C++ Stack Exercises: Remove duplicates from a stack using arrays
C++ Stack: Exercise-12 with Solution
Write a C++ program to remove duplicates from a stack using arrays.
Test Data:
Input some elements onto the stack:
Stack elements are: 5 2 2 4 7
Remove duplicates from the said stack:
Stack elements are: 5 2 4 7
Sample Solution:
C++ Code:
#include <iostream>
using namespace std;
#define MAX_SIZE 15 // Maximum size of stack
class Stack {
private:
int top; // Index of top element
int arr[MAX_SIZE]; // Array to store elements
public:
Stack() {
top = -1; // Initialize top index to -1 (empty stack)
}
bool push(int x) {
if (isFull()) {
cout << "Stack overflow" << endl; // Display message if stack is full
return false; // Return false to indicate failure in pushing element
}
// Increment top index and add element to array
arr[++top] = x;
return true; // Return true to indicate successful element addition
}
int pop() {
if (isEmpty()) {
cout << "Stack underflow" << endl; // Display message if stack is empty
return 0; // Return 0 to indicate failure in popping element
}
// Return top element and decrement top index
return arr[top--];
}
int peek() {
if (isEmpty()) {
cout << "Stack is empty" << endl; // Display message if stack is empty
return 0; // Return 0 to indicate failure in peeking element
}
// Return top element without modifying top index
return arr[top];
}
bool isEmpty() {
// Stack is empty if top index is -1
return (top < 0);
}
bool isFull() {
// Stack is full if top index is equal to MAX_SIZE - 1
return (top >= MAX_SIZE - 1);
}
void display() {
if (top < 0) {
cout << "Stack is empty" << endl; // Display message if stack is empty
return;
}
cout << "\nStack elements are: ";
for (int i = top; i >= 0; i--)
cout << arr[i] << " "; // Display elements of the stack
cout << endl;
}
// Function to remove duplicates from the stack
void remove_duplicates(Stack& stk);
};
void Stack::remove_duplicates(Stack& stk) {
if (stk.isEmpty()) {
cout << "Stack is empty." << endl; // Display message if the stack is empty
return;
}
int size = stk.top + 1; // Get the size of the stack
int temp[size]; // Create a temporary array to store stack elements
int count = 0; // Initialize count to 0
// The stack elements are copied into a temporary array
while (!stk.isEmpty()) {
temp[count++] = stk.pop(); // Pop elements from stack and store in temporary array
}
// Remove duplicates from the temporary array
for (int i = 0; i < count; i++) {
for (int j = i + 1; j < count; j++) {
if (temp[i] == temp[j]) {
// Duplicate elements are marked with -1
temp[j] = -1;
}
}
}
// Non-duplicate elements are pushed back onto the stack
for (int i = count - 1; i >= 0; i--) {
if (temp[i] != -1) {
stk.push(temp[i]); // Push non-duplicate elements back onto the original stack
}
}
}
int main() {
Stack stk;
cout << "Input some elements onto the stack:";
stk.push(7);
stk.push(4);
stk.push(2);
stk.push(2);
stk.push(5);
stk.display();
cout << "\nRemove duplicates from the said stack:";
stk.remove_duplicates(stk); // Remove duplicates from the stack
stk.display();
cout << "\nInput two more elements onto the stack:";
stk.push(7);
stk.push(5);
stk.display();
cout << "\nRemove duplicates from the said stack:";
stk.remove_duplicates(stk); // Remove duplicates from the stack
stk.display();
cout << endl;
}
Sample Output:
Input some elements onto the stack: Stack elements are: 5 2 2 4 7 Remove duplicates from the said stack: Stack elements are: 5 2 4 7 Input two more elements onto the stack: Stack elements are: 5 7 5 2 4 7 Remove duplicates from the said stack: Stack elements are: 5 7 2 4
Flowchart:
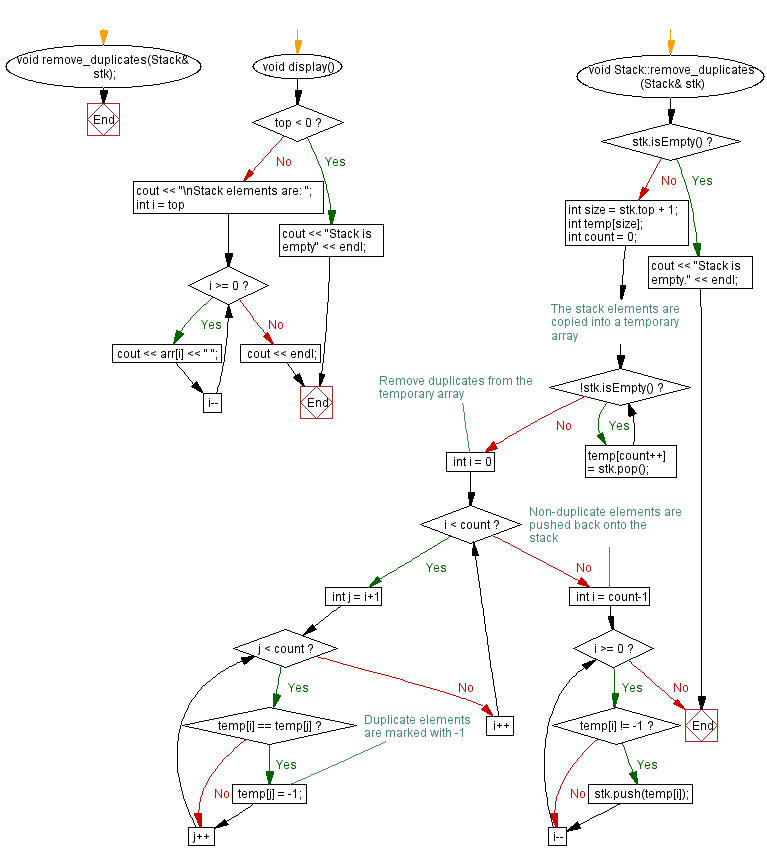
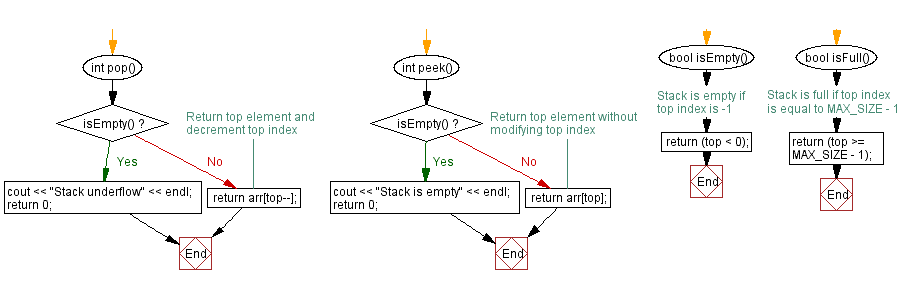
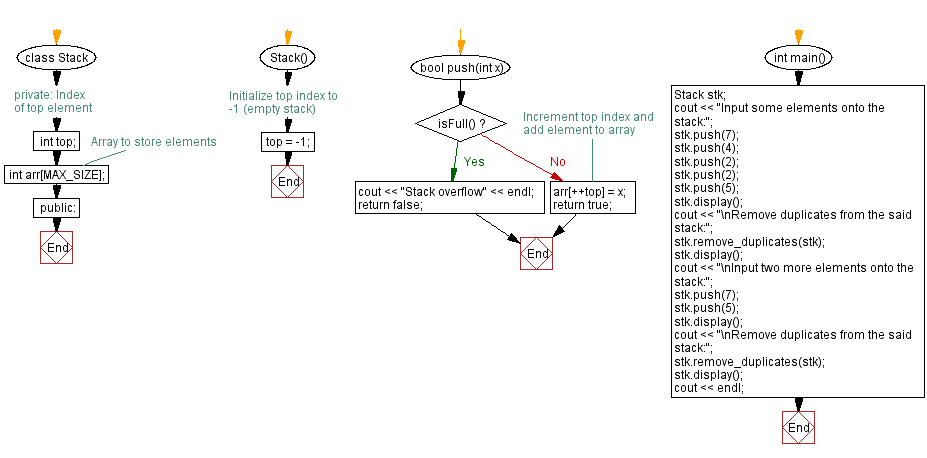
CPP Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Find and remove the lowest element in a stack.
Next C++ Exercise: Delete all occurrences of an item in a stack.
What is the difficulty level of this exercise?
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/cpp-exercises/stack/cpp-stack-exercise-12.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics