C++ Recursion: Maximum and minimum elements in an array
C++ Recursion: Exercise-5 with Solution
Write a C++ program to implement a recursive function to find the maximum and minimum elements in an array.
Sample Solution:
C Code:
// Recursive function to find the maximum and minimum elements in an array
#include <iostream> // Including the Input/Output Stream Library
// Recursive function to find the maximum element in the array
int findMax(int nums[], int start, int end) {
// Base case: when there is only one element, it is the maximum
if (start == end)
return nums[start];
// Recursive case: divide the array in half and find the maximum in each half,
// then return the maximum of the two halves
int mid = (start + end) / 2;
int max1 = findMax(nums, start, mid);
int max2 = findMax(nums, mid + 1, end);
return (max1 > max2) ? max1 : max2;
}
// Recursive function to find the minimum element in an array
int findMin(int nums[], int start, int end) {
// Base case: when there is only one element, it is the minimum
if (start == end)
return nums[start];
// Recursive case: Divide the array in half and find the minimum in each half,
// then return the minimum of the two halves
int mid = (start + end) / 2;
int min1 = findMin(nums, start, mid);
int min2 = findMin(nums, mid + 1, end);
return (min1 < min2) ? min1 : min2;
}
int main() {
int nums[] = { 9, 2, 4, 0, 2, 2, 3, 4, 5, 7 }; // Initializing an array with elements
int size = sizeof(nums) / sizeof(nums[0]); // Calculating the size of the array
std::cout << "Array elements: " << std::endl;
// Loop through the array elements and display them
for (size_t i = 0; i < size; i++) {
std::cout << nums[i] << ' ';
}
// Find the maximum and minimum elements in the array using recursion
int max = findMax(nums, 0, size - 1);
int min = findMin(nums, 0, size - 1);
std::cout << "\nMaximum element: " << max << std::endl; // Display the maximum element
std::cout << "Minimum element: " << min << std::endl; // Display the minimum element
return 0; // Returning 0 to indicate successful execution of the program
}
Sample Output:
Array elements: 9 2 4 0 2 2 3 4 5 7 Maximum element: 9 Minimum element: 0
Explanation:
In the above exercise,
- The "findMax()" function takes an array (nums), the starting index (start), and the ending index (end) as parameters.
- Use recursion to find the maximum element in the array.
- The base case is when there is only one element (start == end), in which case the function returns that element.
- In the recursive case, the function divides the array in half, finds the maximum in each half by recursively calling "findMax()", and returns the maximum of the two halves.
- Similarly, the "findMin()" function takes the same parameters and finds the minimum element in the array using a similar recursive approach.
- The "main()" function initializes an array, calculates its size, and then calls "findMax()" and "findMin()" to find the maximum and minimum elements, respectively.
- Finally, it displays the results.
Flowchart:
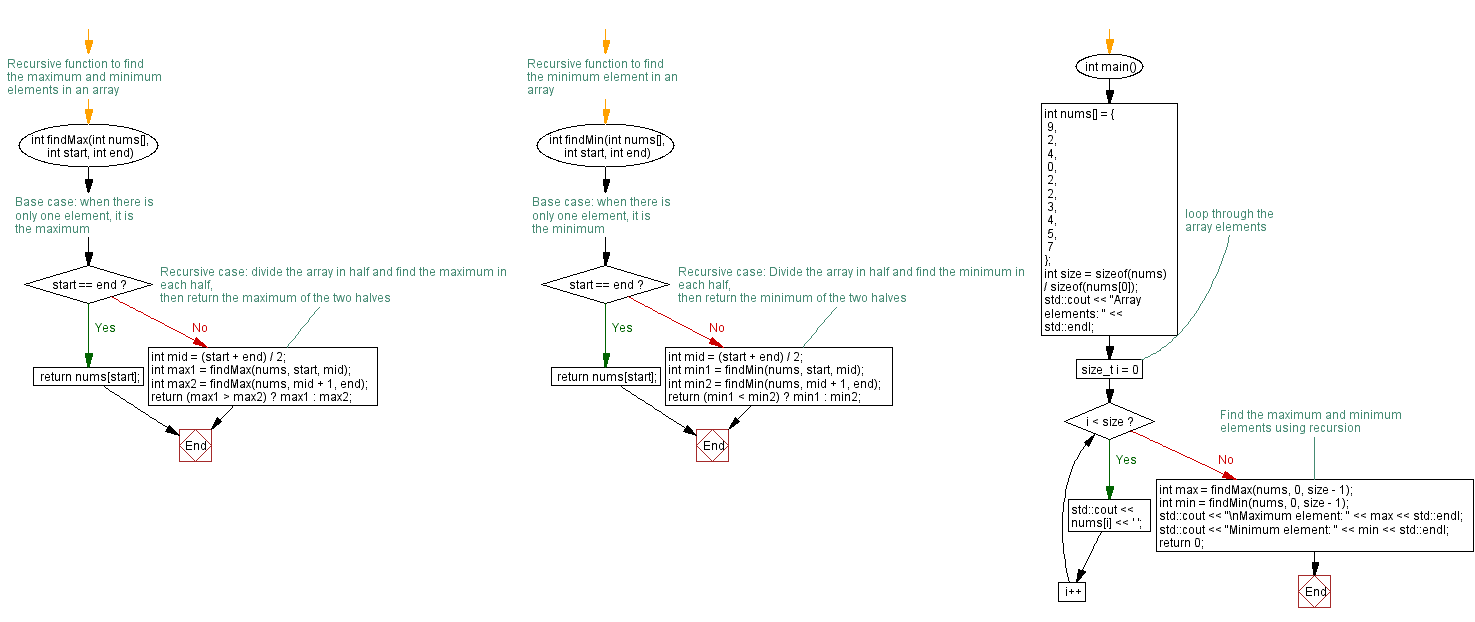
CPP Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Implementing recursive function for sum of digits.
Next C++ Exercise: Reversing a string using recursive function.
What is the difficulty level of this exercise?
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/cpp-exercises/recursion/cpp-recursion-exercise-5.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics