C++ String Exercises: Print an integer with commas as thousands separators
Write a C++ program to print a given integer with commas separating the thousands.
Sample Data:
(1000) -> "1,000"
(10000) -> "10,000"
(23423432) -> " 23,423,432"
Sample Solution-1:
C++ Code:
#include <bits/stdc++.h> // Include all standard libraries
using namespace std; // Using the standard namespace
// Function to format an integer with commas as thousands separators
string test(int n) {
string result = to_string(n); // Convert integer 'n' to a string and store it in 'result'
// Loop to insert commas for thousands separators in the string
for (int i = result.size() - 3; i > 0; i -= 3) {
result.insert(i, ","); // Insert a comma after every three digits from the end
}
return result; // Return the formatted string
}
int main() {
int n; // Declare an integer variable to store user input
cout << "Input a number: ";
cin >> n; // Input an integer from the user
cout << "\nPrint the said integer with commas as thousands separators:\n";
cout << test(n) << endl; // Output the formatted integer with commas
}
Sample Output:
Input a number: Print the said integer with commas as thousands separators: 5,000
Flowchart:
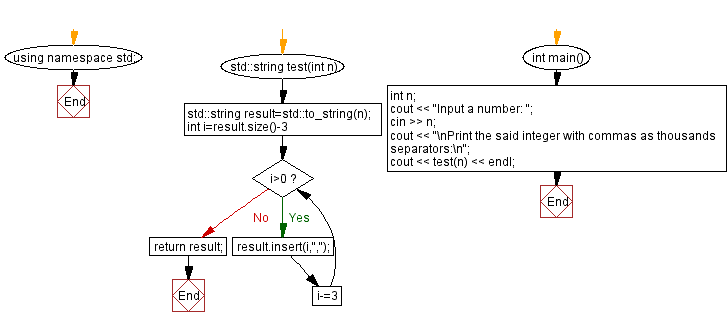
Sample Solution-2:
C++ Code:
#include <bits/stdc++.h> // Include all standard libraries
using namespace std; // Using the standard namespace
// Function to format an integer with commas as thousands separators
string test(int n) {
string result_str = to_string(n); // Convert the integer 'n' to a string and store it in 'result_str'
// Check if the length of the string is greater than 3 (to insert commas for thousands separators)
if (result_str.length() > 3) {
// Loop to insert commas for thousands separators in the string
for (int i = result_str.length() - 3; i > 0; i -= 3) {
result_str.insert(i, ","); // Insert a comma after every three digits from the end
}
}
return result_str; // Return the formatted string
}
int main() {
int n; // Declare an integer variable to store user input
cout << "Input a number: ";
cin >> n; // Input an integer from the user
cout << "\nPrint the said integer with commas as thousands separators:\n";
cout << test(n) << endl; // Output the formatted integer with commas
}
Sample Output:
Input a number: Print the said integer with commas as thousands separators: 5,000
Flowchart:
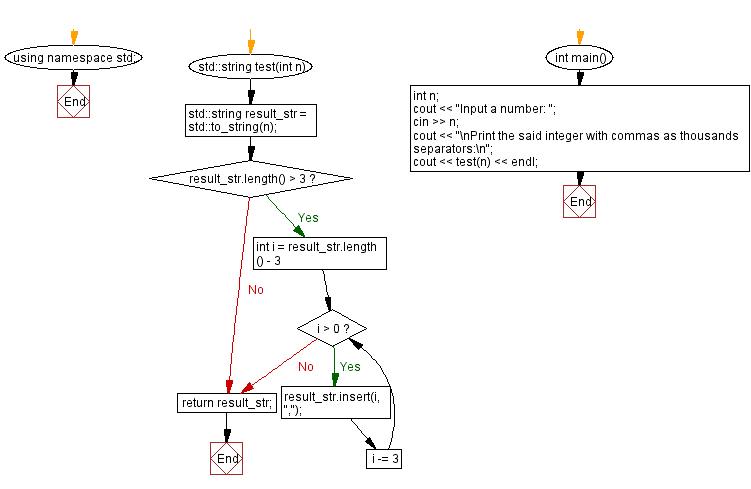
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Extract the first n number of vowels from a string.
Next C++ Exercise: Identify the missing letter in a string.What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics