C++ String Exercises: Reverse only the vowels of a given string
Write a C++ program to reverse only the vowels of a given string.
A vowel is a syllabic speech sound pronounced without any stricture in the vocal tract. Vowels are one of the two principal classes of speech sounds, the other being the consonant.
Example-1:
Input: w3resource
Output: w3resuorce
Example-2:
Input: Python
Output: Python
Example-3:
Input: Hello
Output: Holle
Example-4:
Input: USA
Output: ASU
Sample Solution:
C++ Code:
#include <iostream> // Input/output stream library
#include<algorithm> // Standard library for algorithms such as 'std::swap'
#include <stack> // Standard library for stack data structure
using namespace std; // Using the standard namespace
// Function to reverse the vowels in a given string
string reverse_vowels(string ostr) {
vector<int> vec_data; // Vector to store the indices of vowels in the string
string result_str = ostr; // Initialize result string with the original string
// Loop through the original string to find the indices of vowels and store them in vec_data
for(int i = 0; i < ostr.length(); i++) {
if(ostr[i] == 'A' or ostr[i] == 'E' or ostr[i] == 'I' or ostr[i] == 'O' or ostr[i] == 'U' or ostr[i] == 'a' or ostr[i] == 'e' or ostr[i] == 'i' or ostr[i] == 'o' or ostr[i] == 'u' ) {
vec_data.push_back(i); // Store the index of the vowel in the vector
}
}
// Reverse the vowels in the result string using the indices stored in vec_data
for(int i = 0; i < vec_data.size() / 2; i++) {
swap(result_str[vec_data[i]], result_str[vec_data[vec_data.size() - 1 - i]]); // Swap vowels in the result string
}
return result_str; // Return the string with reversed vowels
}
// Main function
int main() {
// Test cases
char str1[] = "w3resource";
cout << "Original string: " << str1;
cout << "\nAfter reversing the vowels of the said string: " << reverse_vowels(str1);
char str2[] = "Python";
cout << "\n\nOriginal string: " << str2;
cout << "\nAfter reversing the vowels of the said string: " << reverse_vowels(str2);
char str3[] = "Hello";
cout << "\n\nOriginal string: " << str3;
cout << "\nAfter reversing the vowels of the said string: " << reverse_vowels(str3);
char str4[] = "USA";
cout << "\n\nOriginal string: " << str4;
cout << "\nAfter reversing the vowels of the said string: " << reverse_vowels(str4);
return 0; // Return 0 to indicate successful completion
}
Sample Output:
Original string: w3resource After reversing the vowels of the said string: w3resuorce Original string: Python After reversing the vowels of the said string: Python Original string: Hello After reversing the vowels of the said string: Holle Original string: USA After reversing the vowels of the said string: ASU
Flowchart:
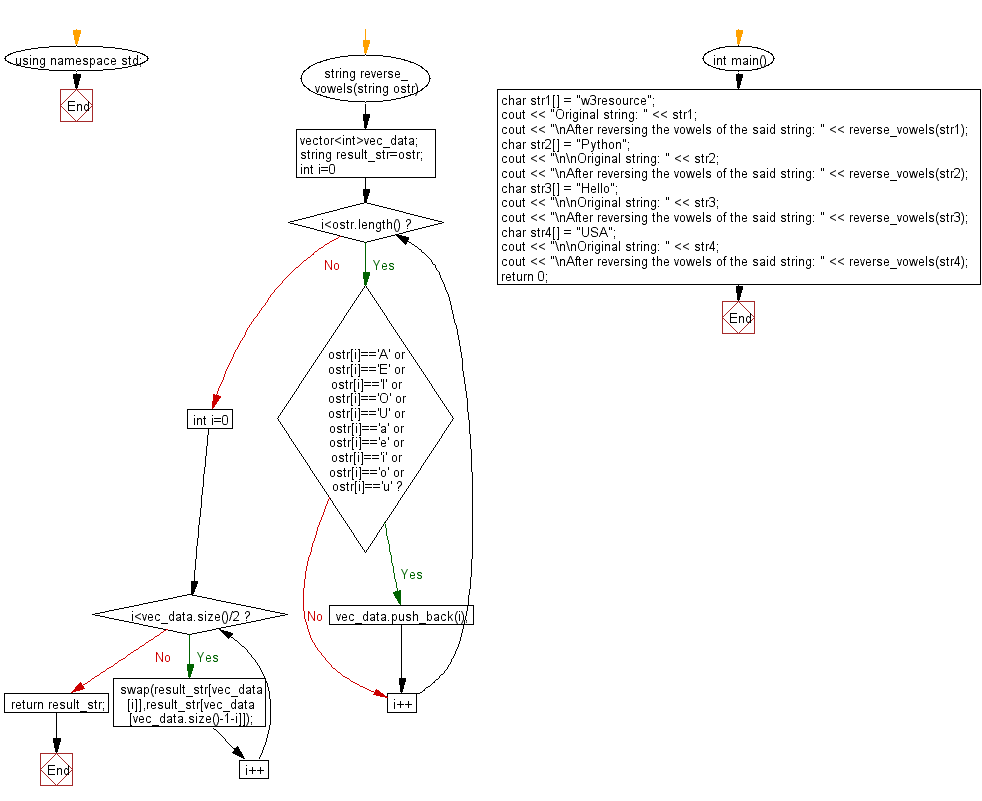
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Length of the longest valid parentheses substring.
Next C++ Exercise: Length of the longest palindrome in a given string.What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics