C++ String Exercises: Count number of duplicate characters in a given string
C++ String: Exercise-24 with Solution
Write a C++ program to count the number of duplicate characters in a given string.
Note: Spaces are included and characters are case sensitive
Sample Data:
(“APple”) -> 0
(“Remove all special characters from a given string”) -> 31
(“Total number of unique characters of the said two strings.”) ->36
Sample Solution-1:
C++ Code:
#include <bits/stdc++.h> // Includes all standard libraries
using namespace std; // Using the standard namespace
int test(std::string text) { // Function to count duplicate characters in a string
int ctr = 0; // Counter to keep track of duplicate characters
sort(text.begin(), text.end()); // Sorts characters in the string alphabetically
// Iterate through each character in the string
for (int i = 0; i < text.size(); i++) {
if (text[i] == text[i + 1]) { // If the current character is the same as the next one
ctr = ctr + 1; // Increment the counter for duplicate characters
}
}
return ctr; // Return the count of duplicate characters
}
int main() {
//string text = "APple";
//string text = "Remove all special characters from a given string.";
string text = "Total number of unique characters of the said two strings."; // Declare and initialize a string
cout << "Original String:\n";
cout << text; // Output the original string
cout << "\n\nNumber of duplicate characters in the said string: ";
cout << test(text) << endl; // Call function test and output the number of duplicate characters in the string
}
Sample Output:
Original String: Total number of unique characters of the said two strings. Number of duplicate characters in the said string: 36
Flowchart:
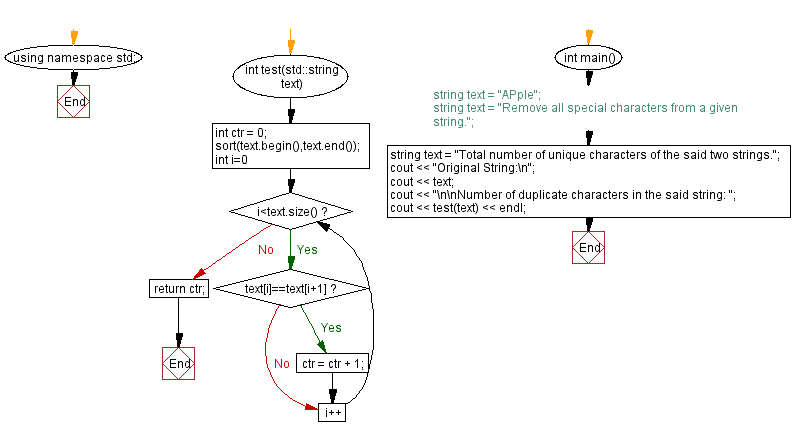
Sample Solution-2:
C++ Code:
#include <bits/stdc++.h> // Includes all standard libraries
using namespace std; // Using the standard namespace
int test(std::string text) { // Function to count duplicate characters in a string
// Creating a set from the characters in the input string, which automatically filters out duplicates
std::set<char> set(text.begin(), text.end());
int ctr = text.length() - set.size(); // Calculating the difference to get the count of duplicates
return ctr; // Return the count of duplicate characters
}
int main() {
string text = "APple"; // Declare and initialize a string
//string text = "Remove all special characters from a given string.";
//string text = "Total number of unique characters of the said two strings.";
cout << "Original String:\n";
cout << text; // Output the original string
cout << "\n\nNumber of duplicate characters in the said string: ";
cout << test(text) << endl; // Call function test and output the number of duplicate characters in the string
}
Sample Output:
Original String: APple Number of duplicate characters in the said string: 0
Flowchart:
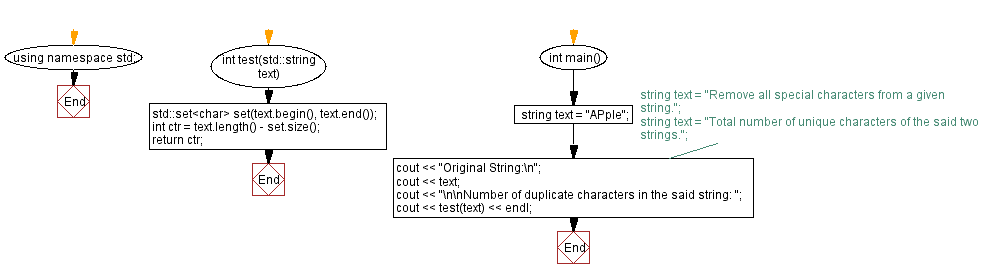
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Count the number of unique characters of two strings.
Next C++ Exercise: Longest consecutive ones in a binary string.What is the difficulty level of this exercise?
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/cpp-exercises/string/cpp-string-exercise-24.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics