C++ Object-Oriented Programming: Rectangle class
Write a C++ program to create a class called Rectangle that has private member variables for length and width. Implement member functions to calculate the rectangle's area and perimeter.
Sample Solution:
C Code:
#include <iostream> // Include necessary header for input/output stream
class Rectangle { // Define a class named Rectangle
private:
double length; // Private member to store the length of the rectangle
double width; // Private member to store the width of the rectangle
public:
// Constructor to initialize the Rectangle object with length and width
Rectangle(double len, double wid): length(len), width(wid) {}
// Member function to calculate the area of the rectangle
double calculateArea() {
return length * width; // Formula to calculate the area of a rectangle
}
// Member function to calculate the perimeter of the rectangle
double calculatePerimeter() {
return 2 * (length + width); // Formula to calculate the perimeter of a rectangle
}
};
int main() {
double length, width;
std::cout << "Input the length of the rectangle: ";
std::cin >> length; // Input the length of the rectangle from the user
std::cout << "Input the width of the rectangle: ";
std::cin >> width; // Input the width of the rectangle from the user
// Create a rectangle object with the provided length and width
Rectangle rectangle(length, width);
// Calculate and display the area of the rectangle
double area = rectangle.calculateArea(); // Calculate the area using the Rectangle object
std::cout << "\nArea: " << area << std::endl; // Output the calculated area
// Calculate and display the perimeter of the rectangle
double perimeter = rectangle.calculatePerimeter(); // Calculate the perimeter using the Rectangle object
std::cout << "Perimeter: " << perimeter << std::endl; // Output the calculated perimeter
return 0; // Return 0 to indicate successful completion
}
Sample Output:
Input the length of the rectangler: 4.0 Input the width of the rectangle: 7.2 Area: 28.8 Perimeter: 22.4
Explanation:
In the above exercise
The Rectangle class represents a rectangle with private member variables length and width. The constructor initializes the rectangle's length and width.
The calculateArea() member function calculates the rectangle area by multiplying its length and width. It returns the calculated area.
The calculatePerimeter() member function calculates the perimeter of the rectangle by adding the lengths of all four sides (2 * (length + width)). It returns the calculated perimeter.
In the main() function, a Rectangle object is created with a length and width taken from the user. The calculateArea() and calculatePerimeter() functions are then called on this object to compute and display the area and perimeter of the rectangle.
Flowchart:
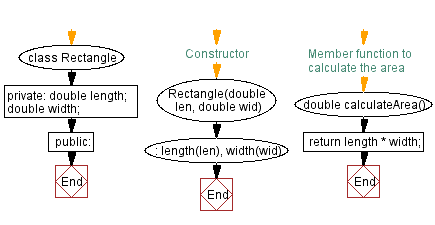
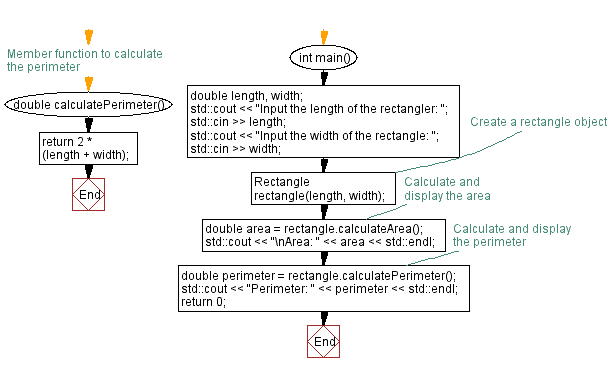
CPP Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Circle class.
Next C++ Exercise: Person class.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics