C++ String Exercises: Longest consecutive ones in a binary string
25. Longest Sequence of Consecutive Ones in a Binary String
Write a C++ program to find the longest sequence of consecutive ones in a given binary string.
Sample Data:
("1100110001") -> “11”
(“00100111011”) -> “111”
(“00000”) -> “”
Sample Solution-1:
C++ Code:
#include <bits/stdc++.h> // Includes all standard libraries
using namespace std; // Using the standard namespace
std::string test(std::string text) { // Function to find the longest sequence of consecutive ones in a binary string
int ctr = 0; // Initialize a counter for consecutive ones
int result = 0; // Initialize a variable to hold the maximum count of consecutive ones
for (int i = 0; i < text.size(); i++) { // Loop through the characters of the input string
if (text[i] == '0') // If the current character is '0', reset the counter
ctr = 0;
else { // If the current character is '1'
ctr++; // Increment the consecutive ones counter
result = std::max(result, ctr); // Update the maximum count of consecutive ones found so far
}
}
std::string result1(result, '1'); // Create a string of '1's with length equal to the maximum count of consecutive ones
return result1; // Return the string of consecutive ones
}
int main() {
string text = "1100110001"; // Declare and initialize a binary string
//string text = "00100111011";
//string text = "00000";
cout << "Original Binary String:\n";
cout << text; // Output the original binary string
cout << "\n\nLongest sequence of consecutive ones of the said binary string:\n";
cout << test(text) << endl; // Output the longest sequence of consecutive ones in the string
}
Sample Output:
Original Binary String: 1100110001 Longest sequence of consecutive ones of the said binary string: 11
Flowchart:
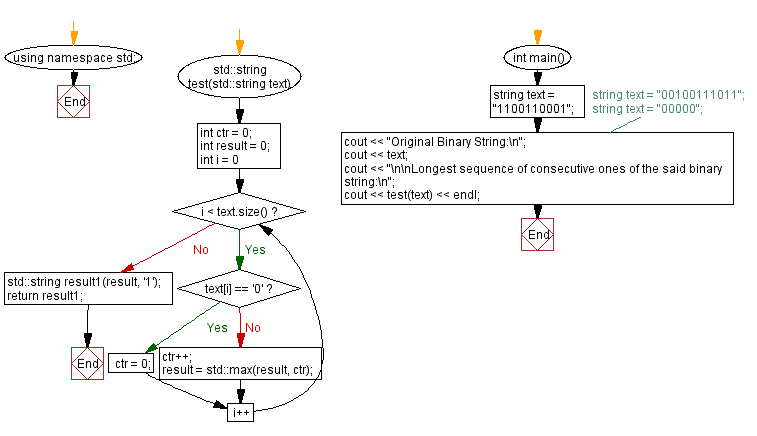
Sample Solution-2:
C++ Code:
#include <bits/stdc++.h> // Includes all standard libraries
using namespace std; // Using the standard namespace
std::string test(std::string text) { // Function to find the longest sequence of consecutive ones in a binary string
int i = 0; // Initialize a counter variable
// Loop to find the longest sequence of consecutive ones
while (text.find(string(i, '1')) != string::npos) // Find the substring of i '1's in the input text
i++; // Increment the counter to find the longest sequence of consecutive ones
return string(i - 1, '1'); // Return a string with (i - 1) consecutive '1's as the longest sequence
}
int main() {
string text = "1100110001"; // Declare and initialize a binary string
//string text = "00100111011";
//string text = "00000";
cout << "Original Binary String:\n";
cout << text; // Output the original binary string
cout << "\n\nLongest sequence of consecutive ones of the said binary string:\n";
cout << test(text) << endl; // Output the longest sequence of consecutive ones in the string
}
Sample Output:
Original Binary String: 1100110001 Longest sequence of consecutive ones of the said binary string: 11
Flowchart:
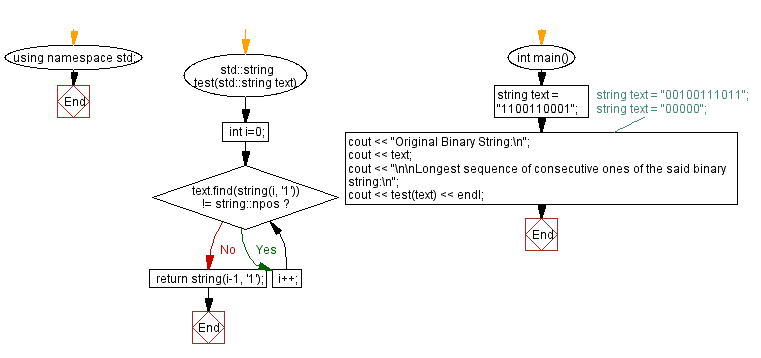
For more Practice: Solve these Related Problems:
- Write a C++ program to find the maximum length of a contiguous sequence of '1's in a binary string using a loop.
- Write a C++ program that scans a binary string and outputs the length of the longest run of consecutive ones.
- Write a C++ program to determine the longest sequence of consecutive '1' characters in a string and print the sequence itself.
- Write a C++ program that iterates through a binary string to find and return the longest streak of '1's using counters.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Count number of duplicate characters in a given string.
Next C++ Exercise: Check a string is a title cased string or not.What is the difficulty level of this exercise?