C++ Recursion: Calculating the power of a number using recursive function
7. Power Calculation Using Recursion
Write a C++ program to implement a recursive function to calculate the power of a number.
Sample Solution:
C Code:
// Recursive function to calculate the power of a number
#include <iostream> // Including the Input/Output Stream Library
// Recursive function to calculate the power of a number
int power(int b, int e) {
// Base case: Any number raised to the power of 0 is 1
if (e == 0)
return 1;
// Recursive case: Calculate the power using recursion
// by multiplying the base with the power of (exponent - 1)
return b * power(b, e - 1);
}
int main() {
int b, e; // Declaring variables for base and exponent
std::cout << "Input the base number: ";
std::cin >> b; // Taking input for the base number
std::cout << "Input the exponent: ";
std::cin >> e; // Taking input for the exponent
// Calculate the power using recursion
int result = power(b, e); // Storing the result of the power calculation
// Display the result
std::cout << b << " raised to the power of " << e << " is: " << result << std::endl;
return 0; // Returning 0 to indicate successful execution of the program
}
Sample Output:
Input the base number: 2 Input the exponent: 3 2 raised to the power of 3 is: 8
Input the base number: 10 Input the exponent: 4 10 raised to the power of 4 is: 10000
Explanation:
In the above exercise,
- The "power()" function takes two integers base and exponent as parameters and calculates the power of the base number using recursion.
- The base case is when the exponent is 0, in which case the function returns 1 since any number raised to the power of 0 is 1.
- In the recursive case, the function calculates the power by multiplying the base with the power of (exponent - 1) using recursion.
- The "main()" function prompts the user to input the base number and the exponent, calls the "power()" function to calculate the power, and then displays the result.
Flowchart:
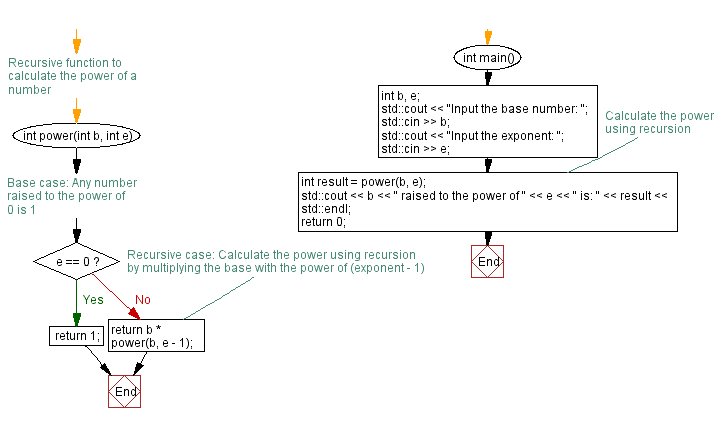
For more Practice: Solve these Related Problems:
- Write a C++ program to implement a recursive function that calculates the power of a number without using the multiplication operator in a loop.
- Write a C++ program to compute exponentiation recursively using divide and conquer to optimize large exponents.
- Write a C++ program that recursively computes the power of a number and prints the recursive calls for each intermediate step.
- Write a C++ program to calculate the power of a number using recursion and compare its efficiency with an iterative solution.
CPP Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Reversing a string using recursive function.
Next C++ Exercise: Checking palindrome strings using recursive function.
What is the difficulty level of this exercise?