C++ Queue Exercises: Find the position of an element in a queue
C++ Queue: Exercise-24 with Solution
Write a C++ program to find the position of an element in a queue.
Sample Solution:
C Code:
#include <iostream> // Including necessary library for input and output operations
using namespace std;
const int MAX_SIZE = 100; // Maximum size for the queue
class Queue {
private:
int front; // Front of the queue
int rear; // Rear of the queue
int arr[MAX_SIZE]; // Array to store queue elements
public:
Queue() {
front = -1; // Initializing front index to -1
rear = -1; // Initializing rear index to -1
}
bool isFull() {
return (rear == MAX_SIZE - 1); // Check if the queue is full
}
bool isEmpty() {
return (front == -1 && rear == -1); // Check if the queue is empty
}
void enqueue(int x) {
if (isFull()) {
cout << "Error: Queue is full" << endl; // Display error if the queue is full
return;
}
if (isEmpty()) {
front = 0;
rear = 0;
} else {
rear++;
}
arr[rear] = x; // Add an element to the rear of the queue
}
void dequeue() {
if (isEmpty()) {
cout << "Error: Queue is empty" << endl; // Display error if the queue is empty
return;
}
if (front == rear) {
front = -1;
rear = -1;
} else {
front++;
}
}
int peek() {
if (isEmpty()) {
cout << "Error: Queue is empty" << endl; // Display error if the queue is empty
return -1;
}
return arr[front]; // Return the element at the front of the queue
}
int find_position(Queue q, int x) {
int pos = 0;
Queue temp;
// Traverse the queue and search for the element
while (!q.isEmpty()) {
pos++;
int curr = q.peek();
q.dequeue();
temp.enqueue(curr);
if (curr == x) {
// Element found, restore the queue and return the position
while (!temp.isEmpty()) {
q.enqueue(temp.peek());
temp.dequeue();
}
return pos;
}
}
// Element not found, restore the queue and return -1
while (!temp.isEmpty()) {
q.enqueue(temp.peek());
temp.dequeue();
}
cout << "Error: Element " << x << " not found in queue." << endl;
return -1;
}
void display() {
if (isEmpty()) {
cout << "Error: Queue is empty" << endl; // Display error if the queue is empty
return;
}
cout << "Queue elements are: ";
for (int i = front; i <= rear; i++) {
cout << arr[i] << " "; // Display all elements in the queue
}
cout << endl;
}
};
int main() {
cout << "Initialize a Queue." << endl;
Queue q1; // Creating an instance of Queue
cout << "\nInsert some elements into the queue:" << endl;
q1.enqueue(0);
q1.enqueue(1);
q1.enqueue(2);
q1.enqueue(3);
q1.enqueue(4);
q1.enqueue(5);
q1.enqueue(6);
q1.display(); // Display elements of the queue
int x = 2;
cout << "\nPosition of the element x = " << x << " is " << q1.find_position(q1, x) << endl;
x = 3;
cout << "\nPosition of the element x = " << x << " is " << q1.find_position(q1, x) << endl;
x = 6;
cout << "\nPosition of the element x = " << x << " is " << q1.find_position(q1, x) << endl;
return 0;
}
Sample Output:
Initialize a Queue. Insert some elements into the queue: Queue elements are: 0 1 2 3 4 5 6 Position of the element x = 2 is 3 Position of the element x = 3 is 4 Position of the element x = 6 is 7
Flowchart:
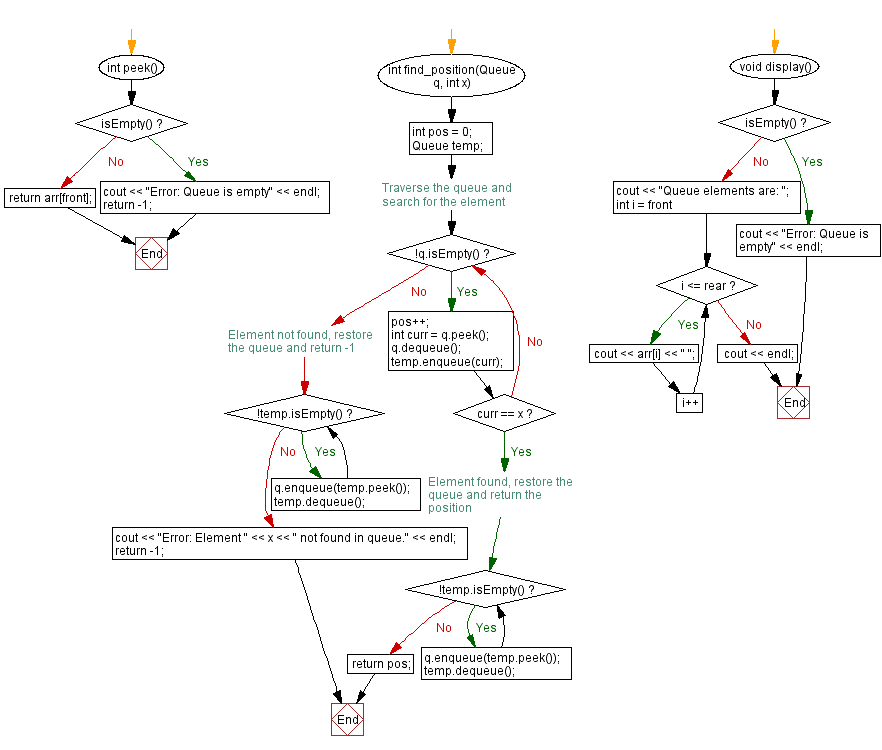
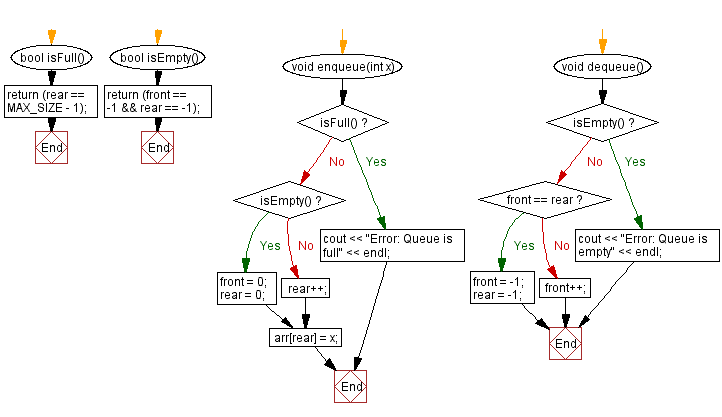
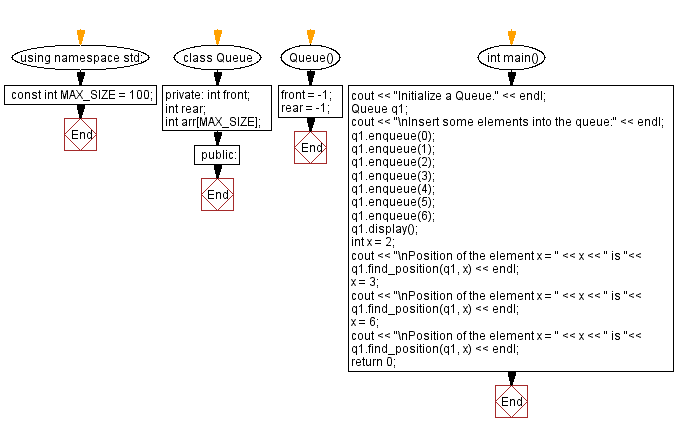
CPP Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Nth element from the top of a queue.
Next C++ Exercise: Check if two queues are equal.
What is the difficulty level of this exercise?
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/cpp-exercises/queue/cpp-queue-exercise-24.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics