C++ Recursion: Reversing a string using recursive function
6. Reverse a String Using Recursion
Write a C++ program to reverse a string using recursion.
Sample Solution:
C Code:
// Recursive function to reverse a string
#include <iostream> // Including the Input/Output Stream Library
#include <string> // Including the String Library
// Recursive function to reverse a string
void reverse_String(std::string & text, int start, int end) {
// Base case: when start >= end, the string is fully reversed
if (start >= end)
return;
// Swap characters at start and end indices
std::swap(text[start], text[end]);
// Recursive case: move to the next pair of characters
reverse_String(text, start + 1, end - 1);
}
int main() {
std::string text; // Declaring a string variable to store user input
std::cout << "Input a string: ";
std::getline(std::cin, text); // Taking a string input from the user
// Reverse the string using recursion
reverse_String(text, 0, text.length() - 1);
std::cout << "Reversed string: " << text << std::endl; // Displaying the reversed string
return 0; // Returning 0 to indicate successful execution of the program
}
Sample Output:
Input a string: C++ Reversed string: ++C
Input a string: String Reversed string: gnirtS
Input a string: madam Reversed string: madam
Explanation:
In the above exercise,
- The "reverse_String()" function takes a reference to a string (str), the starting index (start), and the ending index (end) as parameters.
- Use recursion to reverse the string. The base case is when start >= end, indicating the string is fully reversed.
- In the recursive case, the function swaps the characters at the start and end indices using the std::swap function and then recursively calls the "reverse_String()" function for the next pair of characters (start + 1 and end - 1).
- The "main()" function prompts the user to enter a string, calls "reverse_String()" to reverse the string, and then displays the reversed string.
Flowchart:
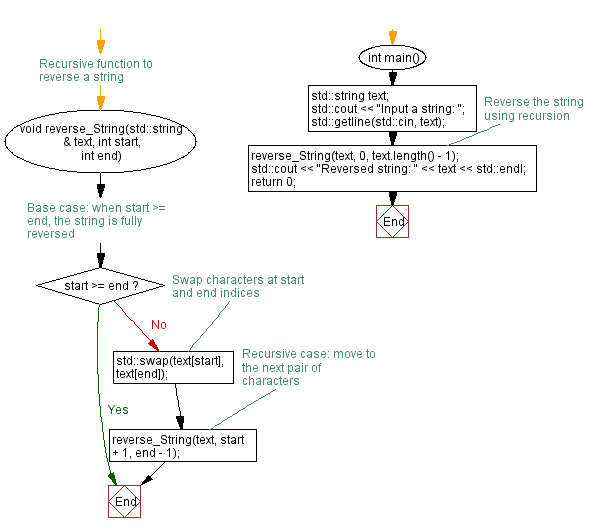
For more Practice: Solve these Related Problems:
- Write a C++ program to reverse a string recursively by swapping the first and last characters in each call.
- Write a C++ program to implement a recursive function that returns a reversed copy of a given string.
- Write a C++ program to reverse a string using recursion and then check if the reversed string is identical to the original.
- Write a C++ program that employs recursion to reverse a string and prints the state of the string at each recursive step.
CPP Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Maximum and minimum elements in an array.
Next C++ Exercise: Calculating the power of a number using recursive function.
What is the difficulty level of this exercise?