C++ Queue Exercises: Find the mode of all elements of a queue
C++ Queue: Exercise-7 with Solution
Write a C++ program to find the mode of all elements of a queue.
Sample Solution:
C Code:
#include <iostream>
#include <map> // Include map library for using map data structure
using namespace std;
const int MAX_SIZE = 100;
class Queue {
private:
int front; // Front index of the queue
int rear; // Rear index of the queue
int arr[MAX_SIZE]; // Array to store elements
public:
Queue() {
front = -1; // Initialize front index to -1
rear = -1; // Initialize rear index to -1
}
bool isFull() {
return (rear == MAX_SIZE - 1); // Check if the queue is full
}
bool isEmpty() {
return (front == -1 && rear == -1); // Check if the queue is empty
}
void enqueue(int x) {
if (isFull()) {
cout << "Error: Queue is full" << endl; // Display error message if queue is full
return;
}
if (isEmpty()) {
front = 0;
rear = 0;
} else {
rear++;
}
arr[rear] = x; // Insert the element at the rear index
}
void dequeue() {
if (isEmpty()) {
cout << "Error: Queue is empty" << endl; // Display error message if queue is empty
return;
}
if (front == rear) {
front = -1;
rear = -1;
} else {
front++;
}
}
int peek() {
if (isEmpty()) {
cout << "Error: Queue is empty" << endl; // Display error message if queue is empty
return -1;
}
return arr[front]; // Return the element at the front of the queue
}
void display() {
if (isEmpty()) {
cout << "Error: Queue is empty" << endl; // Display error message if queue is empty
return;
}
cout << "Queue elements are: ";
for (int i = front; i <= rear; i++) {
cout << arr[i] << " "; // Display all elements in the queue
}
cout << endl;
}
int find_Mode() {
map < int, int > freq; // Map to store element frequency
int mode = -1; // Initialize mode to -1
int maxFreq = -1; // Initialize maximum frequency to -1
for (int i = front; i <= rear; i++) {
freq[arr[i]]++; // Increment frequency count for each element in the queue
if (freq[arr[i]] > maxFreq) {
maxFreq = freq[arr[i]]; // Update maximum frequency
mode = arr[i]; // Update mode to the element with maximum frequency
}
}
return mode; // Return the mode of the elements in the queue
}
};
int main() {
cout << "Initialize a Queue." << endl;
Queue q;
cout << "\nInsert some elements into the queue:" << endl;
q.enqueue(1);
q.enqueue(2);
q.enqueue(3);
q.enqueue(4);
q.enqueue(4);
q.display();
// Find and display the mode of elements in the queue
int mode_val = q.find_Mode();
cout << "Find the mode of all elements of the said queue: " << mode_val;
cout << "\n\nInput one more element into the queue:" << endl;
q.enqueue(3);
q.enqueue(3);
q.display();
// Find and display the mode of elements in the queue after adding new elements
mode_val = q.find_Mode();
cout << "Find the mode of all elements of the said queue: " << mode_val;
return 0;
}
Sample Output:
Initialize a Queue. Insert some elements into the queue: Queue elements are: 1 2 3 4 4 Find the mode of all elements of the said queue: 4 Input one more element into the queue: Queue elements are: 1 2 3 4 4 3 3 Find the mode of all elements of the said queue: 3
Flowchart:
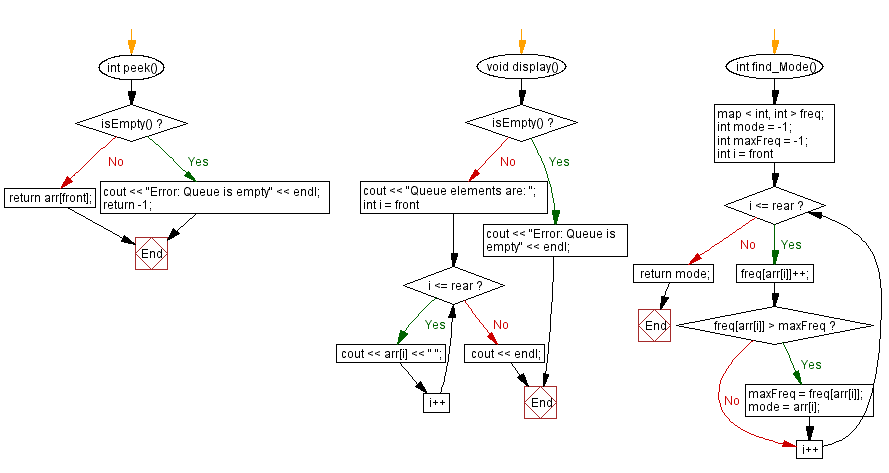
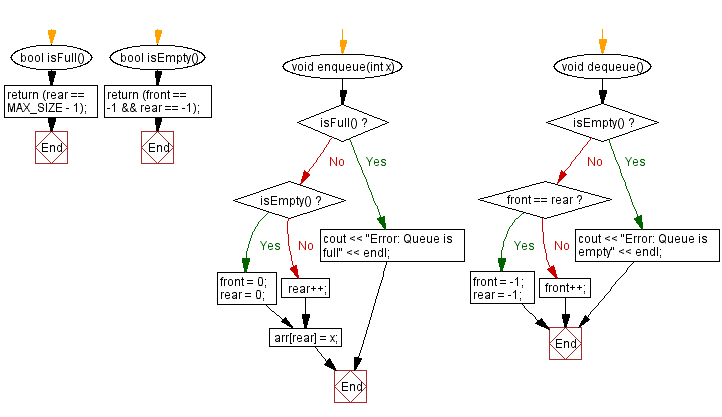
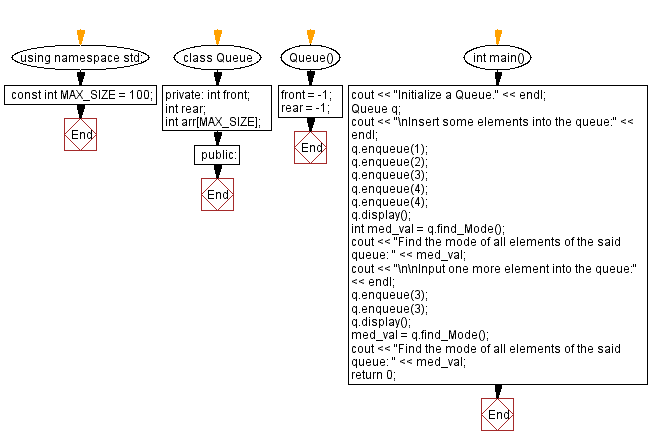
CPP Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Find the median of all elements of the said queue.
Next C++ Exercise: Mean, variance and standard deviation of all elements of a queue.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics