C++ Queue Exercises: Check if two queues are equal
25. Check if Two Queues are Equal
Write a C++ program to check if two queues are equal.
Sample Solution:
C Code:
#include <iostream> // Including necessary library for input and output operations
using namespace std;
const int MAX_SIZE = 100; // Maximum size for the queue
class Queue {
private:
int front; // Front of the queue
int rear; // Rear of the queue
int arr[MAX_SIZE]; // Array to store queue elements
public:
Queue() {
front = -1; // Initializing front index to -1
rear = -1; // Initializing rear index to -1
}
bool isFull() {
return (rear == MAX_SIZE - 1); // Check if the queue is full
}
bool isEmpty() {
return (front == -1 && rear == -1); // Check if the queue is empty
}
void enqueue(int x) {
if (isFull()) {
cout << "Error: Queue is full" << endl; // Display error if the queue is full
return;
}
if (isEmpty()) {
front = 0;
rear = 0;
} else {
rear++;
}
arr[rear] = x; // Add an element to the rear of the queue
}
void dequeue() {
if (isEmpty()) {
cout << "Error: Queue is empty" << endl; // Display error if the queue is empty
return;
}
if (front == rear) {
front = -1;
rear = -1;
} else {
front++;
}
}
int peek() {
if (isEmpty()) {
cout << "Error: Queue is empty" << endl; // Display error if the queue is empty
return -1;
}
return arr[front]; // Return the element at the front of the queue
}
bool isEqual(Queue q1, Queue q2) {
if (q1.isEmpty() && q2.isEmpty()) {
return true; // Return true if both queues are empty
}
if (q1.isEmpty() || q2.isEmpty()) {
return false; // Return false if only one of the queues is empty
}
if (q1.peek() != q2.peek()) {
return false; // Return false if the front elements of the queues are not equal
}
Queue temp1 = q1;
Queue temp2 = q2;
while (!temp1.isEmpty() && !temp2.isEmpty()) {
if (temp1.peek() != temp2.peek()) {
return false; // Return false if any corresponding elements of queues are not equal
}
temp1.dequeue();
temp2.dequeue();
}
return (temp1.isEmpty() && temp2.isEmpty()); // Return true if both queues are empty after comparisons
}
void display() {
if (isEmpty()) {
cout << "Error: Queue is empty" << endl; // Display error if the queue is empty
return;
}
cout << "Queue elements are: ";
for (int i = front; i <= rear; i++) {
cout << arr[i] << " "; // Display all elements in the queue
}
cout << endl;
}
};
int main() {
cout << "Initialize three Queues." << endl;
Queue q1, q2, q3; // Creating instances of Queue
q1.enqueue(1);
q1.enqueue(2);
q1.enqueue(3);
q2.enqueue(1);
q2.enqueue(2);
q2.enqueue(3);
q3.enqueue(3);
q3.enqueue(2);
q3.enqueue(1);
cout << "Queue-1" << endl;
q1.display(); // Display elements of Queue-1
cout << "Queue-2" << endl;
q2.display(); // Display elements of Queue-2
cout << "Queue-3" << endl;
q3.display(); // Display elements of Queue-3
cout << "\nCheck if Queue-1 and Queue-2 are equal? " << q1.isEqual(q1, q2) << endl;
cout << "\nCheck if Queue-2 and Queue-3 are equal? " << q1.isEqual(q2, q3) << endl;
cout << "\nCheck if Queue-1 and Queue-3 are equal? " << q1.isEqual(q1, q3) << endl;
return 0;
}
Sample Output:
Initialize three Queues. Queue-1 Queue elements are: 1 2 3 Queue-2 Queue elements are: 1 2 3 Queue-3 Queue elements are: 3 2 1 Cheque Queue-1 and Queue-2 are equal? 1 Cheque Queue-2 and Queue-3 are equal? 0 Cheque Queue-1 and Queue-3 are equal? 0
Flowchart:
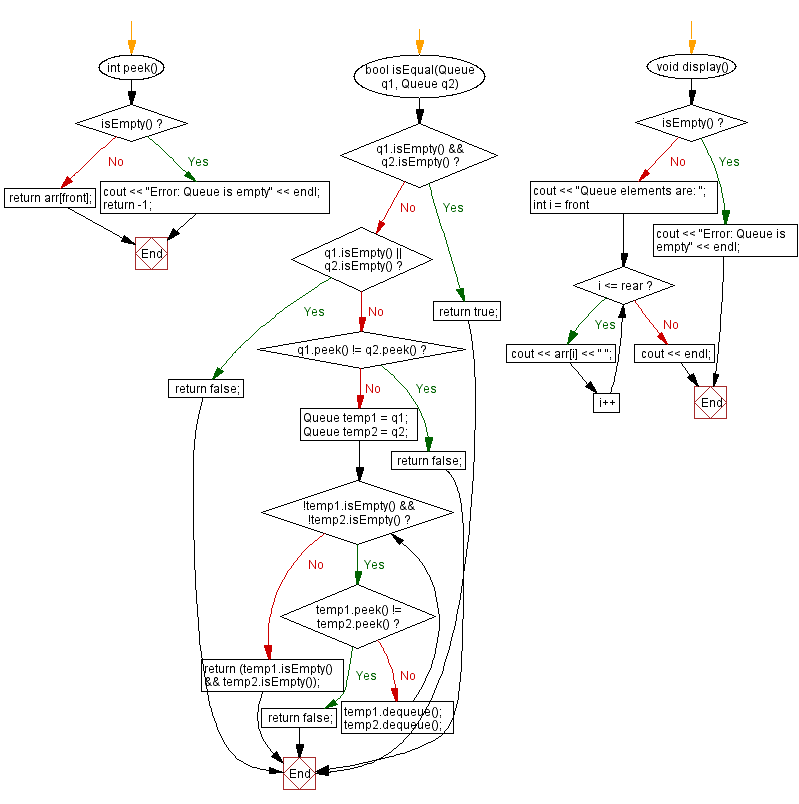
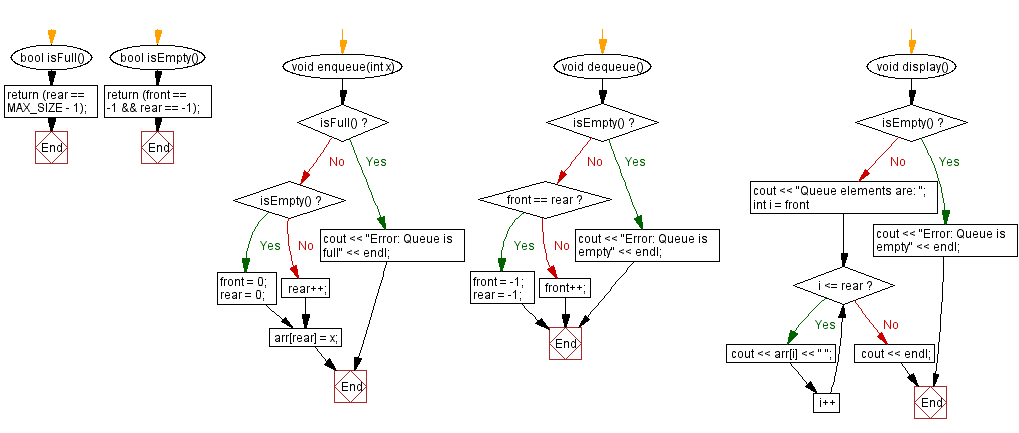
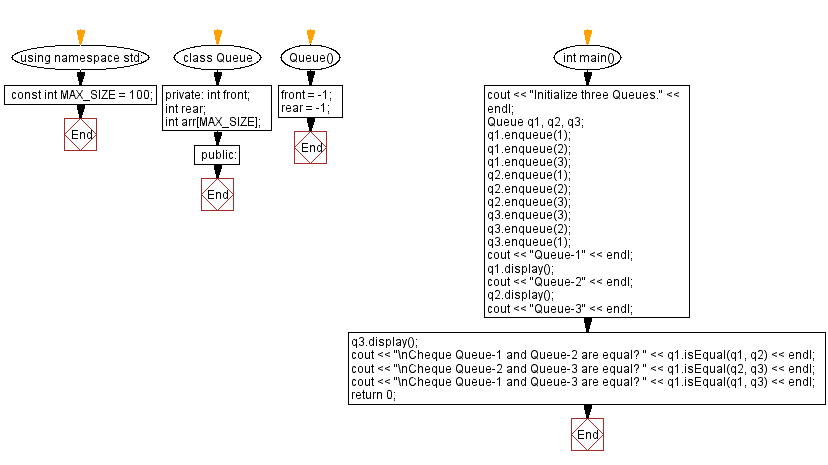
For more Practice: Solve these Related Problems:
- Write a C++ program to compare two queues for equality by checking element-by-element correspondence.
- Write a C++ program to determine if two queues contain the same elements in the same order.
- Write a C++ program that takes two queues as input and outputs whether they are identical without modifying them.
- Write a C++ program to implement an equality check for two queues using a temporary buffer for comparison.
CPP Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Find the position of an element in a queue.
Next C++ Exercise: Intersection of two queues.
What is the difficulty level of this exercise?