C++ Queue Exercises: Find the second lowest element of a queue
12. Second Lowest Element in a Queue
Write a C++ program to find the second lowest element of a queue.
Sample Solution:
C Code:
#include <iostream>
#include <map>
#include <cmath>
using namespace std;
const int MAX_SIZE = 100;
// Class representing a Queue data structure
class Queue {
private:
int front;
int rear;
int arr[MAX_SIZE];
public:
// Constructor initializing the Queue
Queue() {
front = -1;
rear = -1;
}
// Check if the Queue is full
bool isFull() {
return (rear == MAX_SIZE - 1);
}
// Check if the Queue is empty
bool isEmpty() {
return (front == -1 && rear == -1);
}
// Add an element to the Queue (enqueue)
void enqueue(int x) {
if (isFull()) {
cout << "Error: Queue is full" << endl;
return;
}
if (isEmpty()) {
front = 0;
rear = 0;
} else {
rear++;
}
arr[rear] = x;
}
// Remove an element from the Queue (dequeue)
void dequeue() {
if (isEmpty()) {
cout << "Error: Queue is empty" << endl;
return;
}
if (front == rear) {
front = -1;
rear = -1;
} else {
front++;
}
}
// Get the front element of the Queue
int peek() {
if (isEmpty()) {
cout << "Error: Queue is empty" << endl;
return -1;
}
return arr[front];
}
// Display all elements in the Queue
void display() {
if (isEmpty()) {
cout << "Error: Queue is empty" << endl;
return;
}
cout << "Queue elements are: ";
for (int i = front; i <= rear; i++) {
cout << arr[i] << " ";
}
cout << endl;
}
// Function to find the second lowest element in the Queue
int second_lowest(Queue q) {
int min1 = INT_MAX;
int min2 = INT_MAX;
while (!q.isEmpty()) {
int val = q.peek();
q.dequeue();
if (val < min1) {
min2 = min1;
min1 = val;
} else if (val < min2) {
min2 = val;
}
}
return min2;
}
};
// Main function
int main() {
cout << "Initialize a Queue." << endl;
Queue q;
cout << "\nInsert some elements into the queue:" << endl;
q.enqueue(1);
q.enqueue(2);
q.enqueue(3);
q.enqueue(4);
q.enqueue(5);
q.display();
int sh_el = q.second_lowest(q);
cout << "Second lowest element of the said queue: " << sh_el << endl;
cout << "\nInsert two more elements into the queue:" << endl;
q.enqueue(0);
q.enqueue(7);
q.display();
sh_el = q.second_lowest(q);
cout << "Second lowest element of the said queue: " << sh_el << endl;
return 0;
}
Sample Output:
Initialize a Queue. Insert some elements into the queue: Queue elements are: 1 2 3 4 5 Second lowest element of the said queue: 2 Insert two more elements into the queue: Queue elements are: 1 2 3 4 5 0 7 Second lowest element of the said queue: 1
Flowchart:
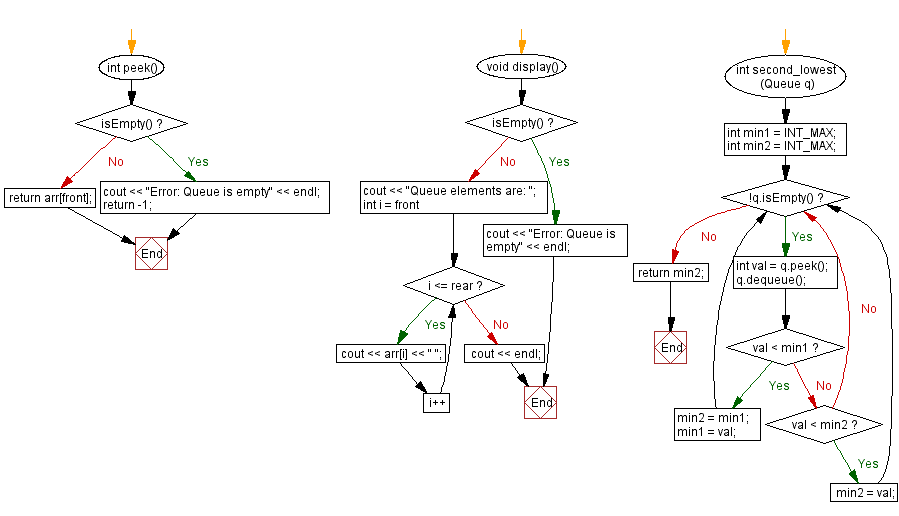
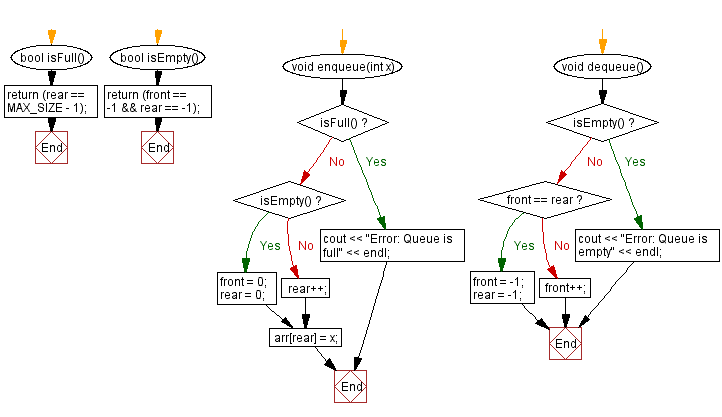
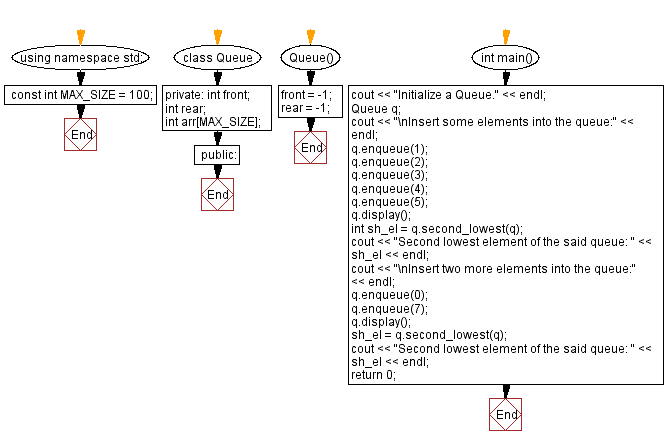
For more Practice: Solve these Related Problems:
- Write a C++ program to identify the second smallest element in a queue by tracking the two lowest values.
- Write a C++ program to compute the second lowest element in a queue while ensuring the first minimum is excluded.
- Write a C++ program to extract the two smallest elements from a queue and output the second lowest.
- Write a C++ program to find the second lowest value in a queue using an efficient one-pass algorithm.
CPP Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Find the second highest element of a queue.
Next C++ Exercise: Remove a given element from a queue.
What is the difficulty level of this exercise?