C++ Object-Oriented Programming: Triangle classification
6. Triangle Class with Side Lengths and Type Determination
An equilateral triangle is a triangle in which all three sides are equal.
A scalene triangle is a triangle that has three unequal sides.
An isosceles triangle is a triangle with (at least) two equal sides.
Visual Presentation:
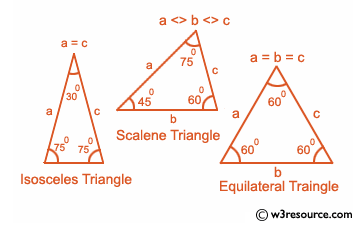
Write a C++ program to create a class called Triangle that has private member variables for the lengths of its three sides. Implement member functions to determine if the triangle is equilateral, isosceles, or scalene.
Sample Solution:
C Code:
#include <iostream> // Include necessary header for input/output stream
class Triangle { // Define a class named Triangle
private:
double side1; // Private member variable to store side1 of the triangle
double side2; // Private member variable to store side2 of the triangle
double side3; // Private member variable to store side3 of the triangle
public:
// Constructor to initialize Triangle object with provided side lengths
Triangle(double s1, double s2, double s3): side1(s1), side2(s2), side3(s3) {}
// Member function to determine the type of the triangle
void determineType() {
if (side1 == side2 && side2 == side3) { // Check if all sides are equal
std::cout << "Equilateral Triangle" << std::endl; // Output if all sides are equal
} else if (side1 == side2 || side1 == side3 || side2 == side3) { // Check if at least two sides are equal
std::cout << "Isosceles Triangle" << std::endl; // Output if at least two sides are equal
} else {
std::cout << "Scalene Triangle" << std::endl; // Output if no sides are equal
}
}
};
int main() {
// Create a triangle object
double s1, s2, s3; // Define variables for side lengths
std::cout << "Input the lengths of the three sides of the triangle:\n"; // Prompt user to input side lengths
std::cout << "Side1: ";
std::cin >> s1; // Input for side1
std::cout << "Side2: ";
std::cin >> s2; // Input for side2
std::cout << "Side3: ";
std::cin >> s3; // Input for side3
Triangle triangle(s1, s2, s3); // Create Triangle object with provided side lengths
// Determine the type of triangle and output the result
triangle.determineType(); // Call determineType method to identify the triangle type
return 0; // Return 0 to indicate successful completion
}
Sample Output:
Input the lengths of the three sides of the triangle: Side1: 3 Side2: 3 Side3: 3 Equilateral Triangle
Input the lengths of the three sides of the triangle: Side1: 3 Side2: 4 Side3: 5 Scalene Triangle
Input the lengths of the three sides of the triangle: Side1: 5 Side2: 5 Side3: 7 Isosceles Triangle
Explanation:
In the above exercise,
- The Triangle class is defined with private member variables side1, side2, and side3 representing the lengths of the triangle's sides.
- The constructor Triangle initializes the side1, side2, and side3 member variables.
- The member function determineType determines the type of triangle based on its side lengths. It checks if all three sides are equal to classify it as an equilateral triangle. If two sides are equal, it is an isosceles triangle. Otherwise, it is considered a scalene triangle. The appropriate message is displayed based on classification.
Flowchart:
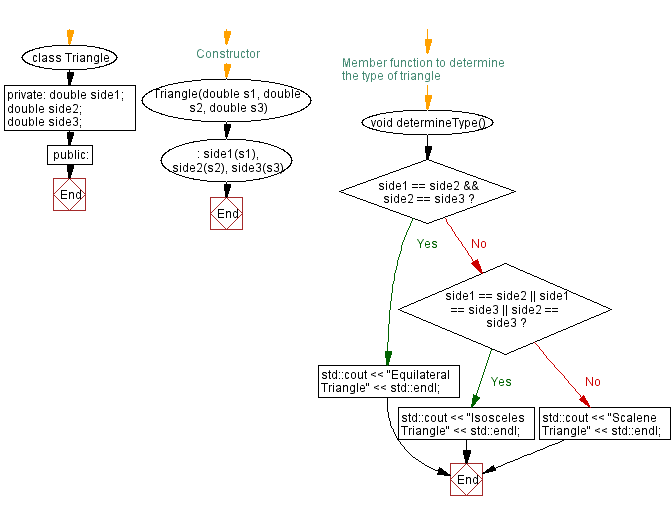
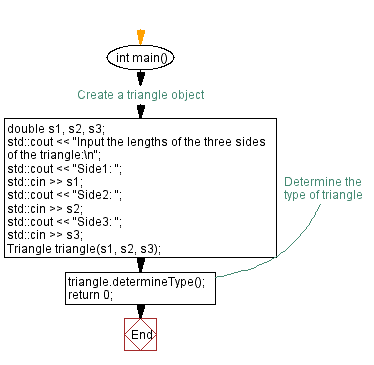
For more Practice: Solve these Related Problems:
- Write a C++ program to implement a Triangle class that calculates whether a triangle is equilateral, isosceles, or scalene based on its side lengths.
- Write a C++ program to create a Triangle class that validates if three sides can form a triangle and determines its type, including a check for right triangles.
- Write a C++ program to implement a Triangle class with private side lengths and member functions to determine the triangle type and calculate its area using Heron’s formula.
- Write a C++ program to design a Triangle class that includes methods for setting sides, checking validity, and determining if it is acute, obtuse, or right-angled.
CPP Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Bank Account Class.
Next C++ Exercise: Employee salary calculation.
What is the difficulty level of this exercise?