C++ Exercises: Check whether a given number is Deficient or not
6. Deficient Number Check
Write a program in C++ to check whether a given number is Deficient or not.
Visual Presentation:
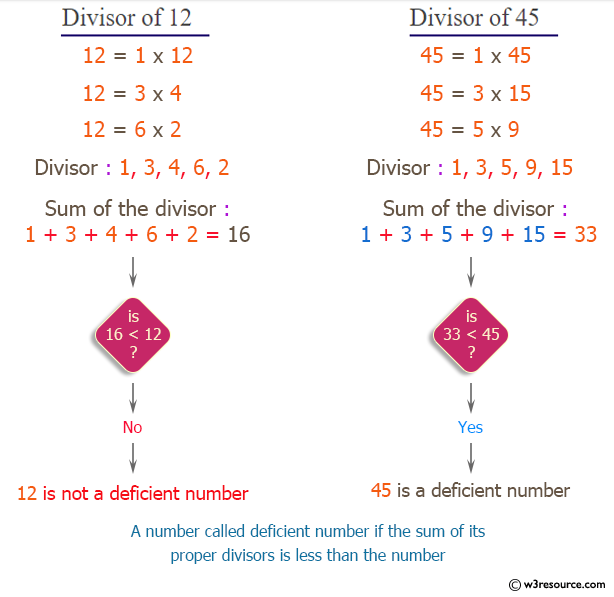
Sample Solution:
C++ Code :
#include <bits/stdc++.h> // Include all standard libraries
using namespace std;
// Function to calculate the sum of divisors of a number 'n'
int getSum(int n)
{
int sum = 0;
for (int i = 1; i <= sqrt(n); i++) // Loop to find divisors up to square root of 'n'
{
if (n % i == 0) // Check if 'i' is a divisor of 'n'
{
if (n / i == i) // If 'i' is a square root divisor of 'n', add 'i' to sum
sum = sum + i;
else // Otherwise, add both 'i' and 'n/i' to sum
{
sum = sum + i;
sum = sum + (n / i);
}
}
}
sum = sum - n; // Subtract 'n' to get the sum of proper divisors
return sum; // Return the sum of divisors
}
// Function to check if a number is deficient or not
bool checkDeficient(int n)
{
return (getSum(n) < n); // Return true if the sum of divisors is less than 'n'
}
int main()
{
int n;
cout << "\n\n Check whether a given number is a Deficient number:\n";
cout << " --------------------------------------------------------\n";
cout << " Input an integer number: ";
cin >> n; // Input the number
// Check if the number is deficient and display the result
checkDeficient(n) ? cout << " The number is Deficient.\n" : cout << " The number is not Deficient.\n";
return 0; // Return 0 to indicate successful completion
}
Sample Output:
Check whether a given number is an Deficient number: -------------------------------------------------------- Input an integer number: 25 The number is Deficient.
Flowchart:
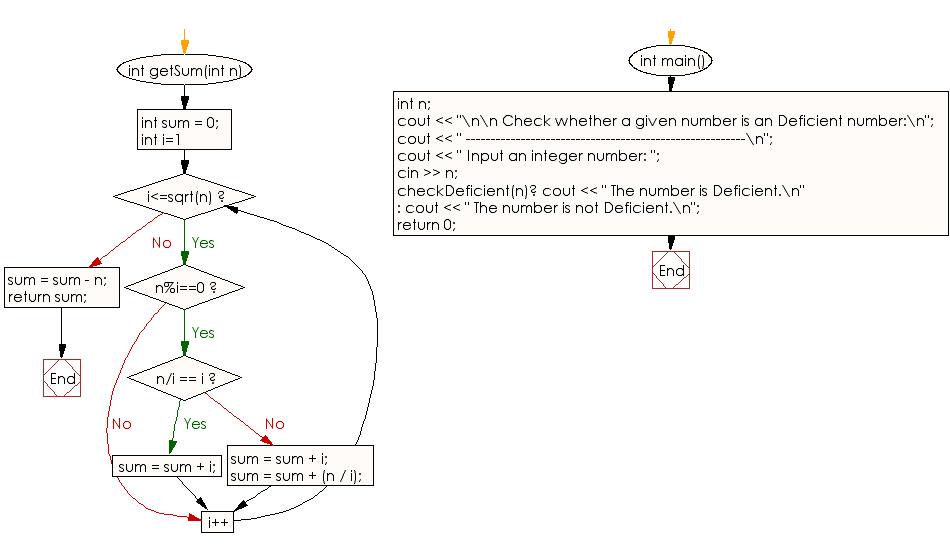
For more Practice: Solve these Related Problems:
- Write a C++ program to check if a number is deficient by comparing the sum of its proper divisors to itself.
- Write a C++ program to verify deficiency of a number using a recursive function to accumulate divisors.
- Write a C++ program to test if a number is deficient with inline functions and a ternary operator for output.
- Write a C++ program to determine deficiency by processing divisor sums in a single pass through the number’s factors.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to find Perfect numbers and number of Perfect numbers between 1 to 1000.
Next: Write a program in C++ to find the Deficient numbers (integers) between 1 to 100.
What is the difficulty level of this exercise?