C++ Object-Oriented Programming: Circle class
Write a C++ program to implement a class called Circle that has private member variables for radius. Include member functions to calculate the circle's area and circumference.
Sample Solution:
C Code:
#include <iostream> // Include necessary header for input/output stream
#include <cmath> // Include necessary header for mathematical functions
const double PI = 3.14159; // Define the value of PI as a constant
class Circle { // Define a class named Circle
private:
double radius; // Private member to store the radius
public:
// Constructor to initialize the Circle object with a radius
Circle(double rad): radius(rad) {}
// Member function to calculate the area of the circle
double calculateArea() {
return PI * pow(radius, 2); // Formula to calculate the area of a circle
}
// Member function to calculate the circumference of the circle
double calculateCircumference() {
return 2 * PI * radius; // Formula to calculate the circumference of a circle
}
};
int main() {
// Create a circle object
double radius;
std::cout << "Input the radius of the circle: ";
std::cin >> radius; // Input the radius from the user
Circle circle(radius); // Create a Circle object with the given radius
// Calculate and display the area of the circle
double area = circle.calculateArea(); // Calculate the area using the Circle object
std::cout << "Area: " << area << std::endl; // Output the calculated area
// Calculate and display the circumference of the circle
double circumference = circle.calculateCircumference(); // Calculate the circumference using the Circle object
std::cout << "Circumference: " << circumference << std::endl; // Output the calculated circumference
return 0; // Return 0 to indicate successful completion
}
Sample Output:
Input the radius of the circle: 5.2 Area: 84.9486 Circumference: 32.6725
Input the radius of the circle: 4 Area: 50.2654 Circumference: 25.1327
Explanation:
In the above exercise,
- const double PI = 3.14159;: This code defines a constant variable PI and assigns it the value of pi (approximately 3.14159).
- class Circle { ... }: This block defines a class named Circle. It has a private member variable radius to store the circle radius.
- Circle(double rad) : radius(rad) { }: This is the constructor of the Circle class. It takes a parameter rad and initializes the radius member variable with the given value.
- double calculateArea() { ... }: This member function calculates the circle area using the formula PI * radius^2. It returns the calculated area.
- double calculateCircumference() { ... }: This member function calculates the circumference of the circle using the formula 2 * PI * radius. It returns the calculated circumference.
- In the main() function, the program prompts the user to input the circle radius using std::cout and std::cin. It creates a Circle object by calling the constructor with the input radius.
- It then calls the calculateArea() and calculateCircumference() member functions on the Circle object to compute the area and circumference.
Flowchart:
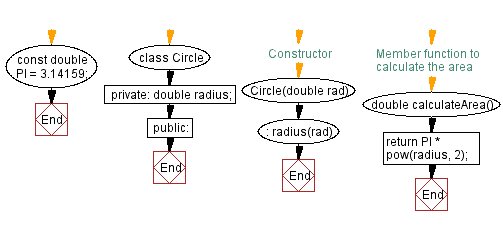
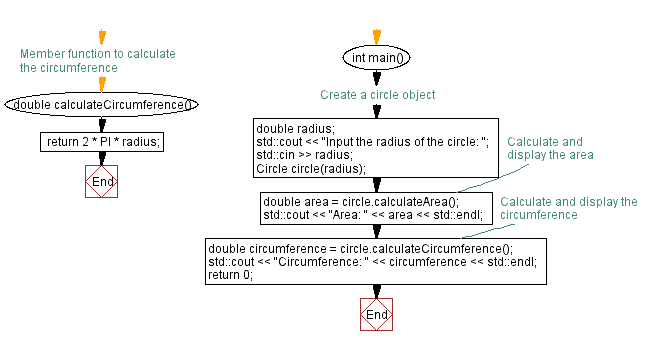
CPP Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: C++ Object oriented programming Exercises Home.
Next C++ Exercise: Rectangle class.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics