C++ Exercises: Generate random integers in a specific range
Write a program in C++ to generate random integers in a specific range.
Visual Presentation:
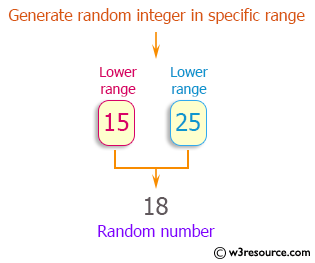
Sample Solution:
C++ Code :
#include <cstdlib> // Including the C Standard Library for functions like srand and rand
#include <ctime> // Including the C Time Library for time related functions
#include <iostream> // Including the standard input/output stream library
using namespace std;
int main()
{
int ln, un; // Declare variables for lower and upper range of numbers
cout << "\n\n Generate random integer in a specific range: \n"; // Display a message to prompt the user
cout << " --------------------------------------------------\n";
cout << " Input the lower range of number: "; // Prompt the user to input the lower range
cin >> ln; // Read the input lower range from the user
cout << " Input the upper range of number: "; // Prompt the user to input the upper range
cin >> un; // Read the input upper range from the user
srand(time(NULL)); // Seed the random number generator using the current time
cout << " The random number between " << ln << " and " << un << " is: "; // Display the range of random numbers
cout << ln + rand() % static_cast<int>(un - ln + 1) << endl; // Generate and display a random number in the specified range
return 0; // Return 0 to indicate successful completion of the program
}
Sample Output:
Generate random integer in a specific range: -------------------------------------------------- Input the lower range of number: 15 Input the upper range of number: 25 The random number between 15 and 25 is: 18
Flowchart:
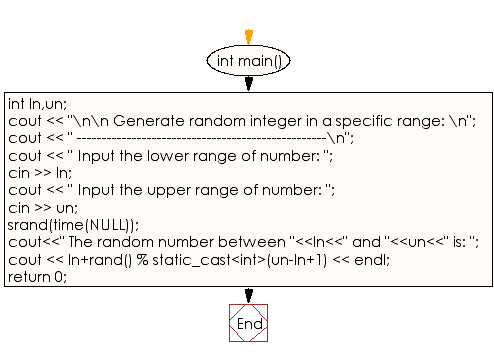
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to find the Deficient numbers (integers) between 1 to 100.
Next: Write a program in C++ to check whether a given number is a Kaprekar number or not.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics