C++ Queue Exercises: Nth element from the top of a queue
23. Get the Nth Element from the Top of a Queue
Write a C++ program to get the Nth element from the top of a queue.
Sample Solution:
C Code:
#include <iostream> // Including necessary library for input and output operations
using namespace std;
const int MAX_SIZE = 100; // Maximum size for the queue
class Queue {
private:
int front; // Front of the queue
int rear; // Rear of the queue
int arr[MAX_SIZE]; // Array to store queue elements
public:
Queue() {
front = -1; // Initializing front index to -1
rear = -1; // Initializing rear index to -1
}
bool isFull() {
return (rear == MAX_SIZE - 1); // Check if the queue is full
}
bool isEmpty() {
return (front == -1 && rear == -1); // Check if the queue is empty
}
void enqueue(int x) {
if (isFull()) {
cout << "Error: Queue is full" << endl; // Display error if the queue is full
return;
}
if (isEmpty()) {
front = 0;
rear = 0;
} else {
rear++;
}
arr[rear] = x; // Add an element to the rear of the queue
}
void dequeue() {
if (isEmpty()) {
cout << "Error: Queue is empty" << endl; // Display error if the queue is empty
return;
}
if (front == rear) {
front = -1;
rear = -1;
} else {
front++;
}
}
int peek() {
if (isEmpty()) {
cout << "Error: Queue is empty" << endl; // Display error if the queue is empty
return -1;
}
return arr[front]; // Return the element at the front of the queue
}
int find_Nth_From_Top(Queue q, int n) {
int size = 0;
Queue temp;
// Find the size of the queue
while (!q.isEmpty()) {
temp.enqueue(q.peek());
q.dequeue();
size++;
}
// If the given index is greater than the size of the queue
if (n > size) {
cout << "Error: Queue does not have " << n << " elements." << endl;
return -1;
}
// Copy the elements back to the original queue
for (int i = 0; i < size; i++) {
q.enqueue(temp.peek());
temp.dequeue();
}
// Dequeue the first (n-1) elements
for (int i = 0; i < n - 1; i++) {
q.enqueue(q.peek());
q.dequeue();
}
// Return the nth element from the top
return q.peek();
}
void display() {
if (isEmpty()) {
cout << "Error: Queue is empty" << endl; // Display error if the queue is empty
return;
}
cout << "Queue elements are: ";
for (int i = front; i <= rear; i++) {
cout << arr[i] << " "; // Display all elements in the queue
}
cout << endl;
}
};
int main() {
cout << "Initialize a Queue." << endl;
Queue q1; // Creating an instance of Queue
cout << "\nInsert some elements into the queue:" << endl;
q1.enqueue(0);
q1.enqueue(1);
q1.enqueue(2);
q1.enqueue(3);
q1.enqueue(4);
q1.enqueue(5);
q1.enqueue(6);
q1.display(); // Display elements of the queue
int n = 2;
cout << "\nNth element from top is " << q1.find_Nth_From_Top(q1, n) << " Where n = " << n << endl;
n = 3;
cout << "\nNth element from top is " << q1.find_Nth_From_Top(q1, n) << " Where n = " << n << endl;
n = 4;
cout << "\nNth element from top is " << q1.find_Nth_From_Top(q1, n) << " Where n = " << n << endl;
return 0;
}
Sample Output:
Initialize a Queue. Insert some elements into the queue: Queue elements are: 0 1 2 3 4 5 6 Nth element from top is 1 Where n = 2 Nth element from top is 2 Where n = 3 Nth element from top is 3 Where n = 4
Flowchart:
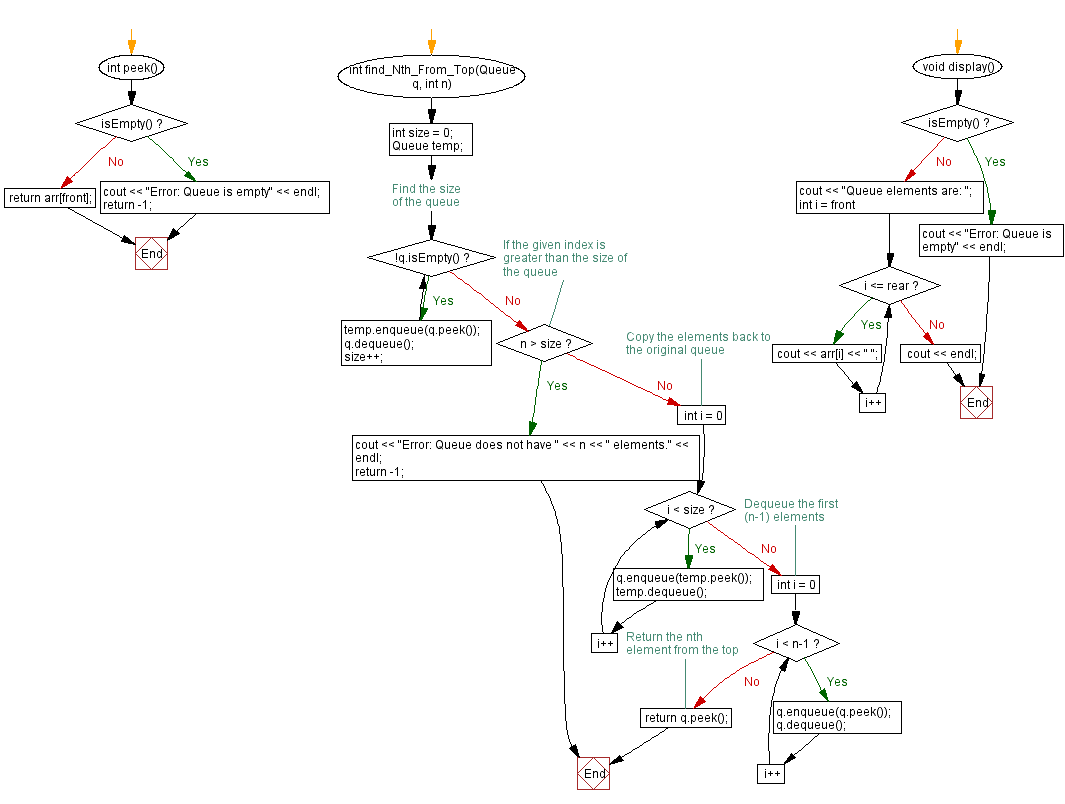
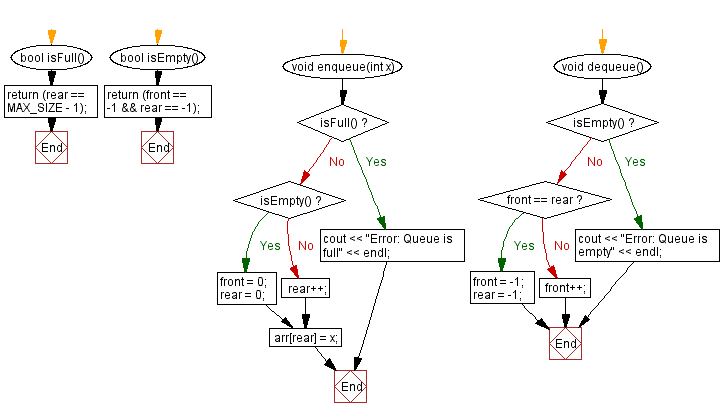
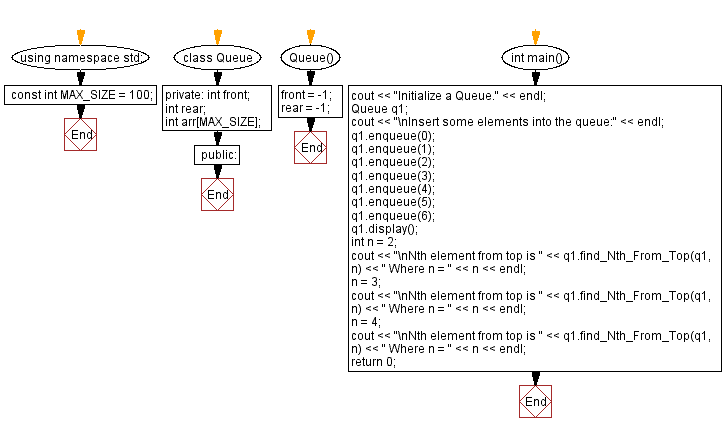
For more Practice: Solve these Related Problems:
- Write a C++ program to retrieve the Nth element from the front of a queue with appropriate bounds checking.
- Write a C++ program to access the Nth element from the top of a queue by transferring its elements to an array.
- Write a C++ program to implement a routine that prints the Nth element from the front of a queue without modification.
- Write a C++ program to fetch and display the Nth element from the head of a queue using iterative traversal.
CPP Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Nth element from the bottom of a queue.
Next C++ Exercise: Find the position of an element in a queue.
What is the difficulty level of this exercise?