C++ Bogosort algorithm Exercise: Sort a list of numbers using Bogosort algorithm
C++ Sorting: Exercise-3 with Solution
Write a C++ program to sort a list of numbers using the Bogosort algorithm.
Sample Solution:
C++ Code :
// Including necessary C++ libraries
#include <algorithm>
#include <iostream>
#include <iterator>
#include <random>
// Function to perform bogo sort based on a custom predicate
template <typename RandomAccessIterator, typename Predicate>
void bogo_sort(RandomAccessIterator begin, RandomAccessIterator end,
Predicate p) {
// Random number generator setup
std::random_device rd;
std::mt19937 generator(rd());
// Loop until the range [begin, end) is sorted
while (!std::is_sorted(begin, end, p)) {
// Shuffle the elements in the range using the random number generator
std::shuffle(begin, end, generator);
}
}
// Function to perform bogo sort using the default 'less' comparison
template <typename RandomAccessIterator>
void bogo_sort(RandomAccessIterator begin, RandomAccessIterator end) {
// Utilizing the bogo_sort function with 'less' as the default comparison
bogo_sort(
begin, end,
std::less<
typename std::iterator_traits<RandomAccessIterator>::value_type>());
}
// Main function
int main() {
// Initializing an array of integers for sorting
int a[] = {125, 0, 695, 3, -256, -5, 214, 44, 55};
// Displaying the original numbers in the array
std::cout << "Original numbers:\n";
std::copy(std::begin(a), std::end(a), std::ostream_iterator<int>(std::cout, " "));
std::cout << "\n";
// Sorting the array using bogo_sort function
bogo_sort(std::begin(a), std::end(a));
// Displaying the sorted numbers after bogo sort
std::cout << "Sorted numbers:\n";
std::copy(std::begin(a), std::end(a), std::ostream_iterator<int>(std::cout, " "));
std::cout << "\n";
// Returning 0 to indicate successful execution
return 0;
}
Sample Output:
Original numbers: 125 0 695 3 -256 -5 214 44 55 Sorted numbers: -256 -5 0 3 44 55 125 214 695
Flowchart:
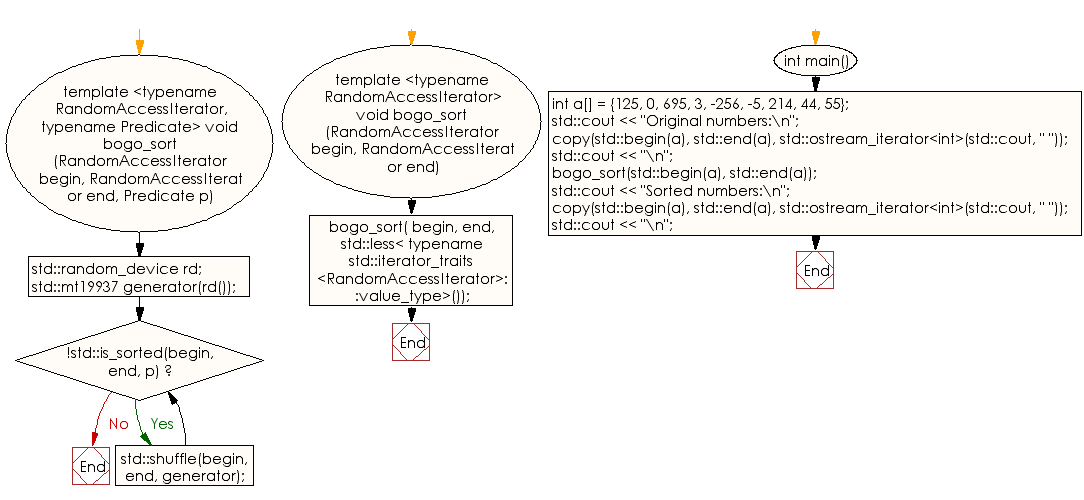
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to sort an array of positive integers using the Bead sort algorithm.
Next: Write a C++ program to sort an array of elements using the Bubble sort algorithm.
What is the difficulty level of this exercise?
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/cpp-exercises/sorting-and-searching/cpp-sorting-and-searching-exercise-3.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics