a C++ program to determine if a string is either entirely uppercase or entirely lowercase, and print a boolean result.
- Write a C++ program that processes a string and outputs "True" if the string is uniform in case, otherwise "False".
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Identify the missing letter in a string.
Next C++ Exercise: Reverse the words of three or more lengths in a string.What is the difficulty level of this exercise?