C++ String Exercises: Insert a dash between two odd numbers in a string
Write a C++ program to insert a dash character (-) between two odd numbers in a given string of numbers.
Visual Presentation:
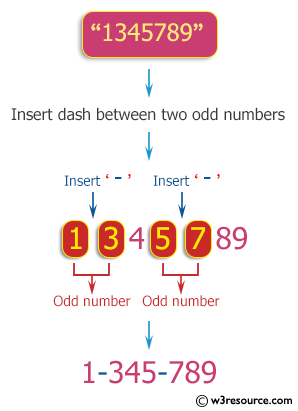
Sample Solution:
C++ Code :
#include <iostream> // Including input/output stream library
#include <string> // Including string library for string operations
using namespace std; // Using the standard namespace
// Function to insert dashes between consecutive odd digits in a string of digits
string Insert_dash(string num_str) {
string result_str = num_str; // Initializing a result string with the input number string
// Loop through the characters in the string to find consecutive odd digits
for (int x = 0; x < num_str.length() - 1; x++)
{
// Check if the current and the next characters are odd digits (1, 3, 5, 7, or 9)
if ((num_str[x] == '1' || num_str[x] == '3' || num_str[x] == '5' || num_str[x] == '7' || num_str[x] == '9') &&
(num_str[x + 1] == '1' || num_str[x + 1] == '3' || num_str[x + 1] == '5' || num_str[x + 1] == '7' || num_str[x + 1] == '9'))
{
// If consecutive odd digits are found, insert a dash between them
result_str.insert(x + 1, "-");
num_str = result_str; // Update the input string with the modified result string
}
}
return result_str; // Return the modified number string with dashes
}
// Main function
int main() {
// Output the original numbers and the numbers with dashes inserted between consecutive odd digits
cout << "Original number-123456789 : Result-> " << Insert_dash("123456789") << endl;
cout << "\nOriginal number-1345789 : Result-> " << Insert_dash("1345789") << endl;
cout << "\nOriginal number-1345789 : Result-> " << Insert_dash("34635323928477") << endl;
return 0; // Return 0 to indicate successful completion
}
Sample Output:
Original number-123456789 : Result-> 123456789 Original number-1345789 : Result-> 1-345-789 Original number-1345789 : Result-> 3463-5-323-92847-7
Flowchart:
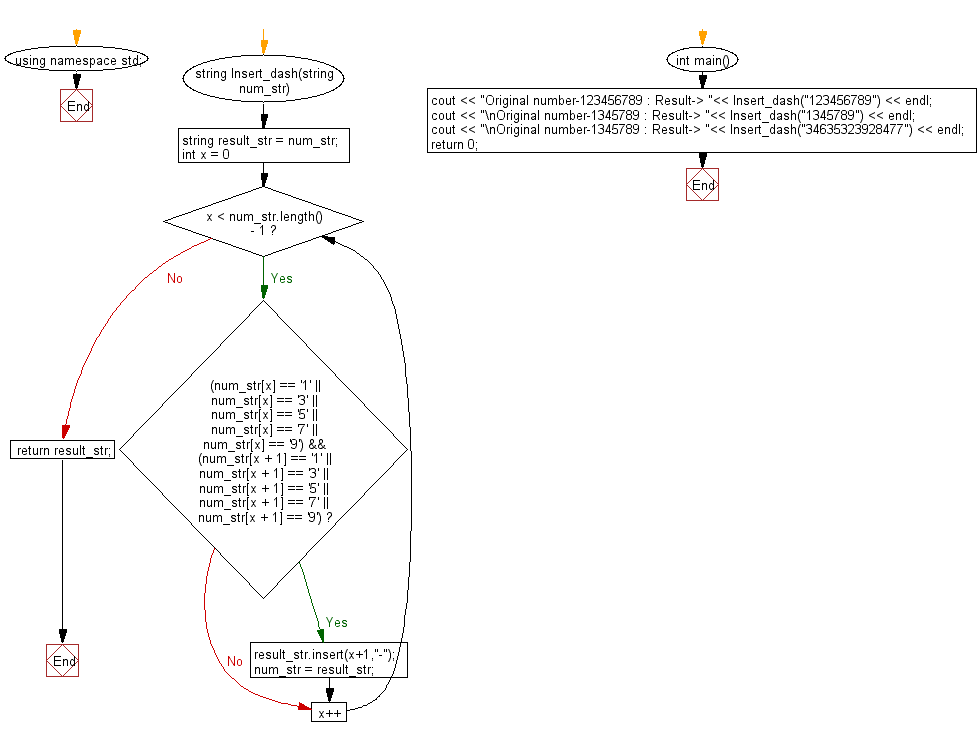
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Find a word with highest number of repeated letters.
Next C++ Exercise: Change the case of each character of a given string.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics