C++ Object-Oriented Programming: Student information and grade calculation
Write a C++ program to implement a class called Student that has private member variables for name, class, roll number, and marks. Include member functions to calculate the grade based on the marks and display the student's information.
Sample Solution:
C Code:
#include <iostream> // Include necessary header for input/output stream
#include <string> // Include necessary header for string handling
class Student { // Define a class named Student
private:
std::string name; // Private member variable to store student's name
std::string studentClass; // Private member variable to store student's class
int rollNumber; // Private member variable to store student's roll number
double marks; // Private member variable to store student's marks
public:
// Constructor to initialize Student object with provided details
Student(const std::string & studentName, const std::string & sClass, int rollNum, double studentMarks)
: name(studentName), studentClass(sClass), rollNumber(rollNum), marks(studentMarks) {}
// Member function to calculate the grade based on marks
std::string calculateGrade() {
if (marks >= 90) {
return "A+";
} else if (marks >= 80) {
return "A";
} else if (marks >= 70) {
return "B";
} else if (marks >= 60) {
return "C";
} else {
return "D";
}
}
// Member function to display student information
void displayInformation() {
std::cout << "\n\nName: " << name << std::endl; // Output student's name
std::cout << "Class: " << studentClass << std::endl; // Output student's class
std::cout << "Roll Number: " << rollNumber << std::endl; // Output student's roll number
std::cout << "Marks: " << marks << std::endl; // Output student's marks
std::cout << "Grade: " << calculateGrade() << std::endl; // Output student's grade
}
};
int main() {
// Create a student object
std::string studentName; // Variable to store student's name
std::string sClass; // Variable to store student's class
int rollNum; // Variable to store student's roll number
double studentMarks; // Variable to store student's marks
std::cout << "Student details: "; // Prompt user for student details
std::cout << "\nName: "; // Prompt user for student's name
std::getline(std::cin, studentName); // Input student's name
std::cout << "Class: "; // Prompt user for student's class
std::getline(std::cin, sClass); // Input student's class
std::cout << "Roll number: "; // Prompt user for student's roll number
std::cin >> rollNum; // Input student's roll number
std::cout << "Marks (0-100): "; // Prompt user for student's marks
std::cin >> studentMarks; // Input student's marks
Student student(studentName, sClass, rollNum, studentMarks); // Create Student object with provided details
// Display student information
student.displayInformation(); // Output student's information
return 0; // Return 0 to indicate successful completion
}
Sample Output:
Student details: Name: Ljubinka Marquita Class: V Roll number: 2 Marks (0-100): 98 Name: Ljubinka Marquita Class: V Roll Number: 2 Marks: 98 Grade: A+
Student details: Name: Lia Chimezie Class: VI Roll number: 8 Marks (0-100): 88 Name: Lia Chimezie Class: VI Roll Number: 8 Marks: 88 Grade: A
Explanation:
In the above exercise,
- The Student class is defined with private member variables name, studentClass, rollNumber, and marks representing the student's name, class, roll number, and marks.
- The constructor Student initializes the name, studentClass, rollNumber, and marks member variables.
- The member function calculateGrade calculates the grade based on the marks. It checks the marks against different ranges and returns the corresponding grade character.
- The member function displayInformation displays the student's information, including name, class, roll number, marks, and grade.
- In the main function, the user inputs the student's name, class, roll number, and marks.
- An instance of the Student class is created using the provided values.
- The displayInformation member function is called on the student object to display the student's information, including the calculated grade.
Flowchart:
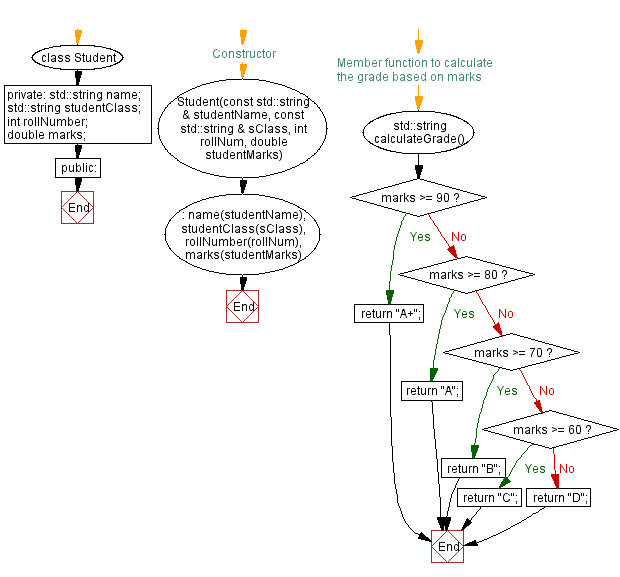
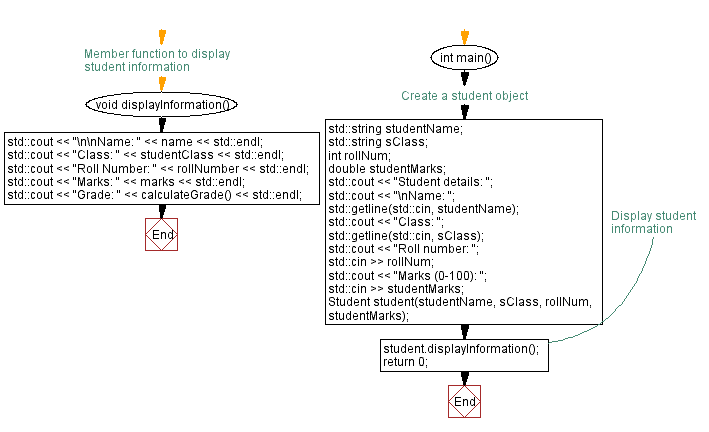
CPP Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Date validation and management.
Next C++ Exercise: Shape class and derived shapes.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics