C++ String Exercises: Convert a given non-negative integer to English words
15. Convert a Non-Negative Integer to English Words
Write a C++ program to convert a given non-negative integer into English words.
Sample Solution:
C++ Code :
#include <stdlib.h> // Standard library for general functions in C
#include <iostream> // Input/output stream library
#include <string> // String operations library
#include <vector> // Vector container library for dynamic arrays
using namespace std; // Using the standard namespace
static string belowTwenty[] = {"Zero", "One", "Two", "Three", "Four", "Five", "Six", "Seven", "Eight", "Nine",
"Ten", "Eleven", "Twelve", "Thirteen", "Fourteen", "Fifteen", "Sixteen",
"Seventeen", "Eighteen", "Nineteen"};
static string belowHundred[] = {"", "", "Twenty", "Thirty", "Forty", "Fifty", "Sixty", "Seventy", "Eighty", "Ninety"};
static string overThousand[] = {"Hundred", "Thousand", "Million", "Billion"};
// Function to convert numbers below 100 into words
string number_to_words_below_hundred(long long int num) {
string result;
// Handling the cases for numbers below 20, between 20 and 99, and numbers greater than or equal to 100
if (num == 0) {
return result;
} else if (num < 20) {
return belowTwenty[num];
} else if (num < 100) {
result = belowHundred[num / 10];
if (num % 10 > 0) {
result += " " + belowTwenty[num % 10];
}
} else {
result = belowTwenty[num / 100] + " " + overThousand[0];
if (num % 100 > 0) {
result += " " + number_to_words_below_hundred(num % 100);
}
}
return result;
}
// Function to convert numbers into words
string number_to_words(int num) {
if (num == 0) return belowTwenty[num];
vector<string> ret;
// Loop to convert the number into words based on groups of thousands
for (; num > 0; num /= 1000) {
ret.push_back(number_to_words_below_hundred(num % 1000));
}
string result = ret[0];
// Concatenating the words and digits according to their positions
for (int i = 1; i < ret.size(); i++) {
if (ret[i].size() > 0) {
if (result.size() > 0) {
result = ret[i] + " " + overThousand[i] + " " + result;
} else {
result = ret[i] + " " + overThousand[i];
}
}
}
return result;
}
// Main function
int main() {
// Printing numbers and their word representation
long long int num = 0;
cout << num << " -> " << number_to_words(num) << endl;
num = 9;
cout << "\n" << num << " -> " << number_to_words(num) << endl;
// More test cases...
// ...
return 0; // Return 0 to indicate successful completion
}
Sample Output:
0 -> Zero 9 -> Nine 12 -> Twelve 29 -> Twenty Nine 234 -> Two Hundred Thirty Four 777 -> Seven Hundred Seventy Seven 1023 -> One Thousand Twenty Three 45321 -> Forty Five Thousand Three Hundred Twenty One 876543 -> Eight Hundred Seventy Six Thousand Five Hundred Forty Three 8734210 -> Eight Million Seven Hundred Thirty Four Thousand Two Hundred Ten 329876120 -> Three Hundred Twenty Nine Million Eight Hundred Seventy Six Thousand One Hundred Twenty 18348797629876120 -> One Billion Five Hundred Fifty Six Million Six Hundred Sixty Two Thousand One Hundred Sixty Eight
Flowchart:
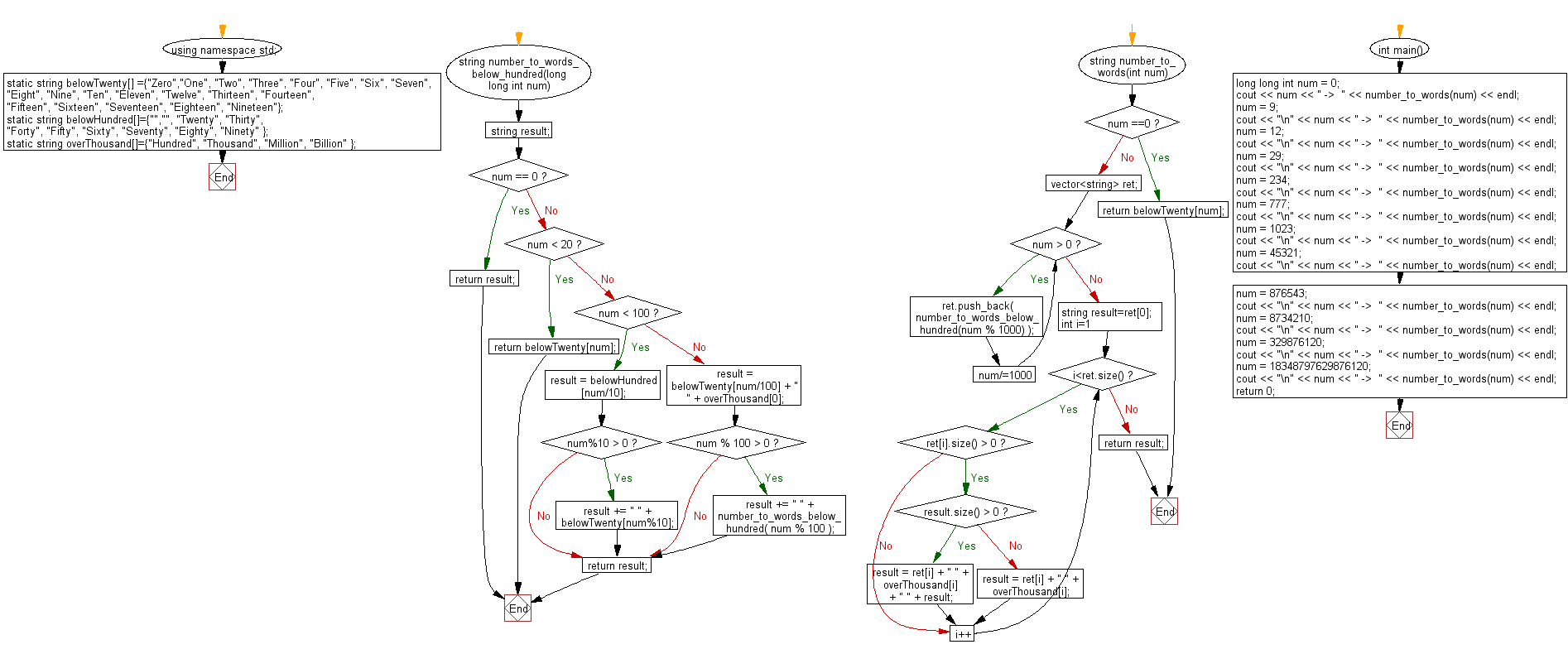
For more Practice: Solve these Related Problems:
- Write a C++ program to convert a number into its English words representation for numbers up to 99.
- Write a C++ program that converts a non-negative integer into words using arrays for digits and tens.
- Write a C++ program to read an integer and output its English equivalent by breaking the number into parts.
- Write a C++ program that handles conversion of a multi-digit number into words, including handling of tens and units properly.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Find the numbers in a string and calculate the sum.
Next C++ Exercise: Find the longest common prefix from a array of strings.What is the difficulty level of this exercise?