C++ Stack Exercises: Sort a stack using another stack
3. Sort a Stack Using an Array and an Auxiliary Stack
Write a C++ program to sort a given stack (using an array) using another stack.
Test Data:
Input some elements onto the stack:
Stack elements: 0 1 5 2 4 7
Sort the elements in the stack:
Display the sorted elements of the stack:
Stack elements: 0 1 2 4 5 7
Sample Solution:
C++ Code:
Sample Output:
Input some elements onto the stack: Stack elements: 0 1 5 2 4 7 Sort the elements in the stack: Display the sorted elements of the stack: Stack elements: 0 1 2 4 5 7 Remove two elements: Stack elements: 2 4 5 7 Input two more elements Stack elements: 10 -1 2 4 5 7 Sort the elements in the stack: Display the sorted elements of the stack: Stack elements: -1 2 4 5 7 10
Flowchart:
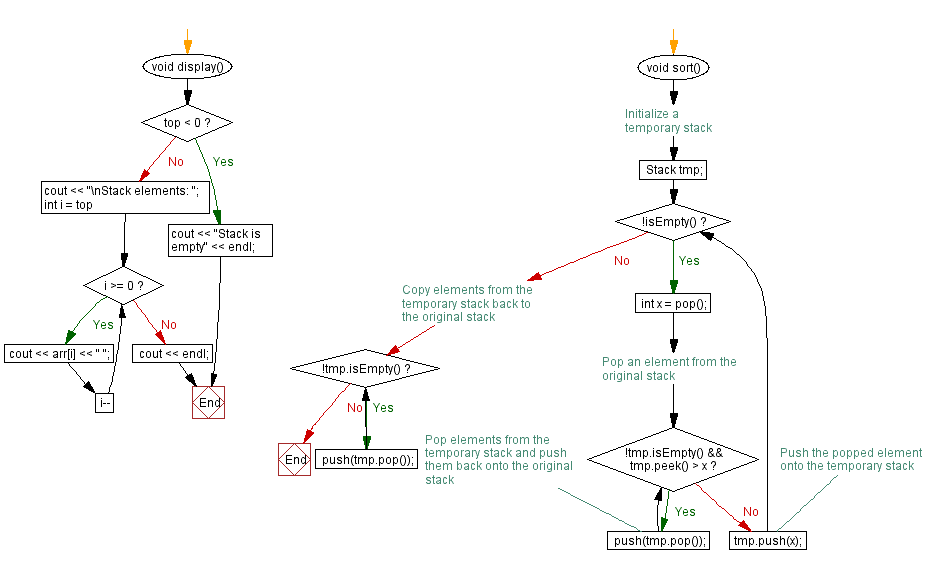
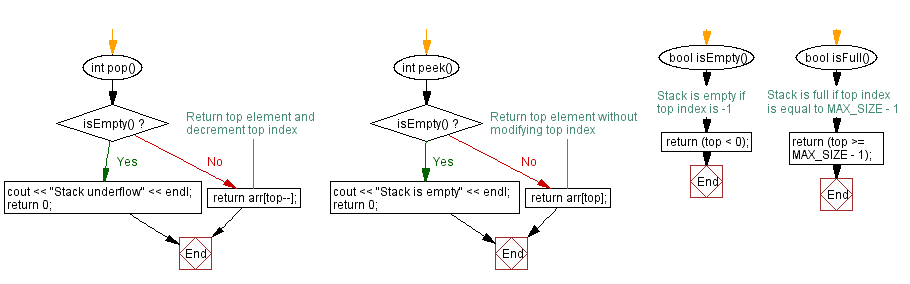
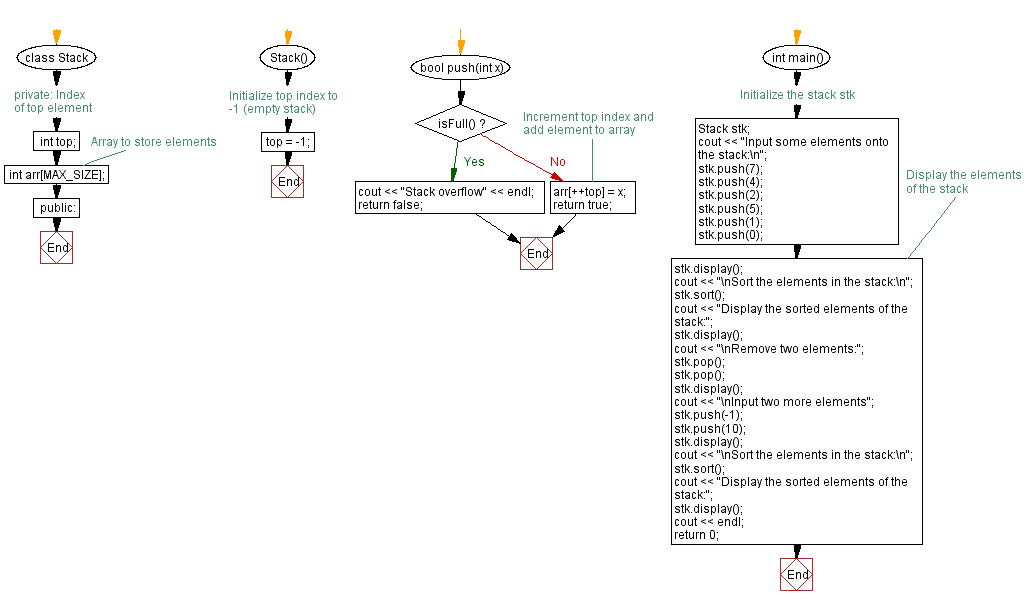
For more Practice: Solve these Related Problems:
- Write a C++ program to sort elements of a stack (implemented with an array) using a temporary auxiliary stack.
- Develop a C++ program that reads stack elements into an array, sorts them using an additional stack, and then displays the sorted stack.
- Design a C++ program to sort an array-based stack by transferring elements between two stacks until the order is ascending.
- Implement a C++ program that sorts a stack (using an array) by iteratively moving elements to an auxiliary stack based on their values.
CPP Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Implement a stack using an array with push, pop operations.
Next C++ Exercise: Reverse a stack (using an array) elements.
What is the difficulty level of this exercise?