C++ Queue Exercises: Sort the elements of a queue
3. Sort Queue Elements
Write a C++ program to sort the elements of a queue.
Sample Solution:
C Code:
#include <iostream>
#include <stack>
using namespace std;
const int MAX_SIZE = 100;
class Queue {
private:
int front; // Front index of the queue
int rear; // Rear index of the queue
int arr[MAX_SIZE]; // Array to store elements
public:
Queue() {
front = -1; // Initialize front index to -1
rear = -1; // Initialize rear index to -1
}
bool isFull() {
return (rear == MAX_SIZE - 1); // Check if the queue is full
}
bool isEmpty() {
return (front == -1 && rear == -1); // Check if the queue is empty
}
void enqueue(int x) {
if (isFull()) {
cout << "Error: Queue is full" << endl; // Display error message if queue is full
return;
}
if (isEmpty()) {
front = 0;
rear = 0;
} else {
rear++;
}
arr[rear] = x; // Insert the element at the rear index
}
void dequeue() {
if (isEmpty()) {
cout << "Error: Queue is empty" << endl; // Display error message if queue is empty
return;
}
if (front == rear) {
front = -1;
rear = -1;
} else {
front++;
}
}
int peek() {
if (isEmpty()) {
cout << "Error: Queue is empty" << endl; // Display error message if queue is empty
return -1;
}
return arr[front]; // Return the element at the front of the queue
}
void display() {
if (isEmpty()) {
cout << "Error: Queue is empty" << endl; // Display error message if queue is empty
return;
}
cout << "Queue elements are: ";
for (int i = front; i <= rear; i++) {
cout << arr[i] << " "; // Display all elements in the queue
}
cout << endl;
}
// Function to sort the elements of the queue in ascending order
void sortQueue(Queue & q) {
if (q.isEmpty()) {
cout << "Error: Queue is empty" << endl; // Display error message if queue is empty
return;
}
int n = q.rear - q.front + 1; // Calculate the number of elements in the queue
for (int i = 0; i < n - 1; i++) {
int minIndex = i;
int minValue = q.arr[q.front + i];
// Find the index and value of the minimum element in the unsorted portion of the queue
for (int j = i + 1; j < n; j++) {
if (q.arr[q.front + j] < minValue) {
minIndex = j;
minValue = q.arr[q.front + j];
}
}
// Shift elements to the right to make space for the minimum element
for (int j = minIndex; j > i; j--) {
q.arr[q.front + j] = q.arr[q.front + j - 1];
}
q.arr[q.front + i] = minValue; // Place the minimum value in the correct sorted position
}
}
};
int main() {
cout << "Initialize a Queue." << endl;
Queue q;
cout << "\nInsert some elements into the queue:" << endl;
q.enqueue(1);
q.enqueue(5);
q.enqueue(3);
q.enqueue(6);
q.enqueue(2);
q.enqueue(0);
q.display();
q.sortQueue(q); // Sort the elements of the queue
cout << "\nSort the above Queue elements:\n";
q.display();
cout << "\nInput two more elements into the queue:" << endl;
q.enqueue(-1);
q.enqueue(4);
q.display();
q.sortQueue(q); // Sort the elements of the queue
cout << "\nSort the above Queue elements:\n";
q.display();
return 0;
}
Sample Output:
Initialize a Queue. Insert some elements into the queue: Queue elements are: 1 5 3 6 2 0 Sort the above Queue elements: Queue elements are: 0 1 2 3 5 6 Input two more elements into the queue: Queue elements are: 0 1 2 3 5 6 -1 4 Sort the above Queue elements: Queue elements are: -1 0 1 2 3 4 5 6
Flowchart:
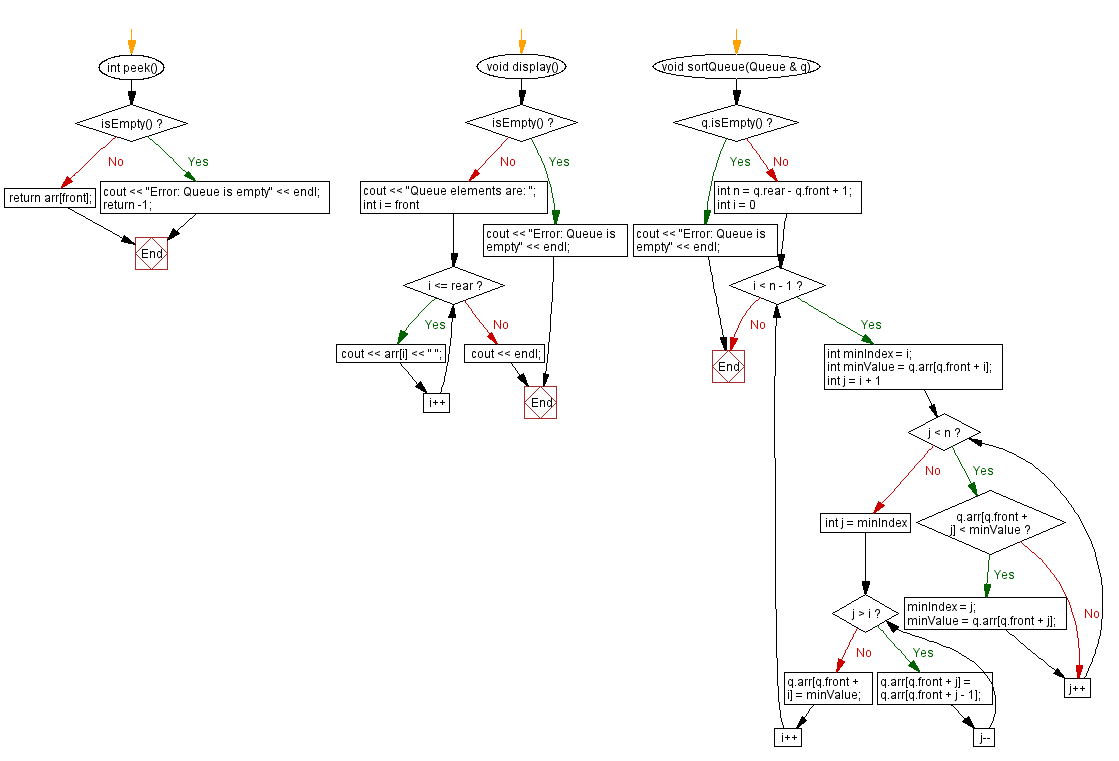
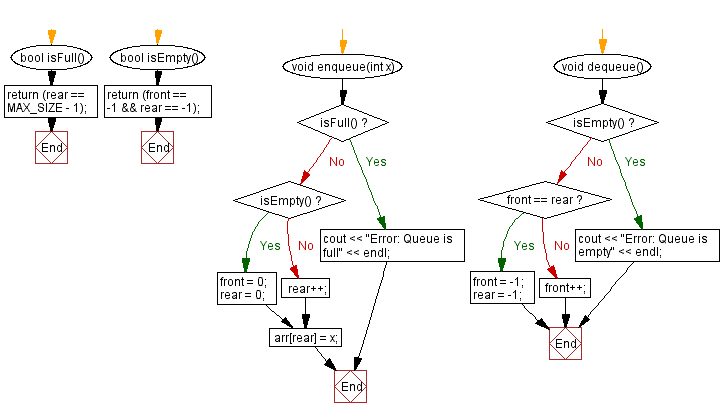
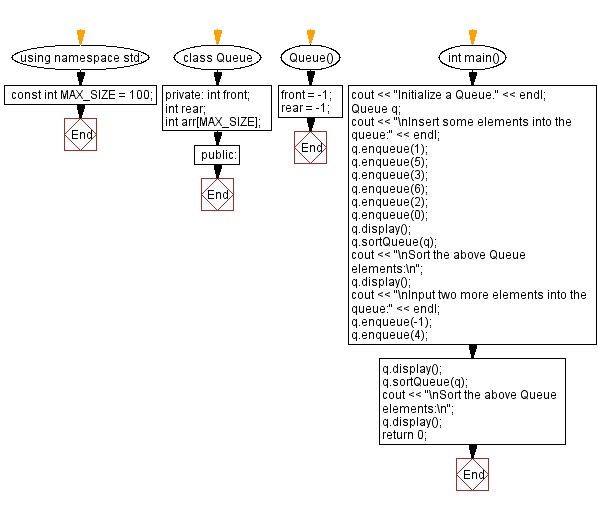
For more Practice: Solve these Related Problems:
- Write a C++ program to sort a queue in ascending order by using an auxiliary priority queue.
- Write a C++ program to rearrange the elements of a queue in descending order using bubble sort.
- Write a C++ program to sort the elements of a queue with a custom comparator function for non-numeric data.
- Write a C++ program that sorts a queue by repeatedly dequeuing and enqueuing elements until the order is achieved.
CPP Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Reverse the elements of a queue.
Next C++ Exercise: Find the sum of all elements of a queue.
What is the difficulty level of this exercise?