C++ String Exercises: Change the case of each character of a given string
13. Toggle Case of Each Character in a String
Write a C++ program to change the case (lower to upper and upper to lower cases) of each character in a given string.
Visual Presentation:
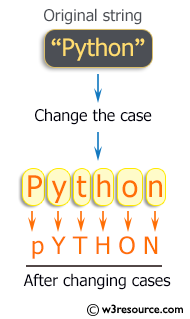
Sample Solution:
C++ Code :
#include <iostream> // Include input/output stream library
#include <string> // Include string library for string operations
#include <cctype> // Include cctype library for character handling functions
using namespace std; // Using the standard namespace
// Function to change the case of characters in a string
string change_Case(string text) {
// Loop through each character in the string
for (int x = 0; x < text.length(); x++)
{
// Check if the character is uppercase
if (isupper(text[x]))
{
text[x] = tolower(text[x]); // Convert uppercase to lowercase
}
else
{
text[x] = toupper(text[x]); // Convert lowercase to uppercase
}
}
return text; // Return the modified string with changed cases
}
// Main function
int main() {
// Output original strings and their modified versions after changing cases
cout << "Original string: Python, After changing cases-> "<< change_Case("Python") << endl;
cout << "Original string: w3resource, After changing cases-> "<< change_Case("w3resource") << endl;
cout << "Original string: AbcdEFH Bkiuer, After changing cases-> "<< change_Case(" AbcdEFH Bkiuer") << endl;
return 0; // Return 0 to indicate successful completion
}
Sample Output:
Original string: Python, After changing cases-> pYTHON Original string: w3resource, After changing cases-> W3RESOURCE Original string: AbcdEFH Bkiuer, After changing cases-> aBCDefh bKIUER
Flowchart:
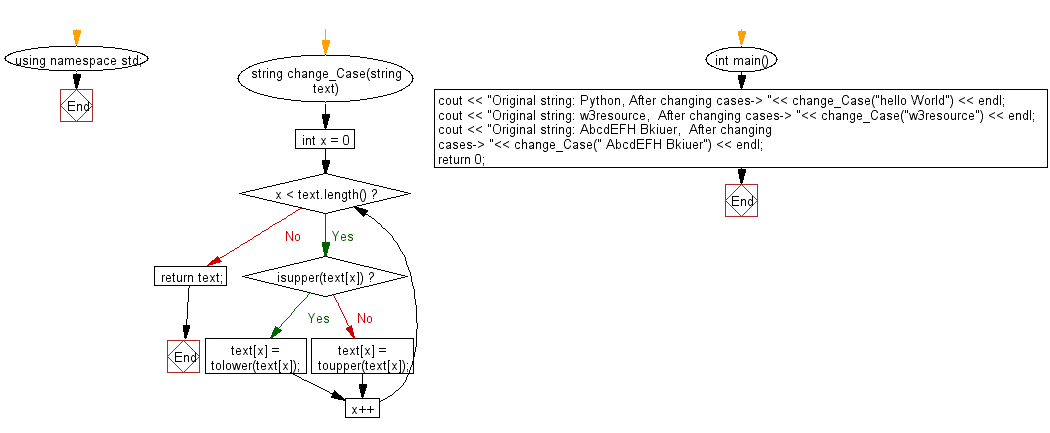
For more Practice: Solve these Related Problems:
- Write a C++ program to swap the case of every letter in a string using ASCII arithmetic.
- Write a C++ program that reads a string and converts uppercase letters to lowercase and vice versa using a loop.
- Write a C++ program to toggle the case of each character in a string without using any library functions.
- Write a C++ program that processes an input string and prints the string with each alphabet character's case reversed.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Insert a dash between two odd numbers in a string.
Next C++ Exercise: Find the numbers in a string and calculate the sum.
What is the difficulty level of this exercise?