C++ Recursion: Calculating product of two numbers without multiplication operator
13. Recursive Multiplication Without Using the Multiplication Operator
Write a C++ program to implement a recursive function to calculate the product of two numbers without using the multiplication operator.
Sample Solution:
C Code:
Sample Output:
Input the first number: 12 Input the second number: 34 Product of 12 and 34: 408
Explanation:
In the above exercise,
- The "multiply()" function takes two integers x and y as parameters and calculates their product without using the multiplication operator.
- The base case is when either x or y is 0, in which case the function returns 0.
- In the recursive case, if y is positive, the function recursively adds x to the product of x and y - 1. If y is negative, the function recursively adds -x to the product of x and y + 1.
- The recursive calls continue until the base case is reached, and the function returns the final product.
- The "main()" function prompts the user to enter two numbers, calls the "multiply()" function to calculate their product, and then displays the result.
Flowchart:
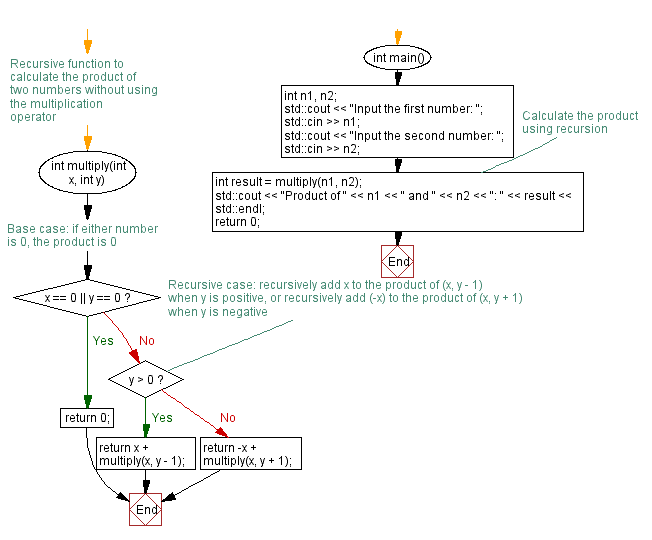
For more Practice: Solve these Related Problems:
- Write a C++ program to recursively multiply two numbers using addition and subtraction only.
- Write a C++ program that implements a recursive function to compute the product of two integers without using the '*' operator.
- Write a C++ program to simulate multiplication recursively by adding one number repeatedly and handling negative values.
- Write a C++ program that calculates the product of two numbers using recursion and compares the result with standard multiplication.
CPP Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Counting occurrences of an element in an array with recursive function.
Next C++ Exercise: Calculating the sum of even and odd numbers in a range.
What is the difficulty level of this exercise?