C++ String Exercises: Capitalize the first letter of each word of a string
Write a C++ program to capitalize the first letter of each word in a given string. Words must be separated by only one space.
Visual Presentation:
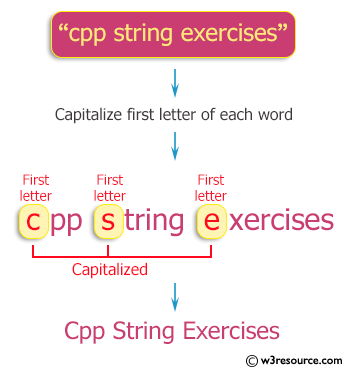
Sample Solution:
C++ Code :
#include <iostream> // Including input/output stream library
#include <string> // Including string library for string manipulation
using namespace std; // Using the standard namespace
// Function to capitalize the first letter of each word in a string
string Capitalize_first_letter(string text) {
// Loop through each character in the string
for (int x = 0; x < text.length(); x++)
{
// If it's the first character of the string or after a space, capitalize it
if (x == 0 || text[x - 1] == ' ')
{
text[x] = toupper(text[x]); // Convert the character to uppercase
}
}
return text; // Return the modified string
}
int main()
{
// Displaying the string with the first letter of each word capitalized
cout << Capitalize_first_letter("Write a C++ program");
// Displaying another string with the first letter of each word capitalized
cout << "\n" << Capitalize_first_letter("cpp string exercises");
return 0; // Returning 0 to indicate successful execution
}
Sample Output:
Write A C++ Program Cpp String Exercises
Flowchart:
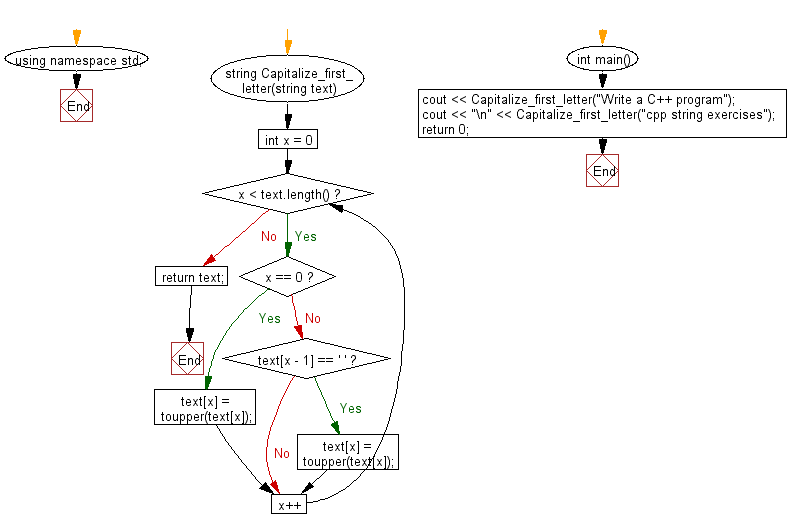
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Change every letter in a string with the next one.
Next C++ Exercise: Find the largest word in a given string.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics