C++ Object-Oriented Programming: Employee salary calculation
C++ Object oriented programming: Exercise-7 with Solution
Write a C++ program to implement a class called Employee that has private member variables for name, employee ID, and salary. Include member functions to calculate and set salary based on employee performance.
Sample Solution:
C Code:
#include <iostream> // Include necessary header for input/output stream
#include <string> // Include necessary header for handling strings
class Employee { // Define a class named Employee
private:
std::string name; // Private member variable to store employee name
int employeeId; // Private member variable to store employee ID
double salary; // Private member variable to store employee salary
public:
// Constructor to initialize Employee object with provided details
Employee(const std::string & empName, int empId, double empSalary): name(empName), employeeId(empId), salary(empSalary) {}
// Member function to calculate salary based on performance rating
void calculateSalary(double performanceRating) {
if (performanceRating >= 0.0 && performanceRating <= 1.4) { // Check if performance rating is within valid range
salary *= performanceRating; // Calculate updated salary based on performance rating
} else {
std::cout << "Invalid performance rating. Salary remains unchanged." << std::endl; // Output for an invalid performance rating
}
}
// Member function to set salary
void setSalary(double empSalary) {
salary = empSalary; // Set employee salary
}
// Member function to get employee name
std::string getName() const {
return name; // Return employee name
}
// Member function to get employee ID
int getEmployeeId() const {
return employeeId; // Return employee ID
}
// Member function to get employee salary
double getSalary() const {
return salary; // Return employee salary
}
};
int main() {
// Create an employee object
std::string empName; // Define variable to store employee name
int empId; // Define variable to store employee ID
double empSalary; // Define variable to store employee salary
std::cout << "Input employee name: "; // Prompt user to input employee name
std::cin >> empName; // Input for employee name
std::cout << "Input employee ID: "; // Prompt user to input employee ID
std::cin >> empId; // Input for employee ID
std::cout << "Input employee salary: "; // Prompt user to input employee salary
std::cin >> empSalary; // Input for employee salary
Employee employee(empName, empId, empSalary); // Create Employee object with provided details
// Calculate and display initial salary
std::cout << "Initial salary: " << employee.getSalary() << std::endl; // Output initial salary
// Perform salary calculation based on performance rating
double performanceRating; // Define variable to store performance rating
std::cout << "Input performance rating (0.0-1.2): "; // Prompt user to input performance rating
std::cin >> performanceRating; // Input for performance rating
employee.calculateSalary(performanceRating); // Calculate updated salary based on performance
// Display updated salary
std::cout << "Updated salary: " << employee.getSalary() << std::endl; // Output updated salary
return 0; // Return 0 to indicate successful completion
}
Sample Output:
Input employee name: Florizan Input employee ID: 12 Input employee salary: 1500 Initial salary: 1500 Input performance rating (0.0-1.2): 1.2 Updated salary: 1800
Input employee name: Hizkiah Input employee ID: 222 Input employee salary: 1200 Initial salary: 1200 Input performance rating (0.0-1.2): .9 Updated salary: 1080
Explanation:
In the above exercise,
- The Employee class is defined with private member variables name, employeeId, and salary representing the employee's name, ID, and salary.
- The constructor Employee initializes the name, employeeId, and salary member variables.
- The member function calculateSalary calculates the salary based on the provided performanceRating parameter. If the performance rating is within the valid range of 0.0 to 1.4, the salary is multiplied by the performance rating. Otherwise, an error message is displayed.
- The member function setSalary sets the salary to the provided value.
- The member functions getName, getEmployeeId, and getSalary are implemented to retrieve the values of the respective member variables.
- In the main function, the user enters the employee's name, ID, and initial salary.
- An instance of the Employee class is created using the provided values.
- The initial salary is displayed.
- The user is prompted to input a performance rating (a value between 0.0 and 1.4).
- The calculateSalary member function is called on the employee object with the provided performance rating to calculate the updated salary.
- The updated salary is displayed.
Flowchart:
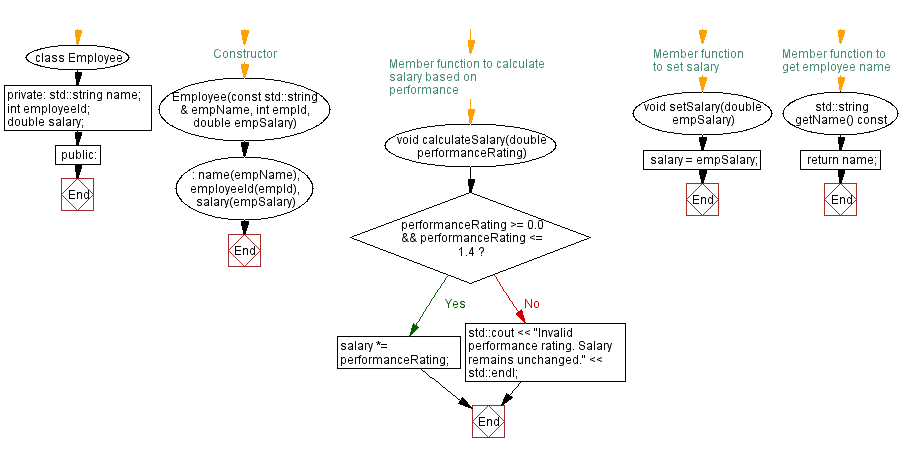
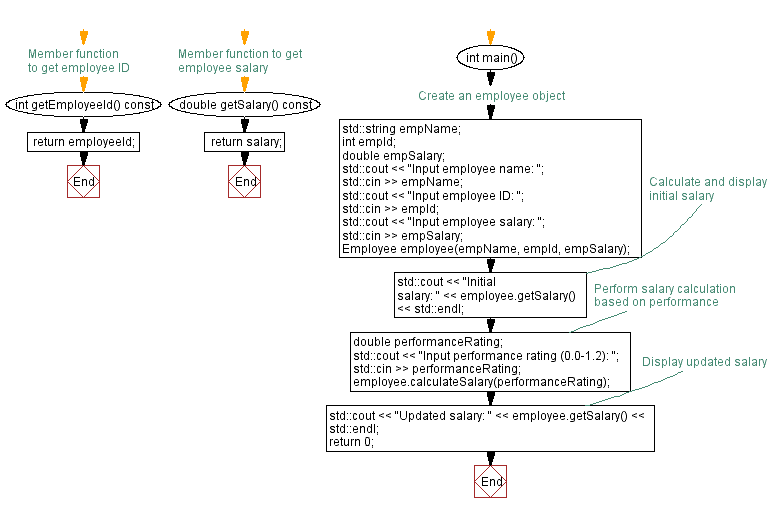
CPP Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Triangle classification.
Next C++ Exercise: Date validation and management.
What is the difficulty level of this exercise?
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/cpp-exercises/oop/cpp-oop-exercise-7.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics