C++ String Exercises: Count the number of unique characters of two strings
23. Count Unique Characters in Two Strings
Write a C++ program that counts the number of unique characters in two given strings.
Sample Data:
("Apple", "red") -> 6
[ Unique characters: A, p, l, e, r, d ]
("Python", "Java") -> 9
[ Unique characters: P, y, t, h, o, n, J, a v ]
Sample Solution-1:
C++ Code:
#include <bits/stdc++.h> // Includes all standard libraries
using namespace std; // Using the standard namespace
int test(std::string text1, std::string text2) {
std::string result; // Declare an empty string to store unique characters
// Loop through each character in the concatenation of text1 and text2
for(char &ch : text1 + text2) {
// Check if the character is not already present in the result string
if (count(result.begin(), result.end(), ch) == 0)
result += ch; // If the character is not in result, add it
}
return result.length(); // Return the length of the resulting string (number of unique characters)
}
int main() {
// Declare and initialize two strings
string text1 = "Python";
string text2 = "Java";
cout << "Original Strings:\n";
cout << "String1: " << text1; // Output the first string
cout << "\nString2: " << text2; // Output the second string
cout << "\nTotal number of unique characters of the said two strings: ";
cout << test(text1, text2) << endl; // Call function test with two strings and output the number of unique characters
}
Sample Output:
Original Strings: String1: Python String2: Java Total number of unique characters of the said two strings: 9
Flowchart:
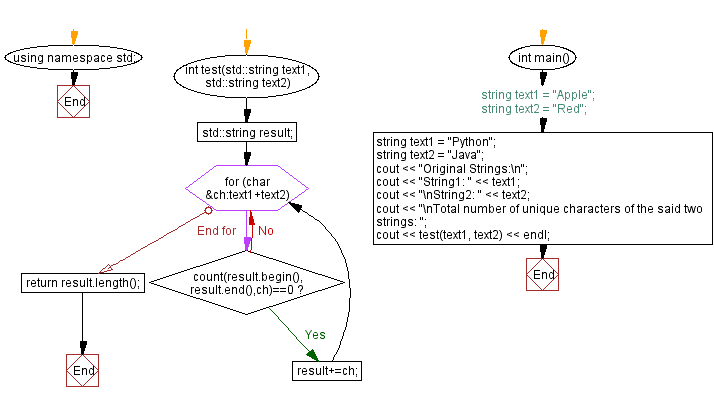
Sample Solution-2:
C++ Code:
#include <bits/stdc++.h> // Includes all standard libraries
using namespace std; // Using the standard namespace
int test(std::string text1, std::string text2) {
std::set<char> result; // Define a set to store unique characters
std::string text = text1 + text2; // Concatenate text1 and text2 into a single string
// Iterate through each character in the concatenated string
for (char ch : text) {
result.insert(ch); // Insert each character into the set (sets only store unique elements)
}
return result.size(); // Return the size of the set, which represents the count of unique characters
}
int main() {
// Declare and initialize two strings
string text1 = "Python";
string text2 = "Java";
cout << "Original Strings:\n";
cout << "String1: " << text1; // Output the first string
cout << "\nString2: " << text2; // Output the second string
cout << "\nTotal number of unique characters of the said two strings: ";
cout << test(text1, text2) << endl; // Call function test with two strings and output the number of unique characters
}
Sample Output:
Original Strings: String1: Python String2: Java Total number of unique characters of the said two strings: 9
Flowchart:
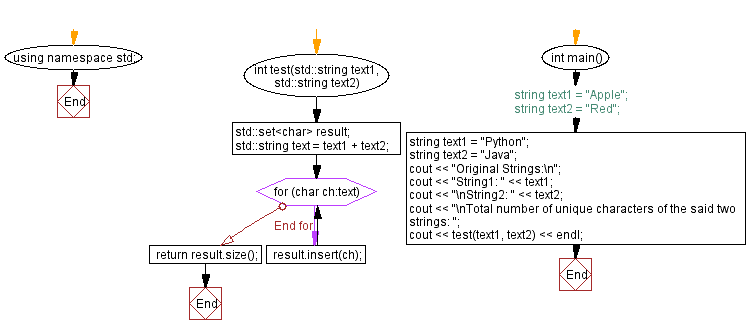
For more Practice: Solve these Related Problems:
- Write a C++ program to count the total number of distinct characters present in two given strings combined.
- Write a C++ program that reads two strings and uses an array or set to determine the count of unique letters.
- Write a C++ program to merge two strings and then count how many unique alphabetic characters appear.
- Write a C++ program that finds and prints the number of characters that occur only once across two strings.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Remove all special characters from a given string.
Next C++ Exercise: Count number of duplicate characters in a given string.What is the difficulty level of this exercise?