C++ Exercises: Check whether a given number is a Kaprekar number or not
9. Kaprekar Number Check
Write a program in C++ to check whether a given number is a Kaprekar number or not.
Visual Presentation:
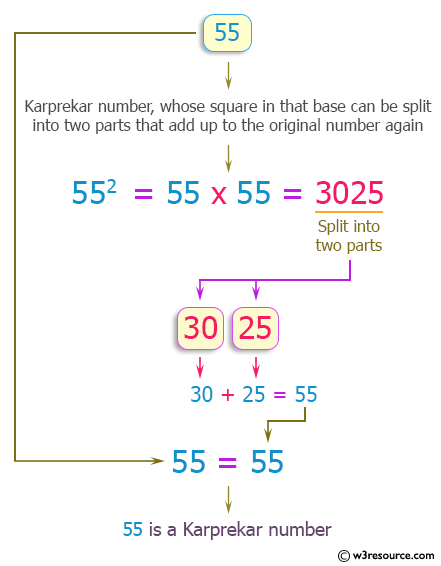
Sample Solution:
C++ Code :
#include<bits/stdc++.h> // Include the entire standard C++ library
using namespace std;
bool chkkaprekar(int n)
{
if (n == 1)
return true; // If the number is 1, it's considered a Kaprekar number
int sqr_n = n * n; // Calculate the square of the number
int ctr_digits = 0; // Variable to count the number of digits in the square
// Calculate the number of digits in the square of n
while (sqr_n)
{
ctr_digits++; // Increment the digit count
sqr_n /= 10; // Reduce the number by one digit
}
sqr_n = n * n; // Reset sqr_n to n squared
for (int r_digits = 1; r_digits < ctr_digits; r_digits++)
{
int eq_parts = pow(10, r_digits); // Determine the equal parts for the comparison
if (eq_parts == n)
continue; // If equal parts match the number, skip the comparison
// Calculate the sum of two parts after splitting the square number
int sum = sqr_n / eq_parts + sqr_n % eq_parts;
if (sum == n)
return true; // If the sum matches the original number, it's a Kaprekar number
}
return false; // If no condition satisfies, it's not a Kaprekar number
}
int main()
{
int kpno; // Declare a variable for the input number
cout << "\n\n Check whether a given number is a Kaprekar number: \n"; // Display a message for user
cout << " -------------------------------------------------------\n";
cout << " Input a number: "; // Prompt the user to input a number
cin >> kpno; // Read the input number
// Check if the entered number is a Kaprekar number and display the result
chkkaprekar(kpno) ? cout << kpno << " is a Kaprekar number. " : cout << kpno << " is not a Kaprekar number.";
cout << endl;
}
Sample Output:
Check whether a given number is a Kaprekar number: ------------------------------------------------------- Input a number: 45. 45 is a Kaprekar number.
Flowchart:
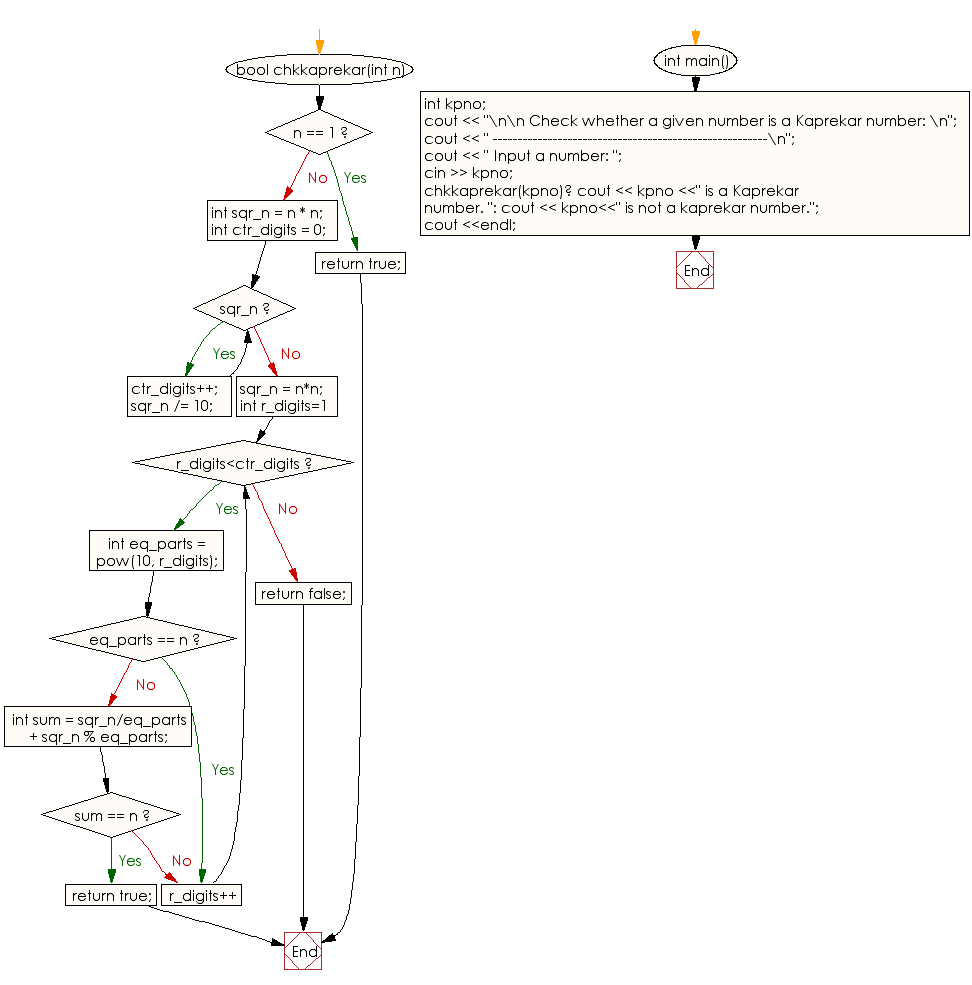
For more Practice: Solve these Related Problems:
- Write a C++ program to determine if a number is a Kaprekar number by splitting its square into two parts.
- Write a C++ program to verify Kaprekar numbers using string manipulation to split the squared value.
- Write a C++ program to check for Kaprekar numbers with dynamic memory allocation for handling large numbers.
- Write a C++ program to test if a number is Kaprekar by implementing custom functions for digit extraction and summation.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to generate random integers in a specific range.
Next: Write a program in C++ to generate and show all Kaprekar numbers less than 1000.
What is the difficulty level of this exercise?