C++ Queue Exercises: Remove all the elements from a queue
Write a C++ program to remove all the elements from a queue.
Sample Solution:
C Code:
#include <iostream>
#include <map>
#include <cmath>
using namespace std;
const int MAX_SIZE = 100;
class Queue {
private:
int front; // Holds the front index of the queue
int rear; // Holds the rear index of the queue
int arr[MAX_SIZE]; // Array to hold queue elements
public:
Queue() {
front = -1; // Initialize front index to -1
rear = -1; // Initialize rear index to -1
}
bool isFull() {
return (rear == MAX_SIZE - 1); // Checks if the queue is full
}
bool isEmpty() {
return (front == -1 && rear == -1); // Checks if the queue is empty
}
void enqueue(int x) {
if (isFull()) {
cout << "Error: Queue is full" << endl; // Displays error if the queue is full
return;
}
if (isEmpty()) {
front = 0;
rear = 0;
} else {
rear++;
}
arr[rear] = x; // Adds an element to the rear of the queue
}
void dequeue() {
if (isEmpty()) {
cout << "Error: Queue is empty" << endl; // Displays error if the queue is empty
return;
}
if (front == rear) {
front = -1;
rear = -1;
} else {
front++;
}
}
int peek() {
if (isEmpty()) {
cout << "Error: Queue is empty" << endl; // Displays error if the queue is empty
return -1;
}
return arr[front]; // Returns the element at the front of the queue
}
void display() {
if (isEmpty()) {
cout << "Error: Queue is empty" << endl; // Displays error if the queue is empty
return;
}
cout << "Queue elements are: ";
for (int i = front; i <= rear; i++) {
cout << arr[i] << " "; // Displays all elements in the queue
}
cout << endl;
}
void clear() {
while (!isEmpty()) {
dequeue(); // Removes all elements from the queue
}
}
};
int main() {
cout << "Initialize a Queue." << endl;
Queue q;
cout << "\nInsert some elements into the queue:" << endl;
q.enqueue(1);
q.enqueue(2);
q.enqueue(3);
q.enqueue(4);
q.enqueue(5);
q.enqueue(6);
q.display();
cout << "\nRemove all the elements from the said queue:" << endl;
q.clear();
q.display(); // Display an empty queue
cout << "\nInput two more elements into the queue:" << endl;
q.enqueue(1);
q.enqueue(2);
q.display();
cout << "\nRemove all the elements from the said queue:" << endl;
q.clear();
q.display(); // Display an empty queue again
return 0;
}
Sample Output:
Initialize a Queue. Insert some elements into the queue: Queue elements are: 1 2 3 4 5 6 Remove all the elements from the said queue: Error: Queue is empty Input two more elements into the queue: Queue elements are: 1 2 Remove all the elements from the said queue: Error: Queue is empty
Flowchart:
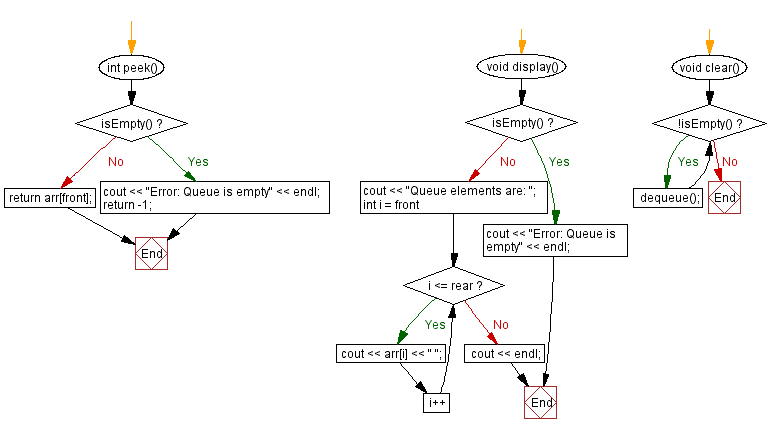
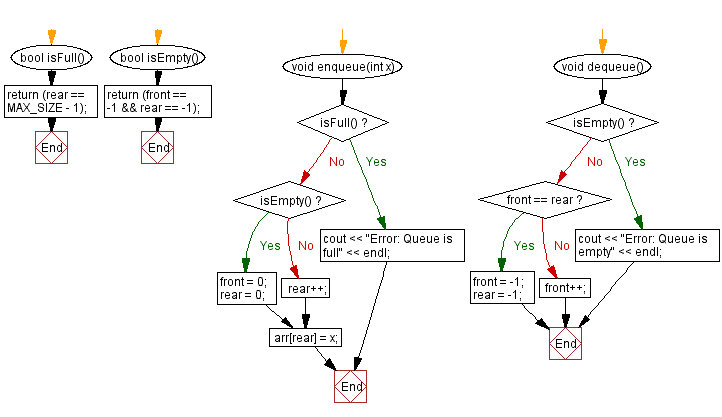
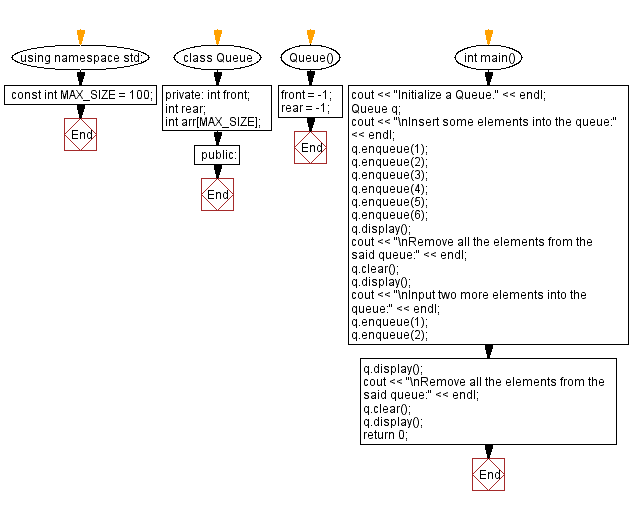
CPP Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Remove a given element from a queue.
Next C++ Exercise: Remove all even elements from a queue.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics