C++ Exercises: Find the Deficient numbers between 1 to 100
7. Find Deficient Numbers Between 1 and 100
Write a program in C++ to find the Deficient numbers (integers) between 1 and 100.
Visual Presentation:
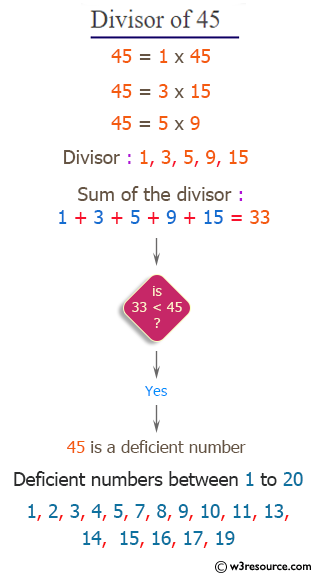
Sample Solution:
C++ Code :
#include <bits/stdc++.h> // Include all standard libraries
using namespace std;
// Function to calculate the sum of divisors of a number 'n'
int getSum(int n) {
int sum = 0;
for (int i = 1; i <= sqrt(n); i++) { // Loop to find divisors up to square root of 'n'
if (n % i == 0) { // Check if 'i' is a divisor of 'n'
if (n / i == i) // If 'i' is a square root divisor of 'n', add 'i' to sum
sum = sum + i;
else { // Otherwise, add both 'i' and 'n/i' to sum
sum = sum + i;
sum = sum + (n / i);
}
}
}
sum = sum - n; // Subtract 'n' to get the sum of proper divisors
return sum; // Return the sum of divisors
}
// Function to check if a number is deficient or not
bool checkDeficient(int n) {
return (getSum(n) < n); // Return true if the sum of divisors is less than 'n'
}
int main() {
int n, ctr = 0;
cout << "\n\n The Deficient numbers between 1 to 100 are: \n";
cout << " ------------------------------------------------\n";
for (int j = 1; j <= 100; j++) { // Loop through numbers from 1 to 100
n = j;
// Check if the number is deficient and display it
checkDeficient(n) ? cout << n << " " : cout << "";
if (checkDeficient(n)) {
ctr++; // Increment count if the number is deficient
}
}
cout << endl << "The Total number of Deficient numbers are: " << ctr << endl; // Display the total count of deficient numbers
}
Sample Output:
The Deficient numbers between 1 to 100 are: ------------------------------------------------ 1 2 3 4 5 7 8 9 10 11 13 14 15 16 17 19 21 22 23 25 26 27 29 31 32 33 3 4 35 37 38 39 41 43 44 45 46 47 49 50 51 52 53 55 57 58 59 61 62 63 64 65 67 68 69 71 73 74 75 76 77 79 81 82 83 85 86 87 89 91 92 93 94 95 97 98 99 The Total number of Deficient numbers are: 76
Flowchart:
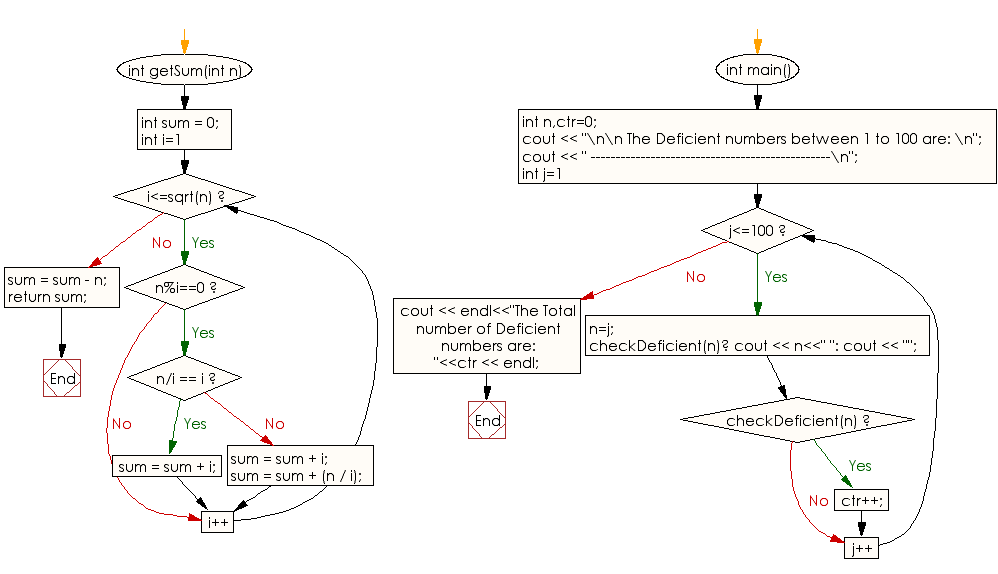
For more Practice: Solve these Related Problems:
- Write a C++ program to list all deficient numbers between 1 and 100 using an efficient sieve-like method.
- Write a C++ program to generate and display deficient numbers in a range by using recursion for divisor calculation.
- Write a C++ program to compute deficient numbers between 1 and 100 with a focus on optimized arithmetic operations.
- Write a C++ program to display deficient numbers and their counts using STL containers and algorithms.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to check whether a given number is Deficient or not.
Next: Write a program in C++ to generate random integers in a specific range.
What is the difficulty level of this exercise?