C++ Sorting and Searching Exercise: Sort the values of three variables which contain any value
C++ Sorting: Exercise-1 with Solution
Write a C++ program to sort the values of three variables which contain any value (numbers and/or literals).
Sample Solution:
C++ Code :
// Including necessary C++ libraries
#include <iostream>
#include <string>
#include <vector>
// Template function to sort three variables of type T
template <class T>
void sort3var(T& x, T& y, T& z) {
// Creating a vector to hold the variables
std::vector<T> v;
// Adding variables x, y, and z to the vector
v.push_back(x);
v.push_back(y);
v.push_back(z);
// Boolean flag to control the sorting loop
bool b = true;
// Sorting loop using a bubble sort algorithm
while (b) {
b = false;
// Iterating through the vector elements for sorting
for (size_t i = 0; i < v.size() - 1; i++) {
// Checking if the current element is greater than the next element
if (v[i] > v[i + 1]) {
// Swapping elements if the condition is met
T t = v[i];
v[i] = v[i + 1];
v[i + 1] = t;
// Setting flag to continue sorting
b = true;
}
}
}
// Assigning sorted values back to variables x, y, and z
x = v[0];
y = v[1];
z = v[2];
}
// Main function
int main(int argc, char* argv[]) {
// Initializing integer variables x, y, and z
int x = 2539, y = 0, z = -2560;
// Sorting integers x, y, and z using sort3var function and displaying them
sort3var(x, y, z);
std::cout << x << "\n" << y << "\n" << z << "\n\n";
// Initializing string variables xstr, ystr, and zstr
std::string xstr, ystr, zstr;
xstr = "abcd, ABC, xzy";
ystr = "abc @ example . com!";
zstr = "(from the \"Page of 123\")";
// Sorting strings xstr, ystr, and zstr using sort3var function and displaying them
sort3var(xstr, ystr, zstr);
std::cout << xstr << "\n" << ystr << "\n" << zstr << "\n\n";
// Initializing floating-point variables xnf, ynf, and znf
float xnf = 100.3f, ynf = -36.5f, znf = 12.15f;
// Sorting floats xnf, ynf, and znf using sort3var function and displaying them
sort3var(xnf, ynf, znf);
std::cout << xnf << "\n" << ynf << "\n" << znf << "\n\n";
// Returning 0 to indicate successful execution
return 0;
}
Sample Output:
-2560 0 2539 (from the "Page of 123") abc @ example . com! abcd, ABC, xzy -36.5 12.15 100.3
Flowchart:
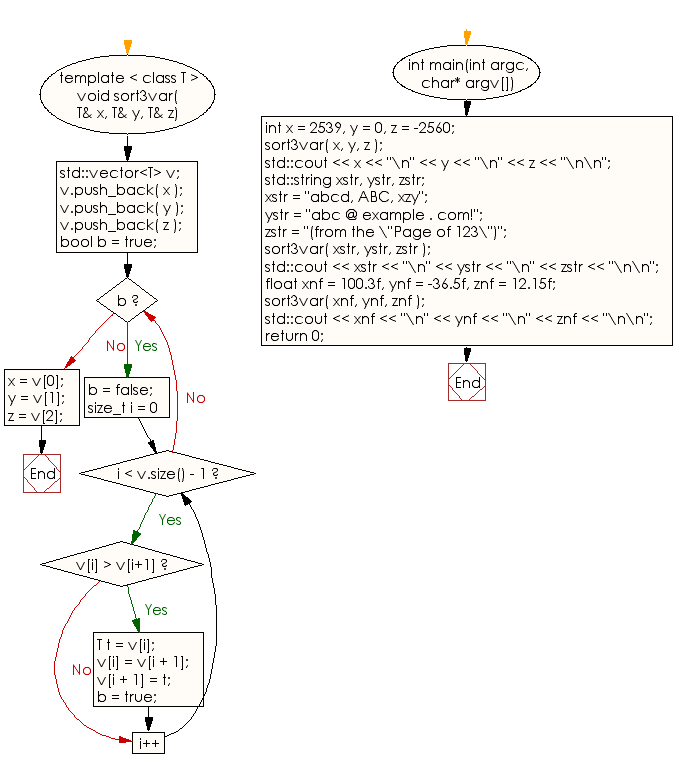
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: C++ Sorting and Searching Exercises Home
Next: Write a C++ program to sort an array of positive integers using the Bead sort algorithm.
What is the difficulty level of this exercise?
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/cpp-exercises/sorting-and-searching/cpp-sorting-and-searching-exercise-1.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics