C++ Object-Oriented Programming: Bank Account Class
5. BankAccount Class with Deposit and Withdraw
Write a C++ program to implement a class called BankAccount that has private member variables for account number and balance. Include member functions to deposit and withdraw money from the account.
Sample Solution:
C Code:
#include <iostream> // Include the necessary header for input/output stream
#include <string> // Include the necessary header for string operations
class BankAccount { // Define a class named BankAccount
private:
std::string accountNumber; // Private member variable to store the account number
double balance; // Private member variable to store the balance
public:
// Constructor to initialize BankAccount object with provided values
BankAccount(const std::string & accNum, double initialBalance): accountNumber(accNum), balance(initialBalance) {}
// Member function to deposit money into the account
void deposit(double amount) {
balance += amount; // Add the deposited amount to the current balance
std::cout << "Deposit successful. Current balance: " << balance << std::endl; // Output success message and current balance
}
// Member function to withdraw money from the account
void withdraw(double amount) {
if (amount <= balance) { // Check if the withdrawal amount is less than or equal to the current balance
balance -= amount; // Deduct the withdrawn amount from the current balance
std::cout << "Withdrawal successful. Current balance: " << balance << std::endl; // Output success message and current balance
} else {
std::cout << "Insufficient balance. Cannot withdraw." << std::endl; // Output error message for insufficient balance
}
}
};
int main() {
// Create a bank account object
std::string sacno = "SB-123"; // Define the account number
double Opening_balance, deposit_amt, withdrawal_amt; // Define variables for opening balance, deposit amount, and withdrawal amount
Opening_balance = 1000; // Assign the opening balance
std::cout << "A/c. No." << sacno << " Balance: " << Opening_balance << std::endl; // Output the account details
BankAccount account(sacno, 1000.0); // Create a BankAccount object with initial account number and balance
// Deposit money into the account
deposit_amt = 1500; // Define the deposit amount
std::cout << "Deposit Amount: " << deposit_amt << std::endl; // Output the deposit amount
account.deposit(deposit_amt); // Call the deposit method of the account object
// Withdraw money from the account
withdrawal_amt = 750; // Define the withdrawal amount
std::cout << "Withdrawal Amount: " << withdrawal_amt << std::endl; // Output the withdrawal amount
account.withdraw(withdrawal_amt); // Call the withdraw method of the account object
// Attempt to withdraw more money than the balance
withdrawal_amt = 1800; // Define an amount higher than the balance for withdrawal
std::cout << "Attempt to withdrawal Amount: " << withdrawal_amt << std::endl; // Output the withdrawal amount
account.withdraw(withdrawal_amt); // Call the withdraw method of the account object
return 0; // Return 0 to indicate successful completion
}
Sample Output:
A/c. No.SB-123 Balance: 1000 Deposit Amount: 1500 Deposit successful. Current balance: 2500 Withdrawl Amount: 750 Withdrawal successful. Current balance: 1750 Attempt to withdrawl Amount: 1800 Insufficient balance. Cannot withdraw.
Explanation:
In the above exercise,
- The BankAccount class represents a bank account with private member variables accountNumber and balance.
- The constructor initializes the accountNumber and balance member variables.
- The deposit() member function takes a parameter amount and adds it to the current balance. It then displays the updated balance.
- The withdraw() member function takes a parameter amount and checks if it is less than or equal to the current balance. If so, it subtracts the amount from the balance and displays the updated balance. If the amount is greater than the balance, it displays an insufficient balance message.
Flowchart:
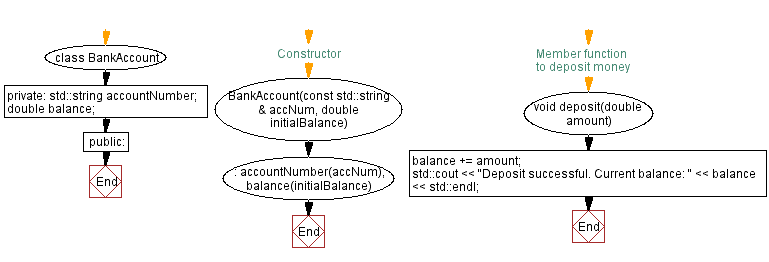
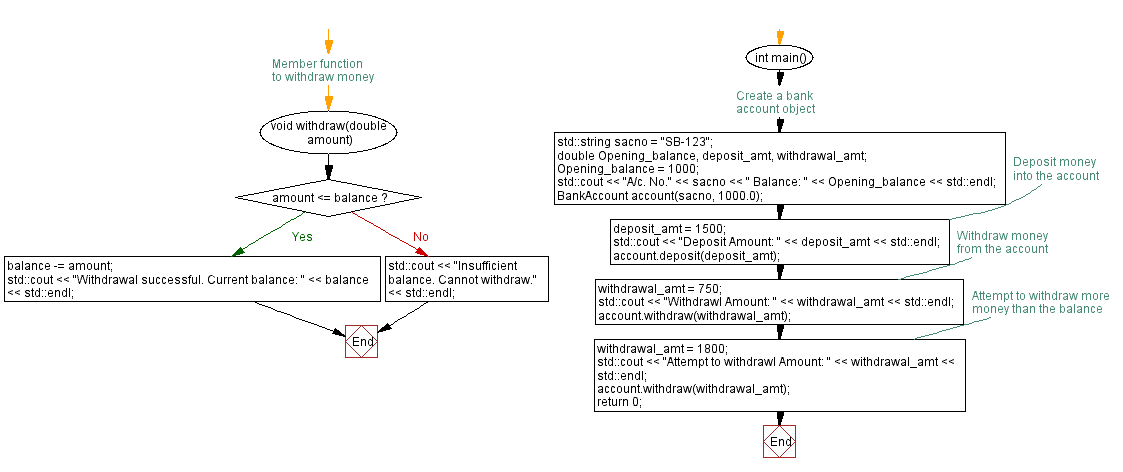
For more Practice: Solve these Related Problems:
- Write a C++ program to implement a BankAccount class that supports deposit, withdrawal, and balance inquiry operations with proper error handling.
- Write a C++ program to create a BankAccount class with private members for account number and balance, and overload the += operator for deposit operations.
- Write a C++ program to design a BankAccount class that includes member functions to perform deposit and withdrawal operations, and a function to display the account summary.
- Write a C++ program to implement a BankAccount class that maintains a transaction log and allows dynamic updating of the balance after each operation.
CPP Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Car class.
Next C++ Exercise: Triangle classification.
What is the difficulty level of this exercise?