C++ String Exercises: Find a word with highest number of repeated letters
Write a C++ program to find a word in a given string that has the highest number of repeated letters.
Visual Presentation:
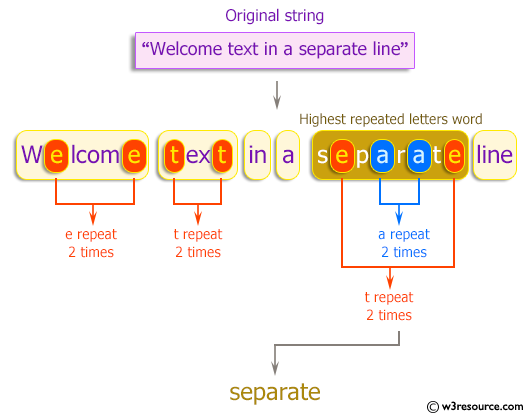
Sample Solution:
C++ Code :
#include <iostream> // Including input/output stream library
#include <string> // Including string library for string operations
using namespace std; // Using the standard namespace
// Function to find the word with the highest number of repeated letters
string highest_repeated_letters(string str) {
int str_size = str.length(); // Store the length of the input string
int ctr1 = 0, ctr2 = 0, high1 = 0, high2 = 0; // Initializing counters and variables
int start = -1, end = 0; // Initializing start and end indices
int temp1, temp2; // Temporary variables for storing indices
char letter; // Variable to store letters
for (int x = 0; x < str_size; x++, end++) // Loop through the input string
{
if (start == -1) // If start is not initialized
{
start = x; // Set the start index
}
if (str[x] == ' ' || x == str_size - 1) // If a word is encountered (space or end of string)
{
if (end == str_size - 1) // Handle case when the last word doesn't end with space
{
end += 1; // Adjust the end index
}
for (int y = start; y < end; y++) // Loop within the word boundaries
{
letter = str[y]; // Get the letter at index y
for (int z = start; z < end; z++) // Loop within the word to check for repeated letters
{
if (y == z)
{
continue; // Skip comparison with the same letter
}
else if (letter == str[z]) // If letters match, count repetition
{
ctr1++;
}
}
if (ctr1) // If there are repetitions
{
ctr2++; // Increase the counter for repeated letters
}
// Check if the current word has the highest number of repeated letters
if (ctr1 > high1 && ctr2 > high2)
{
high1 = ctr1;
high2 = ctr2;
temp1 = start;
temp2 = end;
}
ctr1 = 0; // Reset the letter counter for the next iteration
}
// Update variables for the word with the highest number of repeated letters
if (ctr2 > high2)
{
high2 = ctr2;
temp1 = start;
temp2 = end;
}
ctr2 = 0; // Reset the repeated letters counter for the next word
start = end + 1; // Update the start index for the next word
}
}
if (high1 > 0) // If a word with repeated letters is found
{
string repeated;
// Extract the word with the highest number of repeated letters
for (temp1; temp1 < temp2; temp1++)
{
repeated.push_back(str[temp1]);
}
return repeated; // Return the word with the highest number of repeated letters
}
else
{
return "-1"; // Return -1 if no word with repeated letters is found
}
}
// Main function
int main() {
// Output the original strings and words with the highest number of repeated letters
cout << "Original string: Print a welcome text in a separate line.\nWord which has the highest number of repeated letters. ";
cout << highest_repeated_letters("Print a welcome text in a separate line.") << endl;
cout << "\nOriginal string: Count total number of alphabets, digits and special characters in a string.\nWord which has the highest number of repeated letters. ";
cout << highest_repeated_letters("Count total number of alphabets, digits and special characters in a string.") << endl;
cout << "\nOriginal string: abcdef abcdfgh ijklop 1234.\nWord which has the highest number of repeated letters. ";
cout << highest_repeated_letters("abcdef abcdfgh ijklop 1234") << endl; // Not found, returns -1
return 0; // Return 0 to indicate successful completion
}
Sample Output:
Original string: Print a welcome text in a separate line. Word which has the highest number of repeated letters. separate Original string: Count total number of alphabets, digits and special characters in a string. Word which has the highest number of repeated letters. characters Original string: abcdef abcdfgh ijklop 1234. Word which has the highest number of repeated letters. -1
Flowchart:
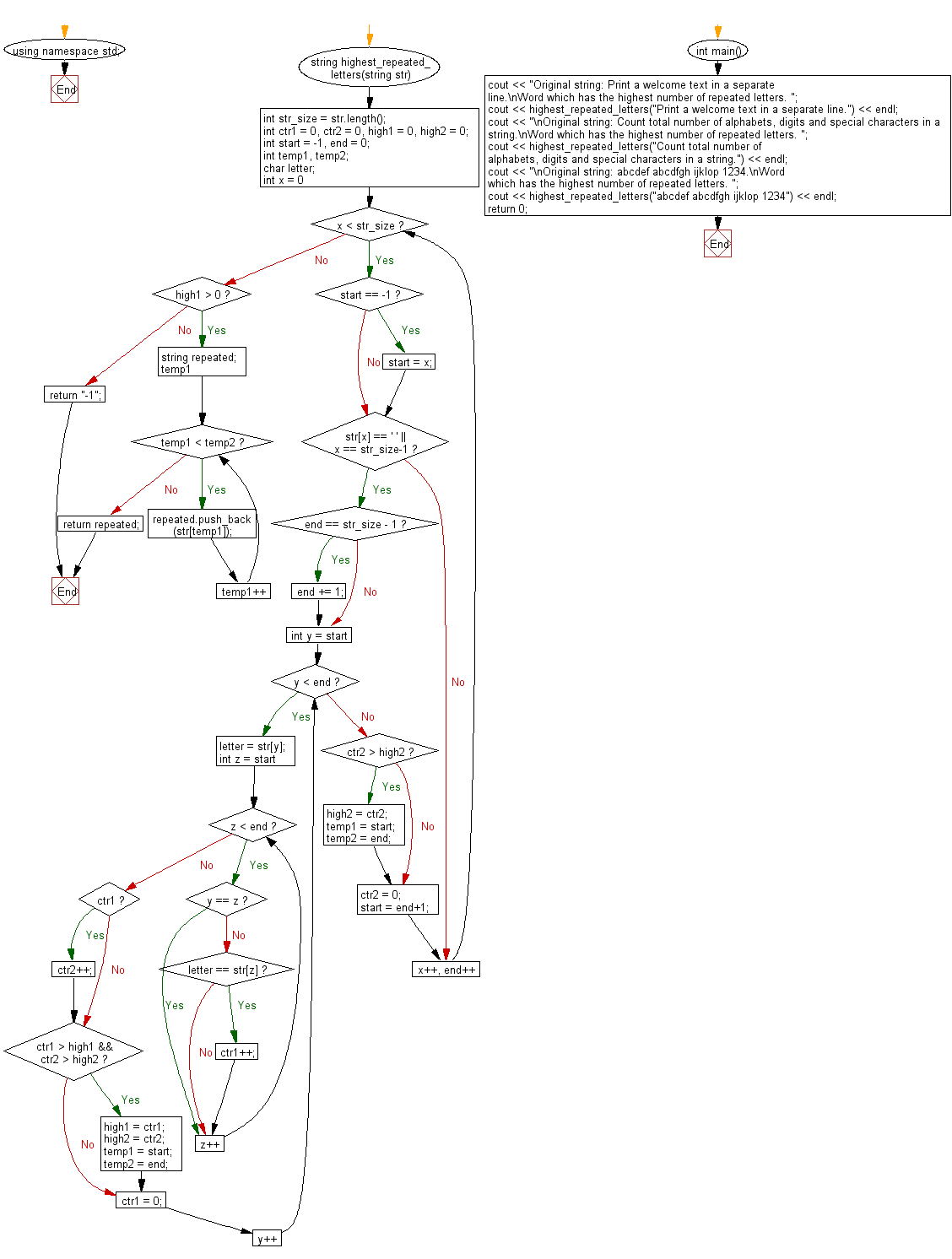
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Check if a given string is a Palindrome or not.
Next C++ Exercise: Insert a dash between two odd numbers in a string.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics