C++ String Exercises: Insert white spaces between lower and uppercase Letters
27. Insert Space Before Lowercase Following Uppercase
Write a C++ program to insert a space when a lower character follows an upper character in a given string.
Sample Data:
(“TheQuickBrownFox”) -> "The Quick Brown Fox."
(“redgreenwhite”) -> "redgreenwhite"
Sample Solution-1:
C++ Code:
#include <bits/stdc++.h> // Includes all standard libraries
using namespace std; // Using the standard namespace
// Function to insert white spaces between lower and uppercase letters in the input string
string test(string text) {
string new_str = ""; // Initialize an empty string to store the modified string
// Iterate through the characters in the string (except the last character)
for(int i = 0; i < (text.length() - 1); i++) {
new_str += text[i]; // Append the current character to the new string
// Check if the next character is uppercase
if(isupper(text[i + 1])) {
new_str += " "; // If the next character is uppercase, insert a space in the new string
}
}
new_str += text.back(); // Append the last character of the input string to the new string
return new_str; // Return the modified string
}
int main() {
string text = "TheQuickBrownFox."; // Define an input string
//string text = "C++String exercises";
//string text = "redgreenwhite";
cout << "Original String:\n";
cout << text; // Output the original string
cout << "\n\nInsert white spaces between lower and uppercase Letters in the said string:\n";
cout << test(text) << endl; // Output the modified string with spaces inserted
}
Sample Output:
Original String: TheQuickBrownFox. Insert white spaces between lower and uppercase Letters in the said string: The Quick Brown Fox.
Flowchart:
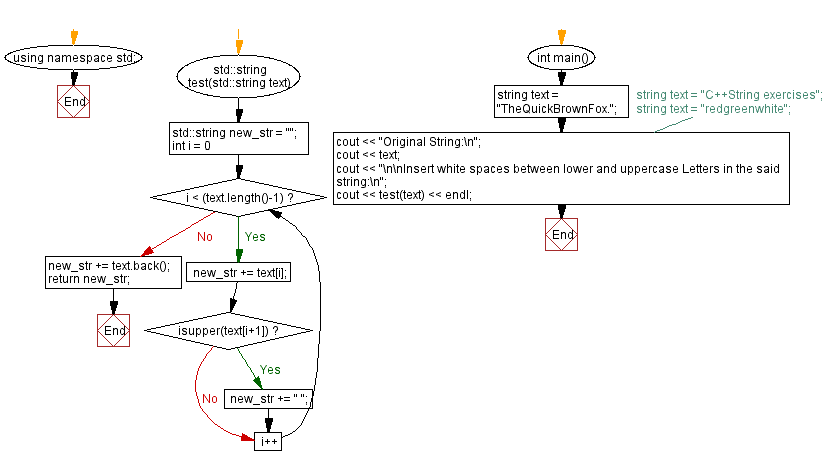
Sample Solution-2:
C++ Code:
#include <bits/stdc++.h> // Includes all standard libraries
using namespace std; // Using the standard namespace
// Function to insert white spaces between lowercase and uppercase letters in the input string
string test(string text) {
int n = 0; // Initialize a counter variable
// Loop through the string to find lowercase followed by uppercase letters
while (n + 1 < text.length()) {
// Check if the current character is lowercase and the next character is uppercase
if (islower(text[n]) && isupper(text[n + 1])) {
// Insert a space between the lowercase and uppercase letters in the string
text = text.substr(0, n + 1) + " " + text.substr(n + 1);
n++; // Increment the counter to skip the inserted space
}
n++; // Move to the next character in the string
}
return text; // Return the modified string
}
int main() {
string text = "TheQuickBrownFox."; // Define an input string
//string text = "C++String exercises";
//string text = "redgreenwhite";
cout << "Original String:\n";
cout << text; // Output the original string
cout << "\n\nInsert white spaces between lower and uppercase Letters in the said string:\n";
cout << test(text) << endl; // Output the modified string with spaces inserted
}
Sample Output:
Original String: TheQuickBrownFox. Insert white spaces between lower and uppercase Letters in the said string: The Quick Brown Fox.
Flowchart:
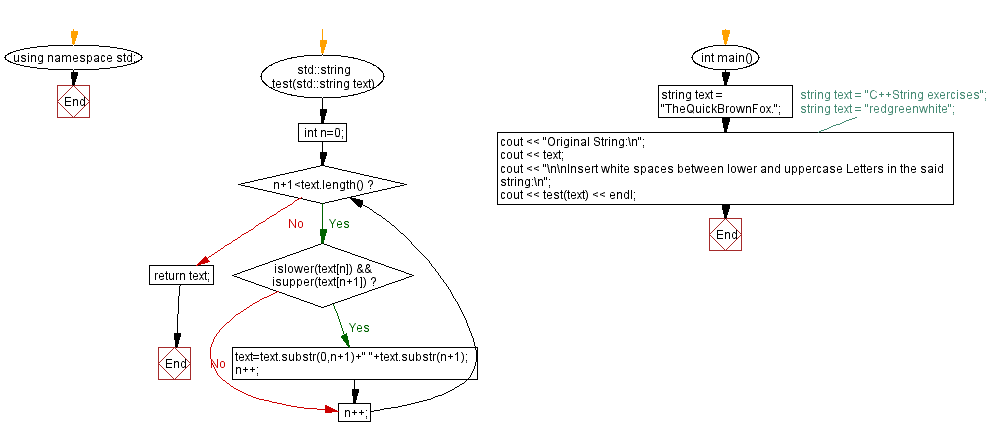
For more Practice: Solve these Related Problems:
- Write a C++ program to insert a space before any lowercase letter that immediately follows an uppercase letter in a string.
- Write a C++ program that processes a camelCase string and outputs it with spaces inserted before each new word.
- Write a C++ program to scan a string and insert whitespace between any transition from an uppercase to a lowercase character.
- Write a C++ program that reads a concatenated string with mixed case and prints it with appropriate spaces inserted.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Check a string is a title cased string or not.
Next C++ Exercise: Extract the first n number of vowels from a string.What is the difficulty level of this exercise?