C++ Exercises: Find the Armstrong number for a given range of number
C++ Numbers: Exercise-44 with Solution
Write a C++ program to find the Armstrong number for a given range of numbers.
/*When the sum of the cube of the individual digits of a number
is equal to that number, the number is called Armstrong number. For example 153.
Sum of its divisor is 13 + 53;+ 33; = 1+125+27 = 153*/
Sample Solution:
C++ Code:
#include <iostream>
#include <math.h>
using namespace std;
int main()
{
// Initialize variables
int num, r, sum, t, mm;
int sno, eno;
// Display instructions to the user
cout << "\n\n Find the Armstrong number for a given range of number:\n";
cout << "-----------------------------------------------------------\n";
// Input starting and ending numbers for the range
cout << " Input starting number of range: ";
cin >> sno;
cout << " Input ending number of range: ";
cin >> eno;
// Display a message for Armstrong numbers found in the range
cout << " Armstrong numbers in given range are: " << endl;
// Loop through the range of numbers to find Armstrong numbers
for (num = sno; num <= eno; num++)
{
t = num; // Store the current number in a temporary variable
sum = 0; // Initialize the sum variable to zero
// Calculate the sum of cubes of digits in the number
while (t != 0)
{
r = t % 10; // Extract the last digit
mm = pow(r, 3); // Calculate the cube of the digit
sum = sum + mm; // Add the cube to the sum
t = t / 10; // Remove the last digit
}
// Check if the sum of cubes of digits equals the original number
if (sum == num)
cout << num << " "; // Print the Armstrong number found
}
cout << endl;
}
Sample Output:
Find the Armstrong number for a given range of number: ----------------------------------------------------------- Input starting number of range: 25 Input ending number of range: 200 Armstrong numbers in given range are: 153
Flowchart:
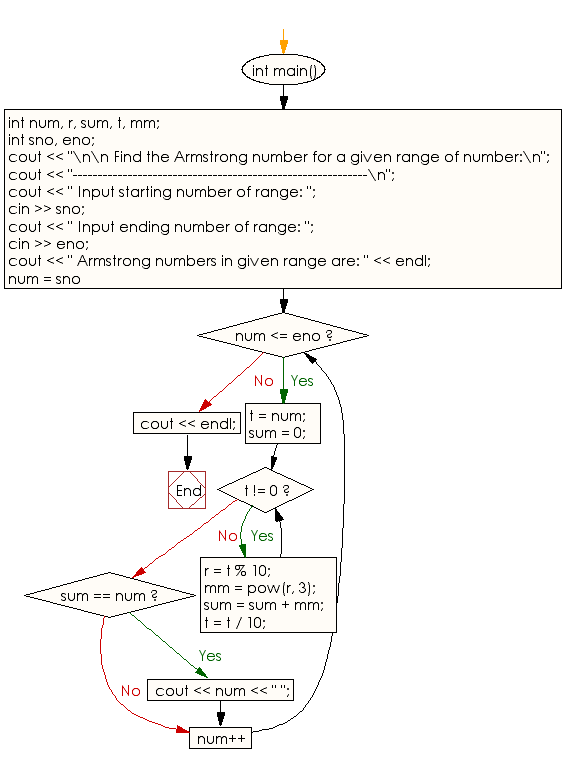
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to check whether a given number is an Armstrong number or not.
Next: Write a program in C++ to check whether a number is a Strong Number or not.
What is the difficulty level of this exercise?
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/cpp-exercises/numbers/cpp-numbers-exercise-44.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics