C++ Exercises: Create the first twenty Hamming numbers
42. Generate First Twenty Hamming Numbers
Write a C++ program to create the first twenty Hamming numbers.
Sample Solution:
C++ Code:
#include <iostream>
#include <cmath>
using namespace std;
// Function to check if a number is a Hamming number
bool chkHhamming(int n) {
if (n == 1) return true; // Hamming number condition for 1
// Checking divisibility by 2, 3, or 5, the prime factors of Hamming numbers
if (n % 2 == 0 or n % 3 == 0 or n % 5 == 0) {
for (int i = 2; i * i < n; ++i) {
// Check for divisibility by prime factors
if (n % i == 0) {
if (i % 2 != 0 and i % 3 != 0 and i % 5 != 0) return false;
}
// Check for divisibility by the other factor
if (n % (n / i) == 0) {
if ((n / i) % 2 != 0 and (n / i) % 3 != 0 and (n / i) % 5 != 0) return false;
}
}
return true; // If no issues, it's a Hamming number
}
return false; // If not divisible by 2, 3, or 5, it's not a Hamming number
}
int main() {
int n, j;
cout << "\n\n Find first twenty Hamming numbers: \n";
cout << " ---------------------------------------\n";
cout << " Input the upper limit of Hamming numbers: ";
cin >> n; // Input the upper limit of Hamming numbers
cout << " The Hamming numbers are: " << endl;
// Loop to find and print the first 'n' Hamming numbers
while (j <= n) {
int count = 0;
int i = 1;
bool first = true;
// Loop to find 'n' Hamming numbers
while (count < n) {
if (chkHhamming(i)) { // If 'i' is a Hamming number
if (not first) cout << ","; // Display ',' for separation
cout << i; // Display the Hamming number
++count; // Increment count of Hamming numbers found
first = false;
}
++i; // Move to the next number
}
j++; // Increment j to control the while loop
}
cout << endl; // End of the program
}
Sample Output:
Find first twenty Hamming numbers: --------------------------------------- Input the upper limit of Hamming numbers: 20 The Hamming numbers are: 1,2,3,4,5,6,8,9,10,12,15,16,18,20,24,25,27,30,32,361,2,3,4,5,6,8,9,10,1 2,15,16,18,20,24,25,27,30,32,361,2,3,4,5,6,8,9,10,12,15,16,18,20,24,25, 27,30,32,361,2,3,4,5,6,8,9,10,12,15,16,18,20,24,25,27,30,32,361,2,3,4,5 ,6,8,9,10,12,15,16,18,20,24,25,27,30,32,361,2,3,4,5,6,8,9,10,12,15,16,1 8,20,24,25,27,30,32,361,2,3,4,5,6,8,9,10,12,15,16,18,20,24,25,27,30,32, 361,2,3,4,5,6,8,9,10,12,15,16,18,20,24,25,27,30,32,361,2,3,4,5,6,8,9,10 ,12,15,16,18,20,24,25,27,30,32,361,2,3,4,5,6,8,9,10,12,15,16,18,20,24,2 5,27,30,32,361,2,3,4,5,6,8,9,10,12,15,16,18,20,24,25,27,30,32,361,2,3,4 ,5,6,8,9,10,12,15,16,18,20,24,25,27,30,32,361,2,3,4,5,6,8,9,10,12,15,16 ,18,20,24,25,27,30,32,361,2,3,4,5,6,8,9,10,12,15,16,18,20,24,25,27,30,3 2,361,2,3,4,5,6,8,9,10,12,15,16,18,20,24,25,27,30,32,361,2,3,4,5,6,8,9, 10,12,15,16,18,20,24,25,27,30,32,361,2,3,4,5,6,8,9,10,12,15,16,18,20,24 ,25,27,30,32,361,2,3,4,5,6,8,9,10,12,15,16,18,20,24,25,27,30,32,361,2,3 ,4,5,6,8,9,10,12,15,16,18,20,24,25,27,30,32,361,2,3,4,5,6,8,9,10,12,15, 16,18,20,24,25,27,30,32,361,2,3,4,5,6,8,9,10,12,15,16,18,20,24,25,27,30 ,32,36
Flowchart:
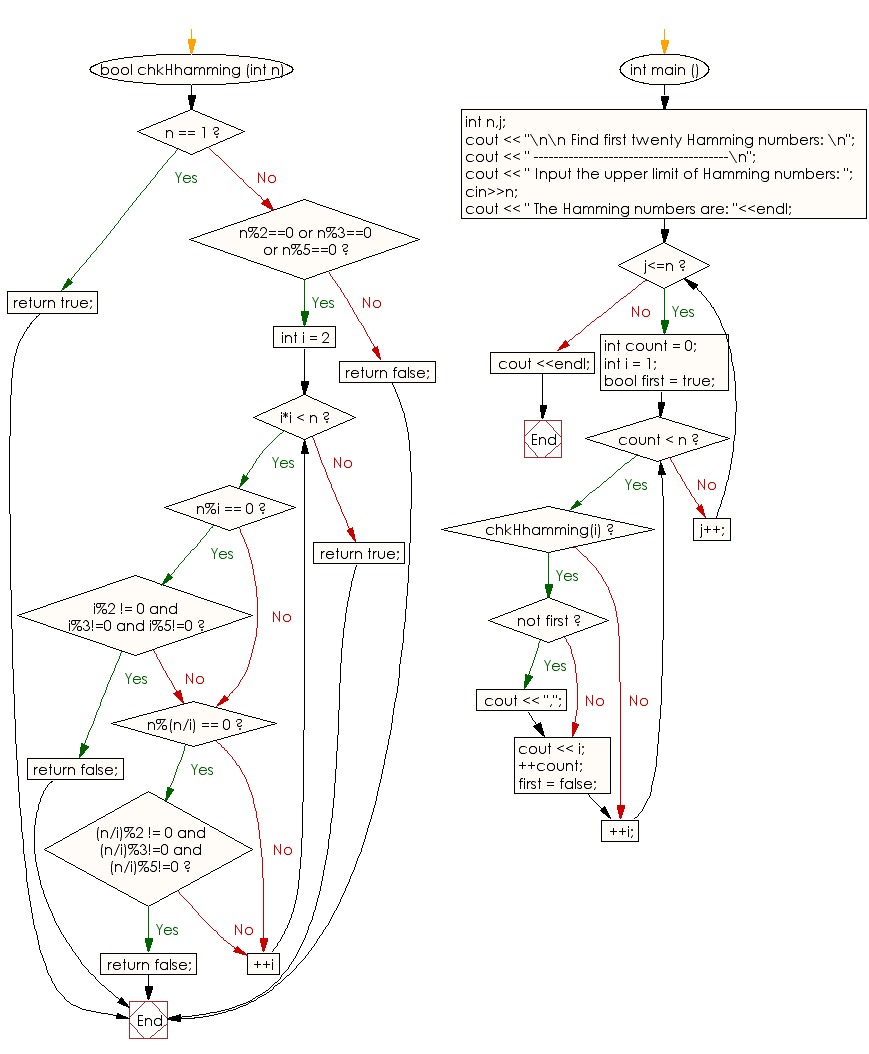
For more Practice: Solve these Related Problems:
- Write a C++ program to generate the first twenty Hamming numbers using an iterative merging algorithm.
- Write a C++ program to compute Hamming numbers with three separate queues for multiples of 2, 3, and 5.
- Write a C++ program to display Hamming numbers by dynamically generating candidates and eliminating duplicates.
- Write a C++ program to list the first twenty Hamming numbers with formatted output using STL containers.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to check if a number is Keith or not.
Next: Write a C++ program to check whether a given number is an Armstrong number or not.
What is the difficulty level of this exercise?