C++ Recursion: Calculating factorial using recursive function
2. Factorial Using Recursion
Write a C++ program to calculate the factorial of a given number using recursion.
Sample Solution:
C Code:
#include <iostream> // Including the Input/Output Stream Library
// Recursive function to calculate the factorial
int factorial(int n) {
// Factorial of 0 or 1 is 1
if (n == 0 || n == 1)
return 1;
// Recursive case: factorial of n is n multiplied by factorial of (n-1)
return n * factorial(n - 1);
}
int main() {
int num;
std::cout << "Input a number: "; // Prompting the user to input a number
std::cin >> num; // Reading the input number from the user
// Calculate the factorial using recursion
int fact = factorial(num); // Calling the recursive function to calculate factorial
std::cout << "Factorial of " << num << " is: " << fact << std::endl; // Displaying the factorial
return 0; // Returning 0 to indicate successful execution of the program
}
Sample Output:
Input a number: 3 Factorial of 3 is: 6
Input a number: 10 Factorial of 10 is: 3628800
Explanation:
In the above exercise
- The "factorial()" function takes an integer n as a parameter and calculates the factorial of n using recursion.
- The base case is when n is 0 or 1, in which case the function returns 1.
- In the recursive case, the function returns n multiplied by n-1's factorial.
- The main function accepts a number from the user, calculates the factorial using the factorial function, and displays the result.
Flowchart:
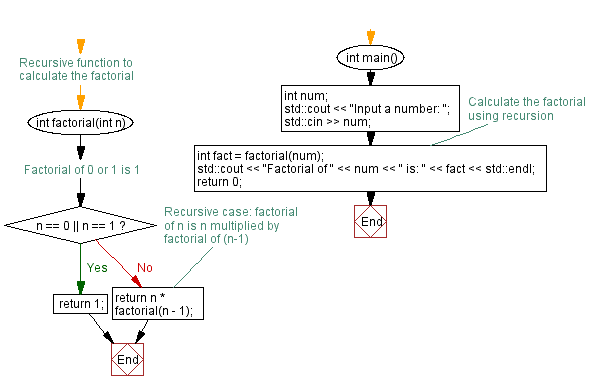
For more Practice: Solve these Related Problems:
- Write a C++ program to calculate the factorial of a number recursively and use memoization to optimize repeated calls.
- Write a C++ program that implements a tail-recursive factorial function to minimize stack usage.
- Write a C++ program to compute factorial recursively and print the intermediate multiplication steps for clarity.
- Write a C++ program to compute factorial using recursion while handling large input values with proper error checking.
CPP Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Sum of array elements using recursion.
Next C++ Exercise: Implementing recursive function for fibonacci numbers.
What is the difficulty level of this exercise?