C++ String Exercises: Remove all special characters from a given string
Write a C++ program to remove all special characters from a given string.
List of special characters: ., !, @, #, $, %, ^, &, \, *, (, )
Sample Data:
("abcd $ js# @acde$") -> “abcd js acde”
Sample Solution-1:
C++ Code:
#include<algorithm> // Standard library that includes various algorithms
#include <iostream> // Input/output stream library
#include <vector> // Standard vector library for dynamic arrays
using namespace std; // Using the standard namespace
// Function to filter special characters from the input text
std::string test(std::string text)
{
std::string result_text; // Declare an empty string to store the filtered characters
// Loop through each character in the input text
for(int i = 0; i < text.length(); ++i)
{
// Check if the character is a letter (uppercase or lowercase), space, hyphen, underscore, or digit
if((text[i] >= 'a' && text[i] <= 'z') ||
(text[i] >= 'A' && text[i] <= 'Z') ||
(text[i] == ' ') || (text[i] == '-') ||
(text[i] == '_') ||
(text[i] >= '0' && text[i] <= '9'))
{
result_text.push_back(text[i]); // If it matches the allowed characters, add it to the result string
}
}
return result_text; // Return the string containing filtered characters
}
int main() {
string str1 ="abcd $ js# @acde$";
cout << "Original string: " << str1; // Output the original string
string result = test(str1); // Call the function to filter special characters
cout << "\nNew string after removing the special characters from the said string:\n" << result; // Output the resulting string after filtering
}
Sample Output:
Original string: abcd $ js# @acde$ New string after removing the special characters from the said string: abcd js acde
Flowchart:
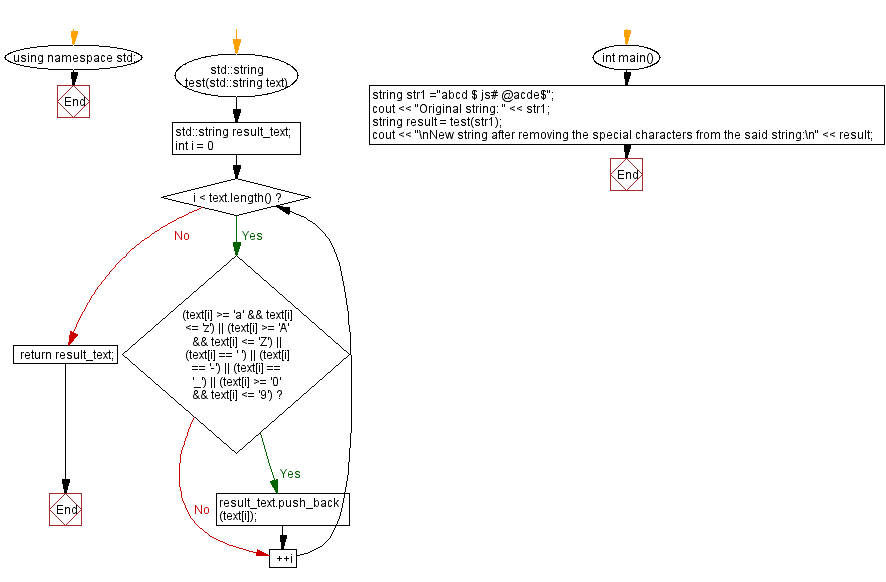
Sample Solution-2:
C++ Code:
#include<algorithm> // Standard library that includes various algorithms
#include <iostream> // Input/output stream library
#include <vector> // Standard vector library for dynamic arrays
using namespace std; // Using the standard namespace
// Function to filter special characters from the input text
std::string test(std::string text) {
std::string result_str; // Declare an empty string to store the filtered characters
// Loop through each character in the input text
for(int i = 0; i < text.size(); i++) {
// Check if the character is a digit ('0' to '9'), uppercase letter, lowercase letter, hyphen, underscore, or space
if ((text[i] >= 48 && text[i] < 59) || (std::toupper(text[i]) >= 65 && std::toupper(text[i]) < 91) || text[i] == '-' || text[i] == ' ' || text[i] == '_') {
result_str.push_back(text[i]); // If it matches the allowed characters, add it to the result string
}
}
return result_str; // Return the string containing filtered characters
}
int main() {
string str1 = "abcd $ js# @acde$";
cout << "Original string: " << str1; // Output the original string
string result = test(str1); // Call the function to filter special characters
cout << "\nNew string after removing the special characters from the said string:\n" << result; // Output the resulting string after filtering
}
Sample Output:
Original string: abcd $ js# @acde$ New string after removing the special characters from the said string: abcd js acde
Flowchart:
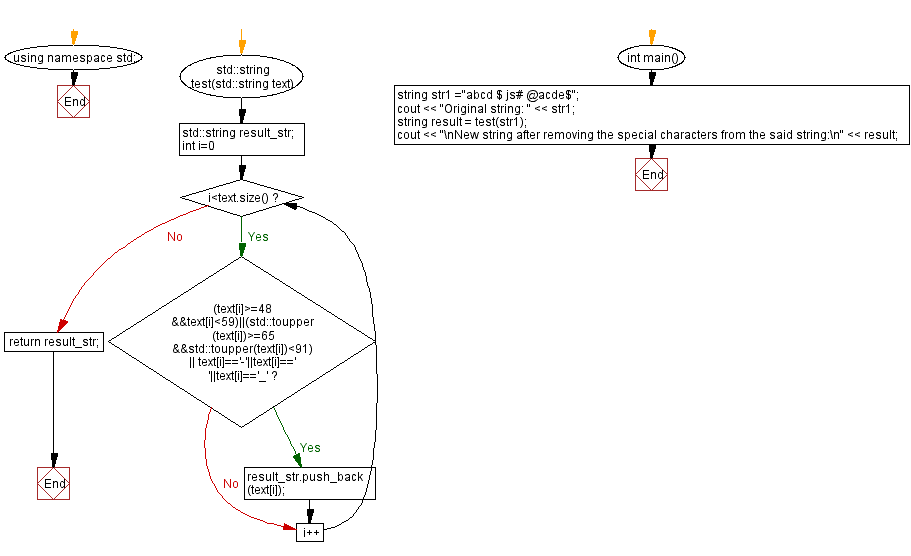
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Check if a string is a subsequence of another string.
Next C++ Exercise: Count the number of unique characters of two strings.What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics