C++ Recursion: Implementing recursive function for sum of digits
C++ Recursion: Exercise-4 with Solution
Write a C++ program to implement a recursive function to calculate the sum of digits of a given number.
Sample Solution:
C Code:
// Recursive function to calculate the sum of digits
#include <iostream> // Including the Input/Output Stream Library
int sumOfDigits(int number) {
// If the number is a single digit, return the number itself
if (number >= 0 && number <= 9)
return number;
// Recursive case: Calculate the sum of the last digit and the sum of the rest of the digits
return (number % 10) + sumOfDigits(number / 10);
}
int main() {
int n;
std::cout << "Input a number: "; // Prompting the user to input a number
std::cin >> n; // Reading the input number from the user
// Calculate the sum of digits using recursion
int sum_digits = sumOfDigits(n); // Calling the recursive function to compute the sum of digits
std::cout << "Sum of digits of " << n << " is: " << sum_digits << std::endl; // Displaying the sum of digits
return 0; // Returning 0 to indicate successful execution of the program
}
Sample Output:
Input a number: 100 Sum of digits of 100 is: 1
Input a number: 123 Sum of digits of 123 is: 6
Input a number: 56459 Sum of digits of 56459 is: 29
Explanation:
In the above exercise,
- The sumOfDigits function takes an integer n as a parameter and calculates the sum of its digits using recursion.
- The base case is when the number is a single digit (0 to 9), in which case the function returns the number itself.
- In the recursive case, the function calculates the sum of the last digit (number % 10) and the sum of the rest of the digits (sumOfDigits(number / 10)).
- The main function prompts the user to enter a number, calculates the sum of its digits using the sumOfDigits function, and displays the result.
Flowchart:
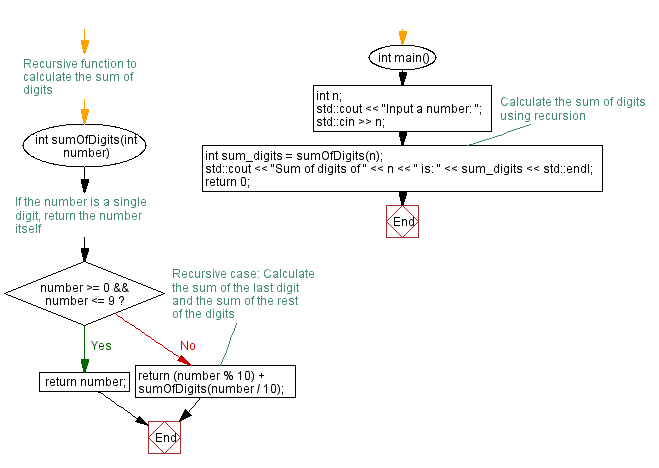
CPP Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Implementing recursive function for fibonacci numbers.
Next C++ Exercise: Maximum and minimum elements in an array.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics