C++ Quick sort Exercise: Sort a collection of integers using the Quick sort
C++ Sorting: Exercise-12 with Solution
Write a C++ program to sort a collection of integers using Quick sort.
Sample Solution:
C++ Code :
#include <iostream>
// Function to perform quick sort
void quick_Sort(int *nums, int low, int high) {
// Initializing indices and pivot
int i = low;
int j = high;
int pivot = nums[(i + j) / 2];
int temp;
// Partitioning step of the quicksort algorithm
while (i <= j) {
// Finding elements less than the pivot on the left side
while (nums[i] < pivot)
i++;
// Finding elements greater than the pivot on the right side
while (nums[j] > pivot)
j--;
// Swapping elements to maintain order
if (i <= j) {
temp = nums[i];
nums[i] = nums[j];
nums[j] = temp;
i++;
j--;
}
}
// Recursive calls for the remaining partitions
if (j > low)
quick_Sort(nums, low, j);
if (i < high)
quick_Sort(nums, i, high);
}
// Main function
int main() {
// Initializing an array of integers for sorting
int nums[] = {125, 0, 695, 3, -256, -5, 214, 44, 55};
// Calculating the number of elements in the array
int n = sizeof(nums) / sizeof(nums[0]);
// Displaying the original numbers in the array
std::cout << "Before Quick Sort:" << std::endl;
for (int i = 0; i < n; ++i)
std::cout << nums[i] << " ";
// Calling the quicksort function
quick_Sort(nums, 0, n - 1);
// Displaying the sorted numbers after Quick Sort
std::cout << "\nAfter Quick Sort:" << std::endl;
for (int i = 0; i < n; ++i)
std::cout << nums[i] << " ";
return (0);
}
Sample Output:
Before Quick Sort: 125 0 695 3 -256 -5 214 44 55 After Quick Sort: -256 -5 0 3 44 55 125 214 695
Flowchart:
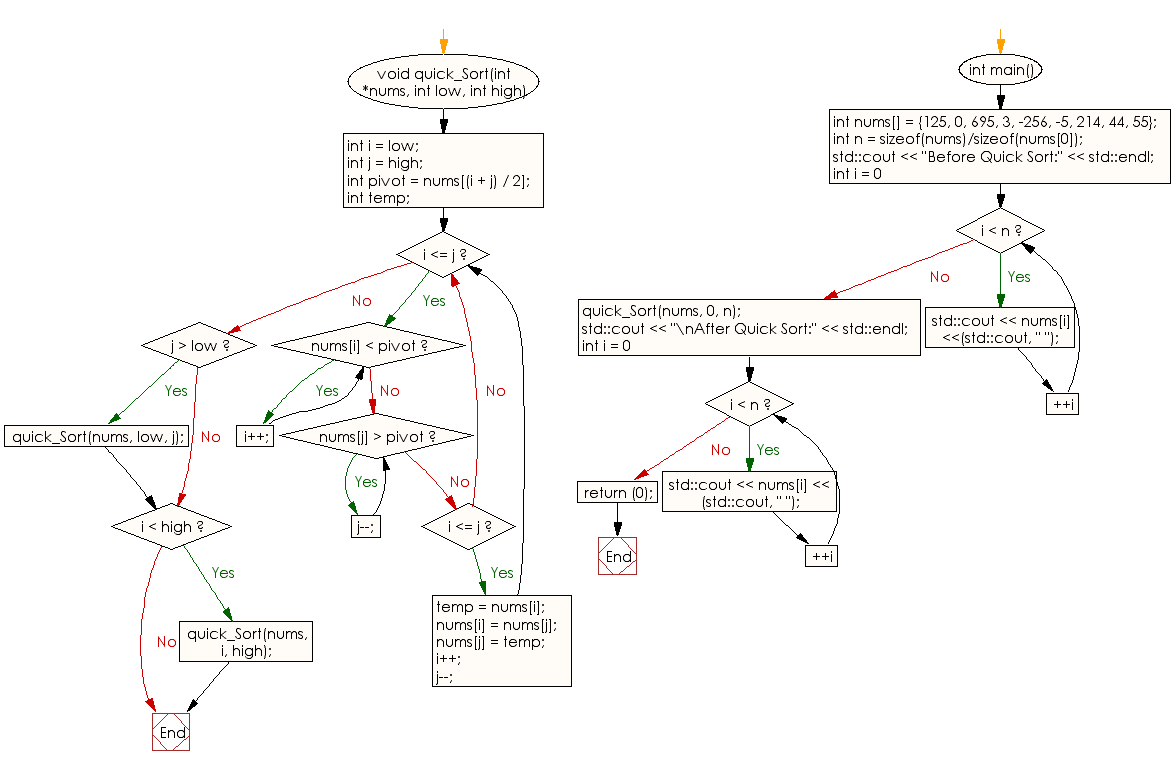
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to sort a collection of integers using the Pancake sort.
Next: Write a C++ program to sort a collection of integers using the Radix sort.
What is the difficulty level of this exercise?
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/cpp-exercises/sorting-and-searching/cpp-sorting-and-searching-exercise-12.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics