C++ Exercises: Check whether a number is a Strong Number or not
C++ Numbers: Exercise-45 with Solution
Write a C++ program to check whether a number is a Strong Number or not.
Sample Solution:
C++ Code:
#include <iostream>
using namespace std;
int main()
{
int i, n, n1, s1 = 0, j; // Declare variables for iteration and sum initialization
long fact; // Declare a variable to store factorial
// Prompt the user to input a number to check if it is a Strong number
cout << "\n\n Check whether a number is Strong Number or not:\n";
cout << "----------------------------------------------------\n";
cout << " Input a number to check whether it is a Strong number: ";
cin >> n; // Take user input
n1 = n; // Store the original number in n1
// Loop through the digits of the number to calculate the sum of factorials
for (j = n; j > 0; j = j / 10) {
fact = 1; // Initialize factorial to 1 for each digit
// Calculate factorial for each digit
for (i = 1; i <= j % 10; i++) {
fact = fact * i; // Multiply each digit's factorial
}
s1 = s1 + fact; // Accumulate the sum of factorials for each digit
}
// Check if the sum of factorials of digits is equal to the original number
if (s1 == n1) {
cout << n1 << " is a Strong number." << endl; // Print if the number is a Strong number
}
else {
cout << n1 << " is not a Strong number." << endl; // Print if the number is not a Strong number
}
}
Sample Output:
Check whether a number is Strong Number or not: ------------------------------------------------------- Input a number to check whether it is Strong number: 24 24 is not a Strong number
Flowchart:
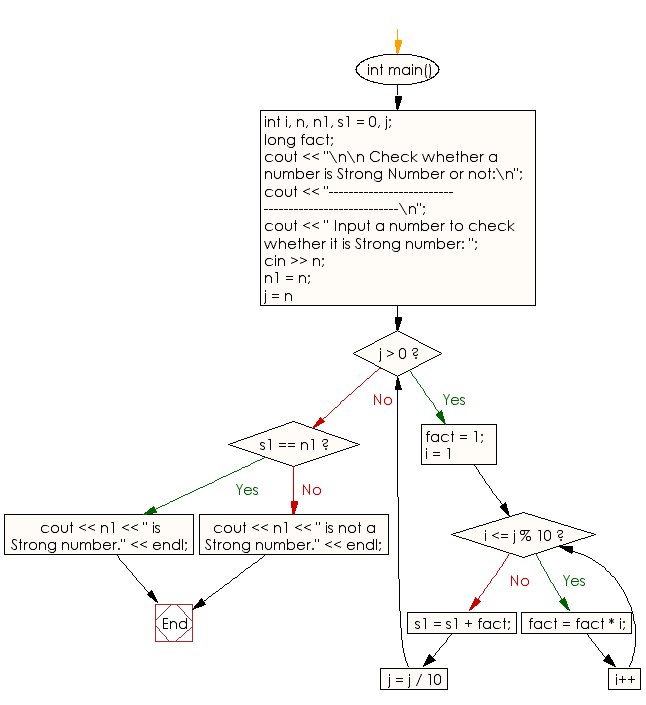
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to find the Armstrong number for a given range of number.
Next: C++ Sorting and Searching Exercises Home
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics