C++ Exercises: Check whether a given number is an Armstrong number or not
43. Armstrong Number Check
Write a C++ program to check whether a given number is an Armstrong number or not.
/*When the sum of the cube of the individual digits of a number
is equal to that number, the number is called Armstrong number. For example 153.
Sum of its divisor is 13 + 53;+ 33; = 1+125+27 = 153*/
Sample Solution:
C++ Code:
#include <iostream>
using namespace std;
int main()
{
int num, r, sum = 0, temp;
// Prompt user for input
cout << "\n\n Check whether a given number is an Armstrong number or not :\n";
cout << "-----------------------------------------------------------------\n";
cout << " Input a number: ";
cin >> num; // Input the number to check
// Loop to calculate the sum of cubes of digits
for (temp = num; num != 0; num = num / 10)
{
r = num % 10; // Extract the digit
sum = sum + (r * r * r); // Cube each digit and add to sum
}
// Check if the sum of cubes of digits equals the original number
if (sum == temp)
cout << temp << " is an Armstrong number." << endl; // Print if it's an Armstrong number
else
cout << temp << " is not an Armstrong number." << endl; // Print if it's not an Armstrong number
}
Sample Output:
Check whether a given number is an Armstrong number or not: ------------------------------------------------------- Input a number: 153 153 is an Armstrong number
Flowchart:
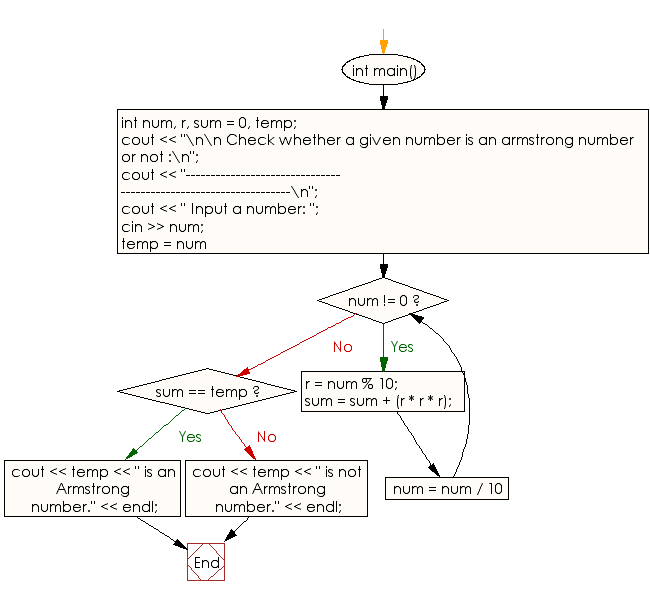
For more Practice: Solve these Related Problems:
- Write a C++ program to verify if a number is an Armstrong number by summing the cubes of its digits.
- Write a C++ program to check Armstrong numbers using a loop to extract digits and power them accordingly.
- Write a C++ program to determine Armstrong numbers by converting the number to a string and processing each digit.
- Write a C++ program to test for Armstrong numbers with error handling for multi-digit inputs using STL functions.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to create the first twenty Hamming numbers.
Next: Write a program in C++ to find the Armstrong number for a given range of number.
What is the difficulty level of this exercise?