C++ String Exercises: Change every letter in a string with the next one
C++ String: Exercise-2 with Solution
Write a C++ program to change every letter in a given string with the letter following it in the alphabet (i.e. a becomes b, p becomes q, z becomes a).
Visual Presentation:
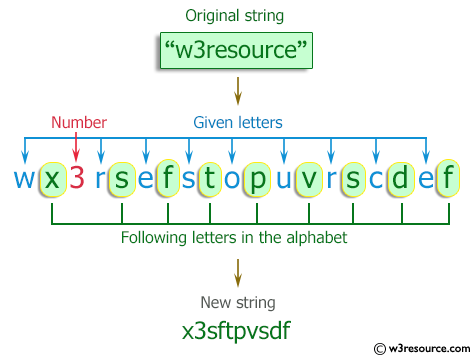
Sample Solution:
C++ Code :
#include <iostream> // Including input/output stream library
#include <string> // Including string library for string manipulation
using namespace std; // Using the standard namespace
// Function to change characters in a string to the next character in the ASCII sequence
string change_letter(string str) {
int char_code; // Variable to store ASCII value of characters
// Loop through each character in the string
for (int x = 0; x < str.length(); x++)
{
char_code = int(str[x]); // Get ASCII value of the character
// Check if the character is 'z', then change it to 'a'
if (char_code == 122)
{
str[x] = char(97);
}
// Check if the character is 'Z', then change it to 'A'
else if (char_code == 90)
{
str[x] = char(65);
}
// Check if the character is an uppercase or lowercase letter
else if (char_code >= 65 && char_code <= 90 || char_code >= 97 && char_code <= 122)
{
str[x] = char(char_code + 1); // Change to the next character in the ASCII sequence
}
}
return str; // Return the modified string
}
int main()
{
cout << "Original string: w3resource"; // Displaying the original string
cout << "\nNew string: " << change_letter("w3resource"); // Displaying the modified string
cout << "\n\nOriginal string: Python"; // Displaying the original string
cout << "\nNew string: " << change_letter("Python"); // Displaying the modified string
return 0; // Returning 0 to indicate successful execution
}
Sample Output:
Original string: w3resource New string: x3sftpvsdf Original string: Python New string: Qzuipo
Flowchart:
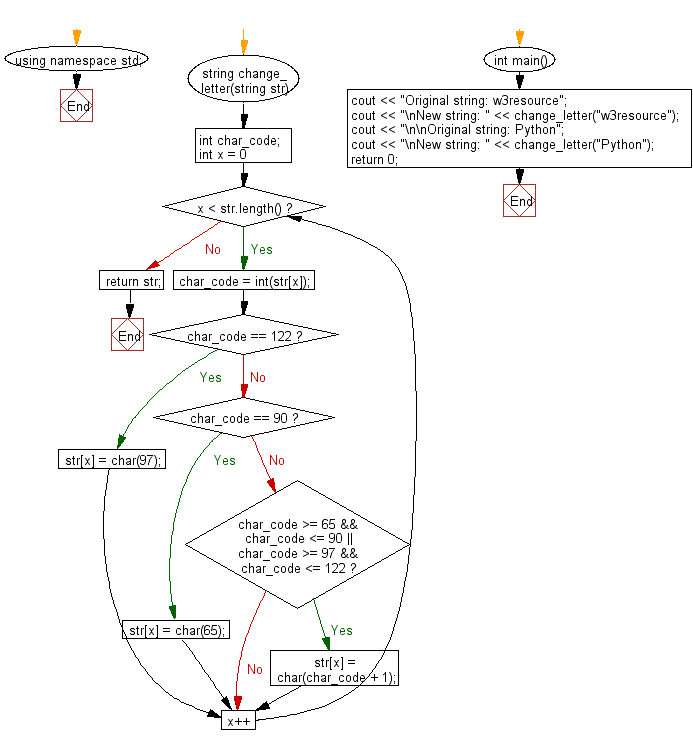
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Reverse a given string.
Next C++ Exercise: Capitalize the first letter of each word of a string.
What is the difficulty level of this exercise?
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/cpp-exercises/string/cpp-string-exercise-2.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics