C++ String Exercises: Identify the missing letter in a string
Write a C++ program to identify the missing letter in a given string (list of alphabets). The method returns, "There is no missing letter!" if no letters are missing from the string.
Sample Data:
("abcef") -> "d"
("abcdef") -> "There is no missing letter!"
Sample Solution:
C++ Code:
#include <bits/stdc++.h> // Include all standard libraries
using namespace std; // Using the standard namespace
// Function to identify a missing letter in a string sequence
string test(string text) {
int length = text.size(); // Get the length of the input string
for (int i = 1; i < length; i++) { // Iterate through the string
if (++text[i - 1] != text[i]) { // Check if the adjacent characters are consecutive
return text.substr(i - 1, 1); // If a missing letter is found, return the missing letter
}
}
return "\nThere is no missing letter!"; // If no missing letter is found, return a message indicating so
}
int main() {
//string text ="abcef";
string text = "abcdef";
cout << "Original string: " << text; // Display the original string
cout << "\n\nIdentify the missing letter in the said string: ";
cout << test(text) << endl; // Call the function to identify the missing letter and display the result
}
Sample Output:
Original string: abcdef Identify the missing letter in the said string: There is no missing letter!
Flowchart:
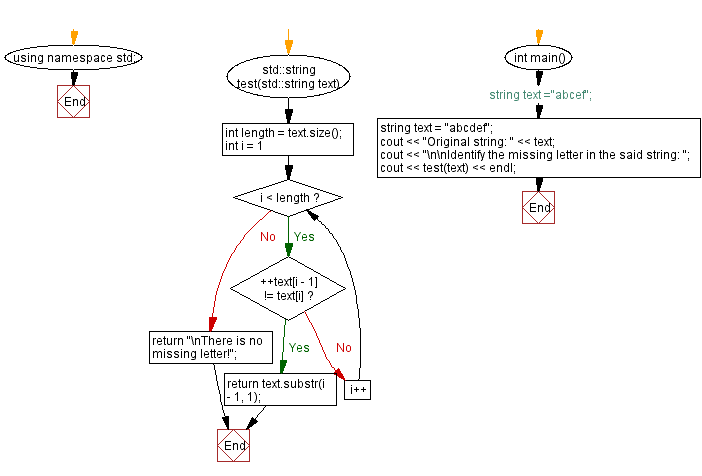
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Print an integer with commas as thousands separators.
Next C++ Exercise: Check whether a string is uppercase or lowercase.What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics