C++ Queue Exercises: Remove all elements greater than a number from a queue
C++ Queue: Exercise-18 with Solution
Write a C++ program to remove all elements greater than a number from a queue.
Sample Solution:
C Code:
#include <iostream> // Including the necessary library for input and output operations
using namespace std;
const int MAX_SIZE = 100; // Maximum size for the queue
class Queue {
private:
int front; // Front of the queue
int rear; // Rear of the queue
int arr[MAX_SIZE]; // Array to store queue elements
public:
Queue() {
front = -1; // Initializing front index to -1
rear = -1; // Initializing rear index to -1
}
bool isFull() {
return (rear == MAX_SIZE - 1); // Check if the queue is full
}
bool isEmpty() {
return (front == -1 && rear == -1); // Check if the queue is empty
}
void enqueue(int x) {
if (isFull()) {
cout << "Error: Queue is full" << endl; // Display error if the queue is full
return;
}
if (isEmpty()) {
front = 0;
rear = 0;
}
else {
rear++;
}
arr[rear] = x; // Add an element to the rear of the queue
}
void dequeue() {
if (isEmpty()) {
cout << "Error: Queue is empty" << endl; // Display error if the queue is empty
return;
}
if (front == rear) {
front = -1;
rear = -1;
}
else {
front++;
}
}
int peek() {
if (isEmpty()) {
cout << "Error: Queue is empty" << endl; // Display error if the queue is empty
return -1;
}
return arr[front]; // Return the element at the front of the queue
}
void display() {
if (isEmpty()) {
cout << "Error: Queue is empty" << endl; // Display error if the queue is empty
return;
}
cout << "Queue elements are: ";
for (int i = front; i <= rear; i++) {
cout << arr[i] << " "; // Display all elements in the queue
}
cout << endl;
}
// Function to remove elements greater than a given limit
void remove_greater_than(Queue& q, int limit) {
if (q.isEmpty()) {
cout << "Error: Queue is empty" << endl; // Display error if the queue is empty
return;
}
int i = q.front;
while (i <= q.rear) {
if (q.arr[i] > limit) {
for (int j = i; j < q.rear; j++) {
q.arr[j] = q.arr[j + 1]; // Remove element by shifting subsequent elements
}
q.rear--; // Decrement the rear pointer
}
else {
i++;
}
}
}
};
int main() {
cout << "Initialize a Queue." << endl;
Queue q;
cout << "\nInsert some elements into the queue:" << endl;
q.enqueue(1);
q.enqueue(2);
q.enqueue(3);
q.enqueue(5);
q.enqueue(5);
q.enqueue(6);
q.enqueue(1);
q.display();
cout << "\nRemove all elements of the said queue greater than 3:" << endl;
q.remove_greater_than(q, 4); // Remove elements greater than 3
q.display();
return 0;
}
Sample Output:
Initialize a Queue. Insert some elements into the queue: Queue elements are: 1 2 3 5 5 6 1 Remove all elements of the said queue greater than 3: Queue elements are: 1 2 3 1
Flowchart:
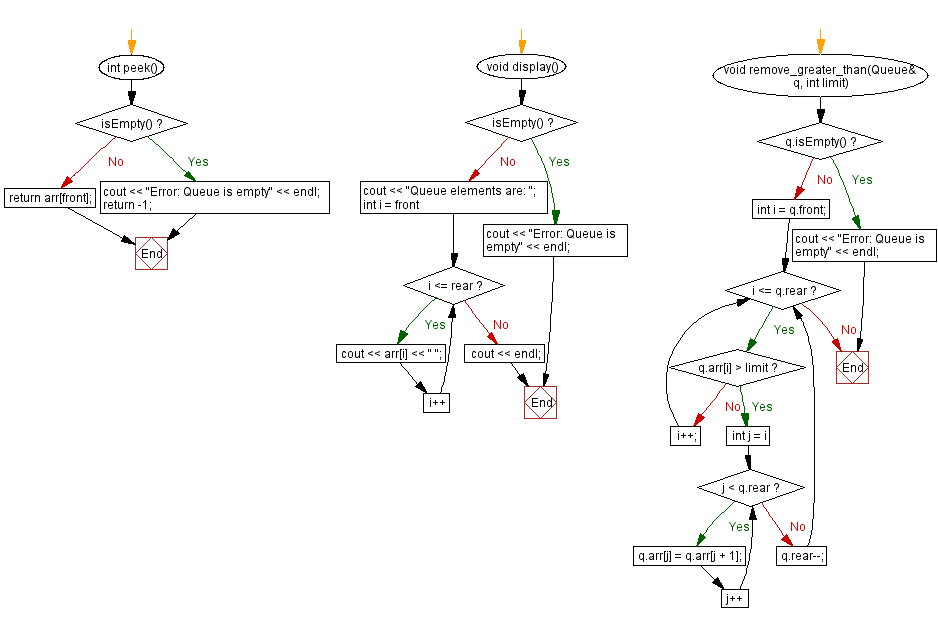
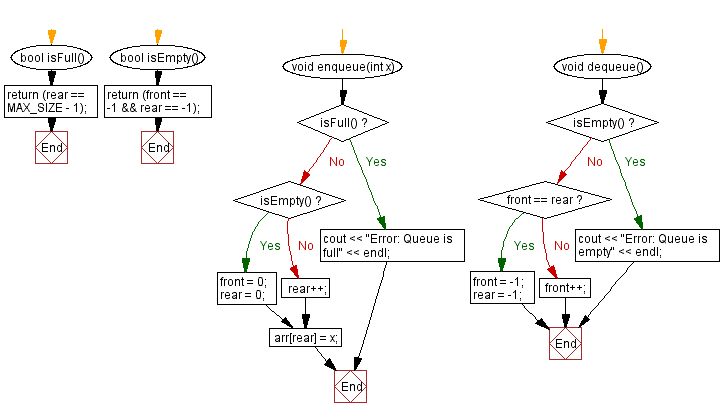
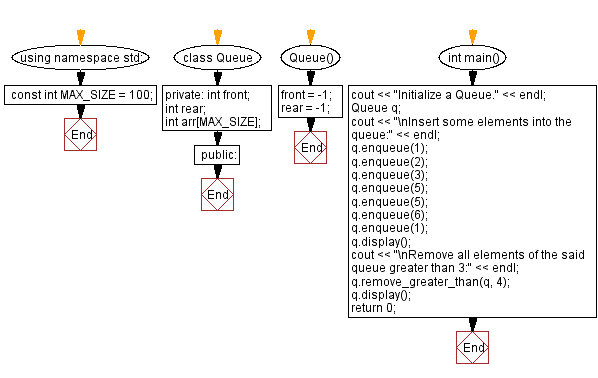
CPP Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Remove all duplicate elements from a queue.
Next C++ Exercise: Concatenate two queues.
What is the difficulty level of this exercise?
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/cpp-exercises/queue/cpp-queue-exercise-18.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics