C++ Exercises: Find Strong Numbers within a range of numbers
40. Find Strong Numbers Within a Range
Write a C++ program to find Strong Numbers within a range of numbers.
Sample Solution:
C++ Code:
#include <iostream>
using namespace std;
int main()
{
int i, n, n1, s1 = 0, j, k, en, sn; // Declare variables for iteration and range of numbers
long fact; // Declare a variable to store factorial
// Prompt the user to input the range of numbers to find Strong numbers
cout << "\n\n Find Strong Numbers within a range of numbers:\n";
cout << "----------------------------------------------------\n";
cout << " Input the starting range of numbers: ";
cin >> sn; // Input starting range
cout << " Input the ending range of numbers: ";
cin >> en; // Input ending range
cout << " The Strong numbers within the range are: ";
// Loop through the numbers within the specified range
for (k = sn; k <= en; k++)
{
n1 = k; // Store the current number in n1
s1 = 0; // Initialize sum of factorials as 0
// Loop to calculate the sum of factorials of digits for each number
for (j = k; j > 0; j = j / 10)
{
fact = 1; // Initialize factorial as 1 for each digit
// Calculate factorial for each digit
for (i = 1; i <= j % 10; i++)
{
fact = fact * i; // Multiply each digit's factorial
}
s1 = s1 + fact; // Accumulate the sum of factorials for each digit
}
// Check if the sum of factorials of digits is equal to the original number
if (s1 == n1)
cout << n1 << " "; // Print the Strong number if found within the range
}
cout << endl;
}
Sample Output:
Check whether a number is Strong Number or not: ------------------------------------------------------- Input starting range of number: 1 Input ending range of number: 500 The Strong numbers are: 1 2 145
Flowchart:
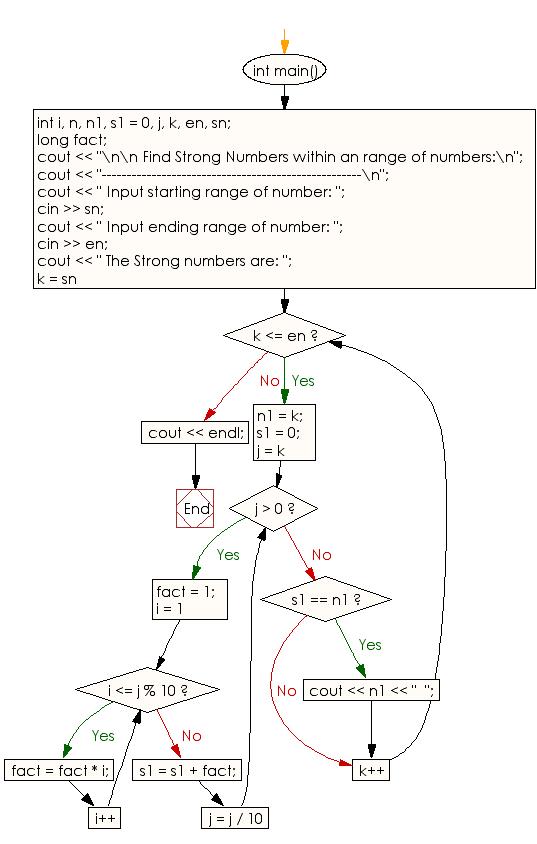
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Print the first 20 numbers of the Pell series.
Next: Check if a number is Keith or not.
What is the difficulty level of this exercise?